Vue is a popular JavaScript framework that powerfully binds data and UI and provides many useful functions. In a Vue application, you may need to write some common methods that will be used throughout the application. In order to improve the reusability and maintainability of the code, it is best to encapsulate these methods into Vue plug-ins and use them where needed. This article will introduce how to encapsulate Vue methods and use them in your application.
Encapsulating the Vue plug-in
The Vue plug-in is a JavaScript object with an install method. This install method receives the Vue constructor as its first parameter and can perform various initializations, such as adding instance methods, global components, or directly modifying the prototype. Let's look at an example of writing a Vue plugin.
const MyPlugin = { install(Vue, options) { // 添加Vue实例方法 Vue.prototype.$greet = function(name) { console.log(`Hello, ${name}!`); } // 添加全局组件 Vue.component('my-component', { // 组件选项 }) // 修改Vue原型 Vue.prototype.$axios = axios; } }; export default MyPlugin;
In this example, we define an object named MyPlugin and implement an install method. This method receives the Vue constructor along with options as its parameters. In this install method, we can add instance methods, global components, modify the Vue prototype, or perform other initialization operations. In this example, we added an instance method named $greet to the Vue instance and added a global component named my-component. Finally, we will also add the axios library to the Vue prototype for use throughout the application.
Using Vue Plugins in Applications
After you finish writing the Vue plugin, it is also easy to use it in your Vue application. We can install plugins by calling the Vue.use method, passing options (if any). For example:
import Vue from 'vue'; import MyPlugin from './my-plugin'; Vue.use(MyPlugin, { someOption: true });
In this example, we imported Vue and MyPlugin and installed the plugin using the Vue.use method. We also pass an options object that will be passed to MyPlugin's install method as the second argument.
Now that our Vue plugin is ready to use, you can use the instance methods added by the plugin, global components, or modify the Vue prototype in any Vue instance in your application. Example using the installed $greet method:
<template> <div> <button @click="$greet('World')">Greet</button> </div> </template> <script> export default { name: 'MyComponent', methods: { // 此组件中的$greet方法来自于MyPlugin } } </script>
In this example, we add a click event on the button and pass the string "World" into it. When the button is clicked, Vue calls the $greet method of the component and outputs "Hello, World!" on the console.
Best practices for Vue method encapsulation
When encapsulating Vue methods, there are some best practices to pay attention to. Here are some of them:
Concentrate methods in a plugin
In order to improve the maintainability and reusability of the code, it is best to encapsulate all Vue methods into a plugin. This helps to uniformly manage and organize all methods. If you have multiple Vue plugins, try splitting them into functional modules, each with its own set of methods. This helps improve the structure and readability of your code.
Add option parameters
It is a good practice to add an option parameter in the install method. You can pass any application-specific configuration options or parameters to the options parameter. This makes the plugin more flexible and allows it to be used in multiple projects.
const MyPlugin = { install(Vue, options) { // 检查选项是否存在 const someOption = options ? options.someOption : false; // ... } };
Use global methods
Adding global methods in the Vue plug-in can greatly improve the usability and reusability of the framework. For example, if you are writing a time formatting function and want to use it throughout your application, it is best to encapsulate it into your Vue plugin. This makes your methods transparently available in your application without having to define them repeatedly in every component.
Avoid modifying Vue prototypes directly
Modifying Vue prototypes can cause your plug-in to cause conflicts in other code. It's best to use Vue.mixin to add mixin properties and methods to your components. This ensures that your plugin does not overwrite changes made by other plugins or libraries when changing the Vue prototype.
Summary
Encapsulating Vue methods is a good way to improve the reusability and maintainability of your code. By encapsulating methods into a Vue plugin, you can make them available throughout your application and reuse your code. When writing Vue plugins, remember to follow best practices to make your code clear, readable, and easy to maintain.
The above is the detailed content of vue method encapsulation and use. For more information, please follow other related articles on the PHP Chinese website!

No,youshouldn'tusemultipleIDsinthesameDOM.1)IDsmustbeuniqueperHTMLspecification,andusingduplicatescancauseinconsistentbrowserbehavior.2)Useclassesforstylingmultipleelements,attributeselectorsfortargetingbyattributes,anddescendantselectorsforstructure

HTML5aimstoenhancewebcapabilities,makingitmoredynamic,interactive,andaccessible.1)Itsupportsmultimediaelementslikeand,eliminatingtheneedforplugins.2)Semanticelementsimproveaccessibilityandcodereadability.3)Featureslikeenablepowerful,responsivewebappl

HTML5aimstoenhancewebdevelopmentanduserexperiencethroughsemanticstructure,multimediaintegration,andperformanceimprovements.1)Semanticelementslike,,,andimprovereadabilityandaccessibility.2)andtagsallowseamlessmultimediaembeddingwithoutplugins.3)Featur

HTML5isnotinherentlyinsecure,butitsfeaturescanleadtosecurityrisksifmisusedorimproperlyimplemented.1)Usethesandboxattributeiniframestocontrolembeddedcontentandpreventvulnerabilitieslikeclickjacking.2)AvoidstoringsensitivedatainWebStorageduetoitsaccess

HTML5aimedtoenhancewebdevelopmentbyintroducingsemanticelements,nativemultimediasupport,improvedformelements,andofflinecapabilities,contrastingwiththelimitationsofHTML4andXHTML.1)Itintroducedsemantictagslike,,,improvingstructureandSEO.2)Nativeaudioand

Using ID selectors is not inherently bad in CSS, but should be used with caution. 1) ID selector is suitable for unique elements or JavaScript hooks. 2) For general styles, class selectors should be used as they are more flexible and maintainable. By balancing the use of ID and class, a more robust and efficient CSS architecture can be implemented.

HTML5'sgoalsin2024focusonrefinementandoptimization,notnewfeatures.1)Enhanceperformanceandefficiencythroughoptimizedrendering.2)Improveaccessibilitywithrefinedattributesandelements.3)Addresssecurityconcerns,particularlyXSS,withwiderCSPadoption.4)Ensur

HTML5aimedtoimprovewebdevelopmentinfourkeyareas:1)Multimediasupport,2)Semanticstructure,3)Formcapabilities,and4)Offlineandstorageoptions.1)HTML5introducedandelements,simplifyingmediaembeddingandenhancinguserexperience.2)Newsemanticelementslikeandimpr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
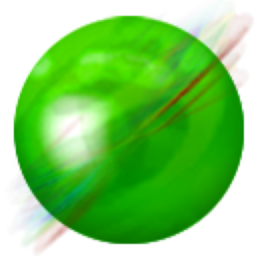
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
