As the Go language becomes increasingly popular in web development, more and more developers are beginning to consider applying it to the Tomcat application server. However, since Tomcat itself is a Java application server and cannot directly support the deployment and operation of the Go language, we need to find some special methods to achieve this goal.
This article will introduce some methods of deploying Go language applications in Tomcat, aiming to provide reference and guidance for developers who are looking for such solutions.
- Using Caddy Server
Caddy Server is an open source web server that supports multiple languages and programming languages. Unlike other web servers, one of the design goals of Caddy Server is to support the Go language, so it can be easily deployed using Caddy Server in Tomcat.
First, we need to download and install Caddy Server. After the installation is complete, rename the file to app.jar and place it in Tomcat's webapps directory.
Next, we need to write a Caddyfile to define our application. For example, if our application listens on port 80 and forwards requests via FastCGI to a Go language application named myapp, we can write the following to the Caddyfile:
myapp { proxy / http://localhost:9000 { transparent } }
Finally, we need Start Tomcat and let it load the Caddy Server application. This can be done with the following command:
$ catalina.sh run
Now we can access the myapp application on port 80 and forward the request to the Go language application via FastCGI.
- Use the FastCGI library officially provided by Go
The Go language provides a set of standard FastCGI libraries. Developers can use these libraries to deploy their applications to FastCGI. On the server, Go language applications can be easily deployed using FastCGI in Tomcat.
First, we need to use the FastCGI library in the Go language application to start the FastCGI server. The following is a simple example:
package main import ( "fmt" "net" "net/http" "net/http/fcgi" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { listener, err := net.Listen("tcp", "localhost:9000") if err != nil { panic(err) } err = fcgi.Serve(listener, http.HandlerFunc(handler)) if err != nil { panic(err) } }
At this point, we already have a basic Go language FastCGI server. Next, we need to configure Apache's mod_fastcgi module in Tomcat so that it can pass requests to the Go language application.
First, we need to install the mod_fastcgi module. If you are using a system such as Ubuntu or Debian, you can use the following command to install it:
$ sudo apt-get install libapache2-mod-fastcgi
After the installation is complete, we need to add some content to Apache’s configuration file. The following is a simple example:
<IfModule mod_fastcgi.c> <Directory /var/www/html/goapp/> Options +ExecCGI SetHandler fastcgi-script </Directory> FastCGIExternalServer /var/www/html/goapp/goapp.fcgi -host 127.0.0.1:9000 <FilesMatch .php$> SetHandler application/x-httpd-fastphp </FilesMatch> </IfModule>
At this point, we have successfully configured the mod_fastcgi module. We can now deploy our Go language application into Tomcat and let Apache forward requests to it.
- Use the net/http library officially provided by Go
In addition to the FastCGI library, the Go language also provides another library that contains a standard HTTP server implementation. We can use this library to start a web server and forward requests to our Go application.
First, we need to write a simple web server in a Go language application. Here is an example:
package main import ( "fmt" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") }) http.ListenAndServe(":9000", nil) }
Next, we need to configure Apache’s mod_proxy module in Tomcat so that it can forward requests to our Go language application.
First, we need to install the mod_proxy module. If you are using a system such as Ubuntu or Debian, you can use the following command to install it:
$ sudo apt-get install libapache2-mod-proxy-html
After the installation is complete, we need to add some content to Apache’s configuration file. The following is a simple example:
<VirtualHost *:*> ProxyPreserveHost On ProxyPass /goapp http://localhost:9000 ProxyPassReverse /goapp http://localhost:9000 </VirtualHost>
At this point, we have successfully configured the mod_proxy module. Now we can deploy our Go language application into Tomcat and forward requests to it.
Summary
As can be seen from the above introduction, there are many methods for Tomcat to deploy Go language applications, each method has its own advantages, disadvantages and applicable scenarios. Choose the method that works best for you and give it a try, and I'm sure you'll be successful in achieving this goal.
The above is the detailed content of tomcat deployment golang. For more information, please follow other related articles on the PHP Chinese website!
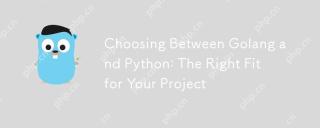
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
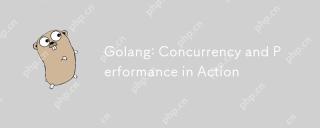
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
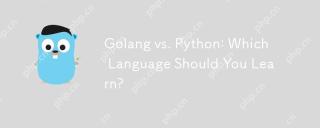
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
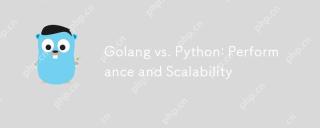
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
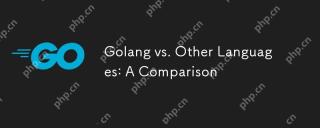
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
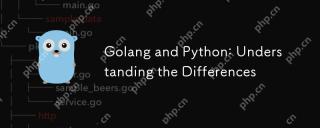
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
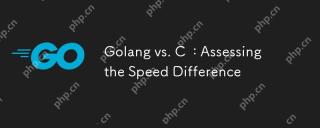
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
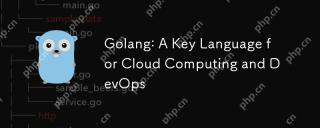
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
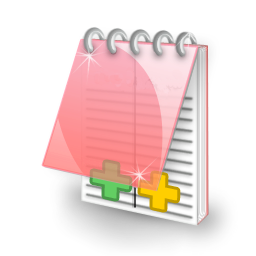
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool