In PHP, an array is a data structure that stores multiple values. Arrays make it easy to organize, store, and access data. This article will introduce how to create arrays in PHP.
1. Introduction
An array is a container used to store multiple values. You can add square brackets [] after the variable name to store multiple values in the variable. Each value in the array is called an element. The elements in the array can be any type of data, including numbers, strings, objects, etc. Elements in an array can be accessed using an index, or using key-value pairs in an associative array.
2. Use square brackets [] to create an array
Arrays can be created using square brackets []. The example is as follows:
// 创建一个整数数组 $numbers = [1, 2, 3, 4, 5]; // 创建一个字符串数组 $names = ['Tom', 'Jerry', 'Bob']; // 创建一个混合类型的数组 $mix = [1, 'Tom', true, ['val1', 'val2']];
In the above code, $numbers
is an integer array, containing five numbers from 1 to 5; $names
is a string array, containing three strings: Tom, Jerry and Bob; $mix
is a mixed type array, containing an integer, a string, a Boolean value and a nested array.
3. Use array() to create an array
Arrays can also be created using the array()
function. The example is as follows:
// 创建一个整数数组 $numbers = array(1, 2, 3, 4, 5); // 创建一个字符串数组 $names = array('Tom', 'Jerry', 'Bob'); // 创建一个混合类型的数组 $mix = array(1, 'Tom', true, array('val1', 'val2'));
The above code is equivalent An example of creating an array using square brackets [].
4. Use index to access array elements
Elements in the array can be accessed using index. The example is as follows:
// 创建一个整数数组 $numbers = [1, 2, 3, 4, 5]; // 访问数组的第二个元素 echo $numbers[1]; // 输出 2 // 修改数组的第一个元素 $numbers[0] = 6; // 输出数组中的每个元素 foreach ($numbers as $num) { echo $num . ' '; } // 输出 6, 2, 3, 4, 5
In the above code, use $numbers[ 1]
to access the second element in the array, the output result is 2. Use $numbers[0] = 6
to change the first element in the array to 6. Use a foreach loop to iterate through each element in the array, and the output result is 6, 2, 3, 4, 5.
5. Use associative arrays
Associative arrays are a special type of array whose elements are not accessed using numeric indexes, but rather using string key-value pairs. An example is as follows:
// 创建一个关联数组 $person = [ 'name' => 'Tom', 'age' => 20, 'gender' => 'male' ]; // 使用键访问数组元素 echo $person['name']; // 输出 Tom // 修改数组元素 $person['age'] = 21; // 输出数组中的每个元素 foreach ($person as $key => $value) { echo $key . ': ' . $value . '<br>'; } // 输出: // name: Tom // age: 21 // gender: male
In the above code, string key-value pairs are used to create an associative array, and $person['name']
is used to access the elements in the associative array. The output result is Tom. Use $person['age'] = 21
to modify the elements in the associative array. Using a foreach loop to iterate through each element in the associative array, the output is name: Tom, age: 21, and gender: male.
6. Use the range() function to create an array
The range() function can be used to create an array within a numerical range. An example is as follows:
// 创建一个 1 到 10 的整数数组 $numbers = range(1, 10); // 输出数组中的每个元素 foreach ($numbers as $num) { echo $num . ' '; } // 输出 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
In the above code, the range() function is used to create an integer array from 1 to 10, and the foreach loop is used to traverse the array and output each element. The output result is 1 to 10.
7. Conclusion
In PHP, an array is a data structure that is convenient for organizing, storing and accessing data. You can use square brackets [] or the array() function to create an array, and You can access elements in an array using indexing or associative array methods. I hope this article is helpful for using arrays in PHP.
The above is the detailed content of How to create an array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
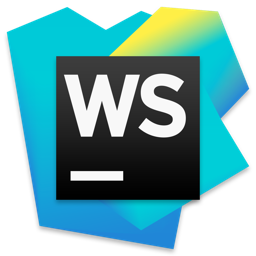
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
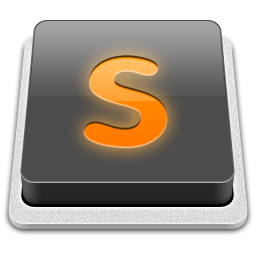
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
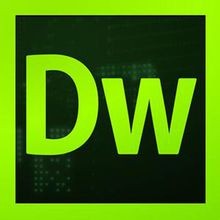
Dreamweaver CS6
Visual web development tools
