


Introduction
Conditional variables are a mechanism that uses global variables shared between threads for synchronization. It mainly includes two actions: one thread waits for the condition of the condition variable to be established and hangs (it no longer occupies the CPU at this time); Another thread makes the condition true (gives a signal that the condition is true). To prevent contention, the use of condition variables is always combined with a mutex lock.
Function prototype
1. Define condition variables
#include <pthread.h> /* 定义两个条件变量 */ pthread_cond_t cond_pro, cond_con;
2. Initialize and destroy condition variables
#include <pthread.h> int pthread_cond_init(pthread_cond_t *restrict cond, const pthread_condattr_t *restrict attr);int pthread_cond_destroy(pthread_cond_t *cond); /* 初始化条件变量 */ pthread_cond_init(&cond_pro, null); pthread_cond_init(&cond_con, null); /* 销毁条件变量 */ pthread_cond_destroy(&cond_pro); pthread_cond_destroy(&cond_pro);
3. Wait and fire conditions
#include <pthread.h> int pthread_cond_wait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex); int pthread_cond_broadcast(pthread_cond_t *cond); int pthread_cond_signal(pthread_cond_t *cond); /* 等待条件 */ /* 注意:pthread_cond_wait为阻塞函数。解开锁,再等待。等条件满足时,需要抢到锁,才可以被唤醒*/ pthread_cond_wait(&cond_pro,&mutex); /* 激发条件 */ /* 所有因为不满足条件的线程都会阻塞在条件变量cond_pro中的一个队列中 */ /* 以广播方式,通知所有被阻塞的所有线程 */ pthread_cond_broadcast(&cond_pro); /* 以signal方式,只通知排在最前面的线程 */ pthread_cond_signal(&cond_pro);
Code
/************************************************************************* > file name: my_con.c > author: krischou > mail:zhoujx0219@163.com > created time: tue 26 aug 2014 10:24:29 am cst ************************************************************************/ #include <stdio.h> #include <stdlib.h> #include <string.h> #include <pthread.h> #include <unistd.h> #define cell 10 #define flore 0 int i = 0; /* 所有线程共享的全局变量,此处假定至多递增至10,最小减到0 */ pthread_mutex_t mutex; /* 定义互斥锁 */ pthread_cond_t cond_pro, cond_con; /* 定义两个条件变量 */ /* 生产者线程 */ void* pro_handler(void *arg) { pthread_detach(pthread_self()); /* 由系统回收线程资源,而非主线程回收资源 ,此类情况主线程是个服务器,永久不会退出 */ while(1) { pthread_mutex_lock(&mutex); while(i >= cell) { pthread_cond_wait(&cond_pro,&mutex); /* continue是轮询,此处是阻塞 */ /* 把锁放开再等 ,第一个参数是结构体指针,其中有成员存放被阻塞的函数 */ /*不占cpu*/ /* 不满足条件时才会等 ,需要别人告诉它,才能唤醒它*//* 当它返回时,锁也要回来了*/ } i++; if(i == 1) { /* 由空到不空,唤醒消费者 */ pthread_cond_signal(&cond_con); /*不会立马signal被阻塞的消费者线程,因为其还要等锁抢回来*/ } printf("add i: %d \n", i); pthread_mutex_unlock(&mutex); sleep(rand() % 5 + 1); } } /* 消费者线程 */ void* con_handler(void *arg) { pthread_detach(pthread_self()); while(1) { pthread_mutex_lock(&mutex); while(i <= flore) { pthread_cond_wait(&cond_cno,&mutex); } i--; if(i == 9) /* 由满到不满,要告诉生产者,以便将其唤醒 *//*此处,直接signal也可以,我们是为了更加精确*/ { pthread_cond_signal(&cond_pro); } printf("con i: %d \n", i); pthread_mutex_unlock(&mutex); sleep(rand() % 5 + 1); } } int main(int argc, char *argv[]) // exe +num -num { srand(getpid()); int con_cnt, pro_cnt; pro_cnt = atoi(argv[1]); con_cnt = atoi(argv[2]); pthread_mutex_init(&mutex,null); pthread_cond_init(&cond_pro,null); pthread_cond_init(&cond_con,null); pthread_t *arr = (pthread_t*)calloc(con_cnt + pro_cnt , sizeof(pthread_t)); int index = 0; while(pro_cnt > 0) { pthread_create(arr + index, null, pro_handler, null); index++; pro_cnt--; } while(con_cnt > 0) { pthread_create(arr + index, null, con_handler, null); index++; con_cnt--; } while(1); pthread_mutex_destroy(&mutex); pthread_cond_destroy(&cond_pro); pthread_cond_destroy(&cond_con); return 0; }
Note
Whether it is in the producer thread or the consumer thread. The judgment condition marked in yellow must use while. Taking the producer thread as an example, when i>=cell, that is, when i is full, pthread_cond_wait(&cond_cno,&mutex); is executed at this time and the producer thread is suspended. Must wait until the consumer thread pthread_cond_signal(&cond_pro); wakes it up. But it is not enough for the consumer to signal it. The producer thread that is suspended must get the lock again before it can be activated. However, since the producer cannot immediately grab the lock when the consumer signals, the i value may change to greater than or equal to 10 at this time. Therefore you must use while. Otherwise, i>10 may result.
The above is the detailed content of How to implement Linux multi-thread programming. For more information, please follow other related articles on the PHP Chinese website!
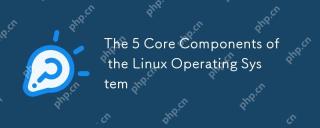
The five core components of the Linux operating system are: 1. Kernel, 2. System libraries, 3. System tools, 4. System services, 5. File system. These components work together to ensure the stable and efficient operation of the system, and together form a powerful and flexible operating system.
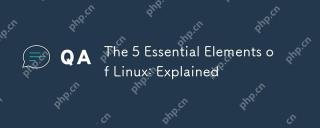
The five core elements of Linux are: 1. Kernel, 2. Command line interface, 3. File system, 4. Package management, 5. Community and open source. Together, these elements define the nature and functionality of Linux.
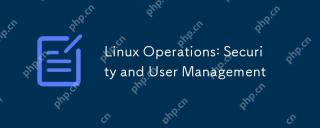
Linux user management and security can be achieved through the following steps: 1. Create users and groups, using commands such as sudouseradd-m-gdevelopers-s/bin/bashjohn. 2. Bulkly create users and set password policies, using the for loop and chpasswd commands. 3. Check and fix common errors, home directory and shell settings. 4. Implement best practices such as strong cryptographic policies, regular audits and the principle of minimum authority. 5. Optimize performance, use sudo and adjust PAM module configuration. Through these methods, users can be effectively managed and system security can be improved.
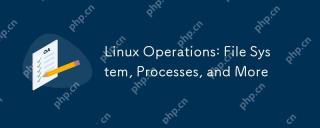
The core operations of Linux file system and process management include file system management and process control. 1) File system operations include creating, deleting, copying and moving files or directories, using commands such as mkdir, rmdir, cp and mv. 2) Process management involves starting, monitoring and killing processes, using commands such as ./my_script.sh&, top and kill.
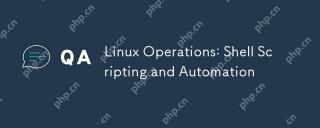
Shell scripts are powerful tools for automated execution of commands in Linux systems. 1) The shell script executes commands line by line through the interpreter to process variable substitution and conditional judgment. 2) The basic usage includes backup operations, such as using the tar command to back up the directory. 3) Advanced usage involves the use of functions and case statements to manage services. 4) Debugging skills include using set-x to enable debugging mode and set-e to exit when the command fails. 5) Performance optimization is recommended to avoid subshells, use arrays and optimization loops.
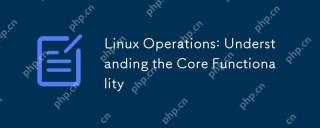
Linux is a Unix-based multi-user, multi-tasking operating system that emphasizes simplicity, modularity and openness. Its core functions include: file system: organized in a tree structure, supports multiple file systems such as ext4, XFS, Btrfs, and use df-T to view file system types. Process management: View the process through the ps command, manage the process using PID, involving priority settings and signal processing. Network configuration: Flexible setting of IP addresses and managing network services, and use sudoipaddradd to configure IP. These features are applied in real-life operations through basic commands and advanced script automation, improving efficiency and reducing errors.
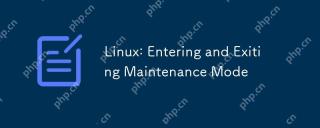
The methods to enter Linux maintenance mode include: 1. Edit the GRUB configuration file, add "single" or "1" parameters and update the GRUB configuration; 2. Edit the startup parameters in the GRUB menu, add "single" or "1". Exit maintenance mode only requires restarting the system. With these steps, you can quickly enter maintenance mode when needed and exit safely, ensuring system stability and security.
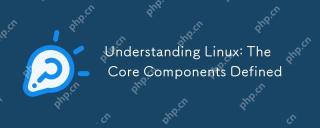
The core components of Linux include kernel, shell, file system, process management and memory management. 1) Kernel management system resources, 2) shell provides user interaction interface, 3) file system supports multiple formats, 4) Process management is implemented through system calls such as fork, and 5) memory management uses virtual memory technology.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
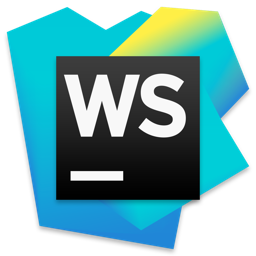
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
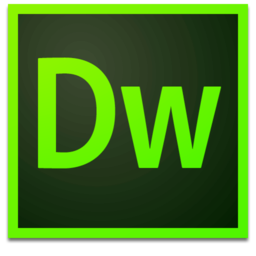
Dreamweaver Mac version
Visual web development tools
