How to log in to Golang
Golang is an open source programming language that was launched by Google in 2009. Golang is widely used in back-end development, cloud computing and other fields because of its advantages such as efficiency, reliability, and easy maintenance. In Golang development, due to the need to implement user login and other functions, how to implement user login in Golang has become an important issue. This article will introduce how to implement user login in Golang.
1. The principle of user login in Golang
To implement the user login function, user information, such as user name, password, etc., needs to be saved on the server side. When the user enters the user name and password, the server needs to verify the user's input. If the match is successful, the server will return authentication success information to the user and save the user's login status on the server side. The login status can be saved in the form of cookies or sessions, so that the server can identify the user the next time he visits and return the corresponding data.
2. Steps to implement user login in Golang
To implement user login in Golang, you need to complete the following steps:
- Create a database table
Create a user table in the database to save the user's user name, password and other information, as shown below:
CREATE TABLE users (
ID int NOT NULL AUTO_INCREMENT,
Name varchar( 20) NOT NULL,
Password varchar(50) NOT NULL,
PRIMARY KEY (ID)
);
- Write login page
In the front-end page, input boxes for username and password need to be provided. After the user enters his account and password, click the login button, and a request will be sent to the server. The request needs to include the account and password information entered by the user.
- Writing Golang interface
After receiving the request from the front-end page, the server needs to write the corresponding interface to receive and process the request. The processing steps are as follows:
(1) Obtain the user name and password information carried in the request.
(2) Connect to the database and verify the user name and password entered by the user. If the username and password match successfully, successful authentication information is returned and the found user information is saved on the server.
(3) Return login status information to the front end.
- Save login status
In Golang, user login status can be saved through the session or cookie mechanism. The session is some information saved on the server side, and the cookie is some information saved on the client side. After the user successfully logs in, the server can save the user information in the session and save the session identification information in the cookie so that the server can identify the user the next time he visits.
- Security issues of user information
During the user login process, the security of user information needs to be ensured. The user's password needs to be encrypted. In Golang, the bcrypt algorithm can be used to encrypt passwords. The bcrypt encryption algorithm is a secure hashing algorithm that repeatedly hashes passwords in multiple rounds, thereby enhancing password security.
3. Example of implementing user login in Golang
The following is a simple example that demonstrates how to implement user login in Golang.
- Create user table
Execute the following SQL statement in MySQL:
CREATE TABLE users (
ID int NOT NULL AUTO_INCREMENT,
Name varchar(20) NOT NULL,
Password varchar(100) NOT NULL,
PRIMARY KEY (ID)
);
- Write login page
In the HTML file, you can write the following code:
<meta charset="UTF-8" /> <title>Login Page</title>
head>
<h1 id="Login-Page">Login Page</h1> <form action="/login" method="POST"> <label for="username">Username:</label> <input type="text" id="username" name="username" /><br /> <label for="password">Password:</label> <input type="password" id="password" name="password" /><br /> <input type="submit" name="submit" value="Login" /> </form>
- Writing Golang code
The Golang code is as follows:
package main
import (
"crypto/rand" "database/sql" "fmt" "html/template" "log" "net/http" "strings" "time" "golang.org/x/crypto/bcrypt" _ "github.com/go-sql-driver/mysql"
)
type User struct {
ID int `json:"id"` Name string `json:"name"` Password string `json:"password"`
}
var db *sql.DB
func dbSetup() {
var err error db, err = sql.Open("mysql", "username:password@tcp(localhost:3306)/dbname") if err != nil { log.Fatal(err) }
}
func dbClose() {
db.Close()
}
func UserExists(username string) bool {
var count int stmt := "SELECT COUNT(*) FROM users WHERE Name = ?" row := db.QueryRow(stmt, username) err := row.Scan(&count) if err != nil { log.Fatal(err) } return count > 0
}
func AddUser(user User) {
stmt := "INSERT INTO users(Name, Password) VALUES(?, ?)" _, err := db.Exec(stmt, user.Name, user.Password) if err != nil { log.Fatal(err) }
}
func GetUserByUsername( username string) (User, error) {
var user User stmt := "SELECT ID, Name, Password FROM users WHERE Name = ?" row := db.QueryRow(stmt, username) err := row.Scan(&user.ID, &user.Name, &user.Password) switch { case err == sql.ErrNoRows: return User{}, fmt.Errorf("No user with username %s", username) case err != nil: return User{}, err } return user, nil
}
func HashPassword(password string) (string, error) {
bytes, err := bcrypt.GenerateFromPassword([]byte(password), 14) return string(bytes), err
}
func CheckPasswordHash(password, hash string) bool {
err := bcrypt.CompareHashAndPassword([]byte(hash), []byte(password)) return err == nil
}
func LoginHandler(w http.ResponseWriter, r *http.Request) {
if r.Method == "GET" { t, _ := template.ParseFiles("login.html") t.Execute(w, nil) } else { r.ParseForm() username := strings.TrimSpace(r.Form.Get("username")) password := strings.TrimSpace(r.Form.Get("password")) if !UserExists(username) { http.Error(w, "User not found.", http.StatusUnauthorized) return } user, err := GetUserByUsername(username) if err != nil { http.Error(w, err.Error(), http.StatusInternalServerError) return } if !CheckPasswordHash(password, user.Password) { http.Error(w, "Invalid credentials.", http.StatusUnauthorized) return } sessionID := createSession() cookie := &http.Cookie{ Name: "sessionid", Value: sessionID, Path: "/", Expires: time.Now().Add(time.Hour * 24 * 7), HttpOnly: true, } http.SetCookie(w, cookie) fmt.Fprintf(w, "Welcome, %s!", user.Name) }
}
func createSession() string {
b := make([]byte, 16) _, err := rand.Read(b) if err != nil { log.Fatal(err) } return fmt.Sprintf("%x", b)
}
func main() {
dbSetup() defer dbClose() http.HandleFunc("/login", LoginHandler) log.Fatal(http.ListenAndServe(":8080", nil))
}
The above code implements the user login function. Its logic mainly includes steps such as determining whether the user exists, verifying the password, generating session ID, and creating cookies. For specific implementation, please refer to the comments in the code.
4. Summary
To implement the user login function in Golang, the main steps that need to be completed include creating a user table, writing a login page, writing a Golang interface, saving login status, and ensuring the security of user information. question. During the implementation process, attention needs to be paid to the security of user information. This article uses a simple example to introduce how to implement the user login function in Golang. On this basis, readers can expand to implement more complex functions.
The above is the detailed content of How to log in golang. For more information, please follow other related articles on the PHP Chinese website!
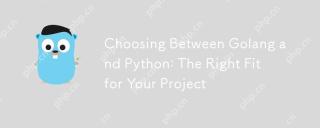
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
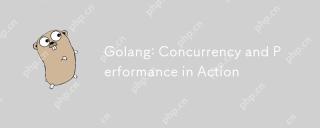
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
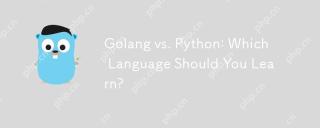
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
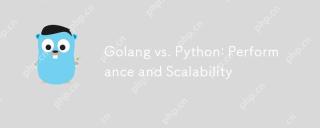
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
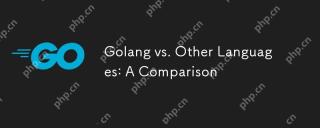
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
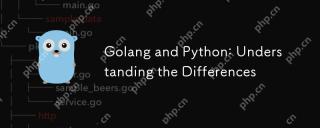
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
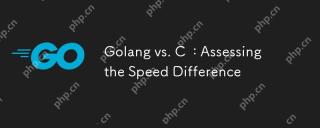
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
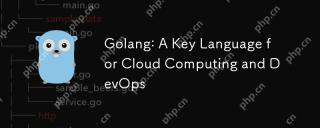
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
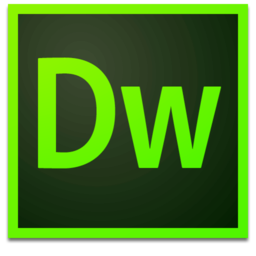
Dreamweaver Mac version
Visual web development tools
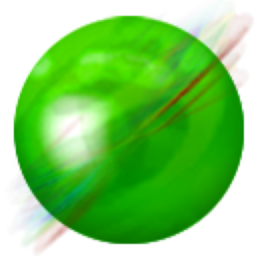
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
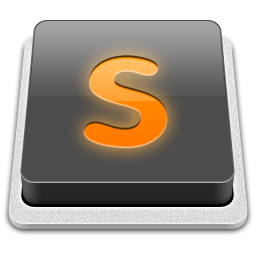
SublimeText3 Mac version
God-level code editing software (SublimeText3)