Data storage functions play an extremely important role in PHP. There are a variety of data storage functions in PHP, including file operation functions, database operation functions, and so on. This article will focus on how to use data storage functions in PHP.
1. File operation function
-
fopen() function: Open the file and return the file pointer. The syntax is as follows:
resource fopen ( string $filename , string $mode [, bool $use_include_path = FALSE [, resource $context ]] )
Among them, $filename is a required option, which refers to the file path to be opened; $mode is a required option, which specifies the file opening mode, such as "r" means read-only opening, "w" means only Write open etc.
-
fwrite() function: writes data to an opened file. The syntax is as follows:
int fwrite ( resource $handle , string $string [, int $length ] )
Among them, $handle is a required option and refers to the file pointer; $ string is a required option and refers to the string to be written; $length is optional and refers to the maximum number of bytes to be written.
-
fclose() function: close an open file. The syntax is as follows:
bool fclose ( resource $handle )
Among them, $handle is a required option and refers to the file pointer.
Sample code:
$file = fopen("test.txt","w"); fwrite($file,"Hello World. Testing!"); fclose($file);
The above code creates a file test.txt and writes the string "Hello World. Testing!" into it.
2. Database operation function
-
mysqli_connect() function: connect to the MySQL database, the syntax is as follows:
mysqli mysqli_connect ( string $host = ini_get("mysqli.default_host") , string $username = ini_get("mysqli.default_user") , string $password = ini_get("mysqli.default_pw") , string $dbname = "" , int $port = ini_get("mysqli.default_port") , string $socket = ini_get("mysqli.default_socket") )
Among them, $host is optional, Specify the address of the MySQL server to be connected; $username is optional and specifies the user name to connect to the MySQL server; $password is optional and specifies the password to connect to the MySQL server; $dbname is optional and specifies the name of the database to be connected; $port It is optional and specifies the MySQL server port number to be connected; $socket is optional and specifies the MySQL server socket to be used.
-
mysqli_query() function: execute a MySQL query statement, the syntax is as follows:
mixed mysqli_query ( mysqli $link , string $query [, int $resultmode = MYSQLI_STORE_RESULT ] )
Among them, $link is a required option, specifying the MySQL connection identifier; $query is Required option, specifies the MySQL query statement to be executed; $resultmode is optional, specifying the method of obtaining the result set. For example, MYSQLI_STORE_RESULT indicates that the result set is retained on the client side, MYSQLI_USE_RESULT indicates that the result set is retained on the server side, etc.
-
mysqli_fetch_array() function: Get a row from the result set as an associative array, a numeric array, or both. The syntax is as follows:
mixed mysqli_fetch_array ( mysqli_result $result [, int $resulttype = MYSQLI_BOTH ] )
Among them, $result is required Option, specifying the result set; $resulttype is optional, specifying the return array type, for example, MYSQLI_BOTH is the default value, indicating that associative arrays and numeric arrays are returned.
Sample code:
$link = mysqli_connect("localhost","my_user","my_password","my_db"); $result = mysqli_query($link,"SELECT * FROM user"); while($row = mysqli_fetch_array($result)) { echo $row['username'] . " - " . $row['email']; echo "<br>"; } mysqli_close($link);
The above code connects to a MySQL database named "my_db", executes a SELECT statement to query all data in the user table, and Print the results line by line.
To sum up, there are many data storage functions in PHP, and file operation functions and database operation functions are the two most commonly used ones. Mastering their usage can enable more efficient data storage and management in actual development.
The above is the detailed content of How to use data storage functions in PHP. For more information, please follow other related articles on the PHP Chinese website!
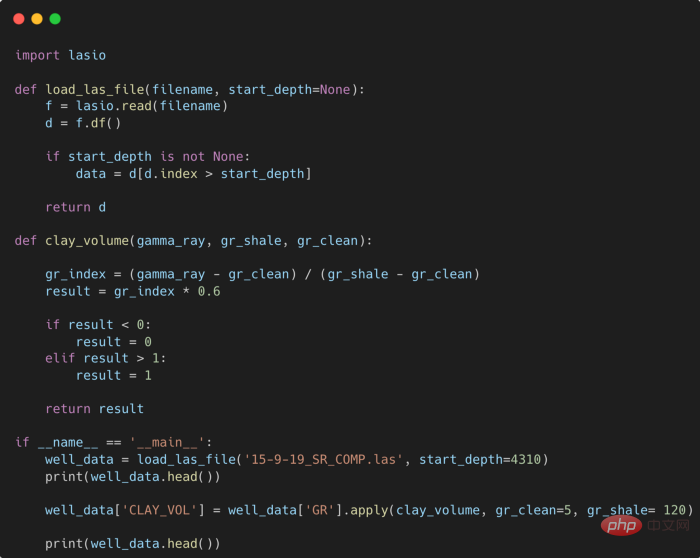
Python 中有许多方法可以帮助我们理解代码的内部工作原理,良好的编程习惯,可以使我们的工作事半功倍!例如,我们最终可能会得到看起来很像下图中的代码。虽然不是最糟糕的,但是,我们需要扩展一些事情,例如:load_las_file 函数中的 f 和 d 代表什么?为什么我们要在 clay 函数中检查结果?这些函数需要什么类型?Floats? DataFrames?在本文中,我们将着重讨论如何通过文档、提示输入和正确的变量名称来提高应用程序/脚本的可读性的五个基本技巧。1. Comments我们可
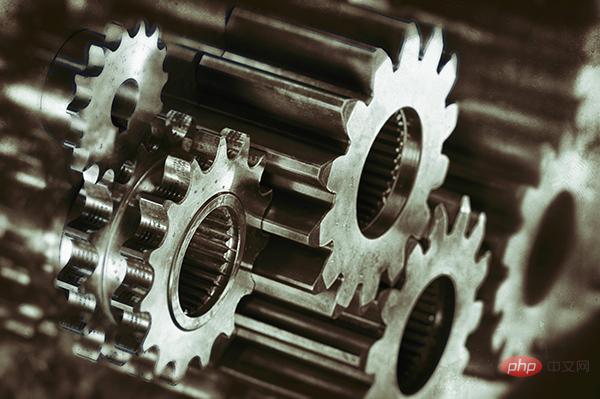
连续分级概率评分(Continuous Ranked Probability Score, CRPS)或“连续概率排位分数”是一个函数或统计量,可以将分布预测与真实值进行比较。机器学习工作流程的一个重要部分是模型评估。这个过程本身可以被认为是常识:将数据分成训练集和测试集,在训练集上训练模型,并使用评分函数评估其在测试集上的性能。评分函数(或度量)是将真实值及其预测映射到一个单一且可比较的值 [1]。例如,对于连续预测可以使用 RMSE、MAE、MAPE 或 R 平方等评分函数。如果预测不是逐点
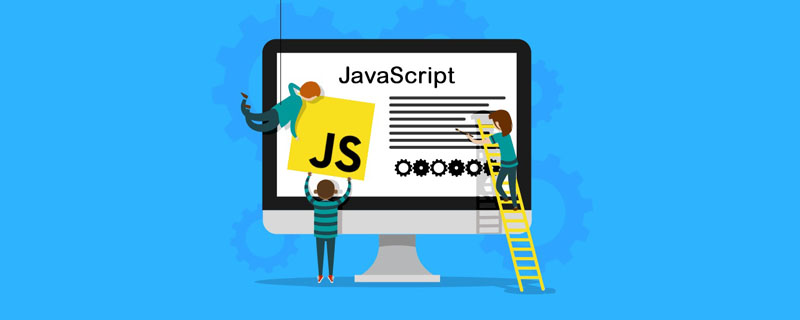
js是弱类型语言,不能像C#那样使用param关键字来声明形参是一个可变参数。那么js中,如何实现这种可变参数呢?下面本篇文章就来聊聊JavaScript函数可变参数的实现方法,希望对大家有所帮助!
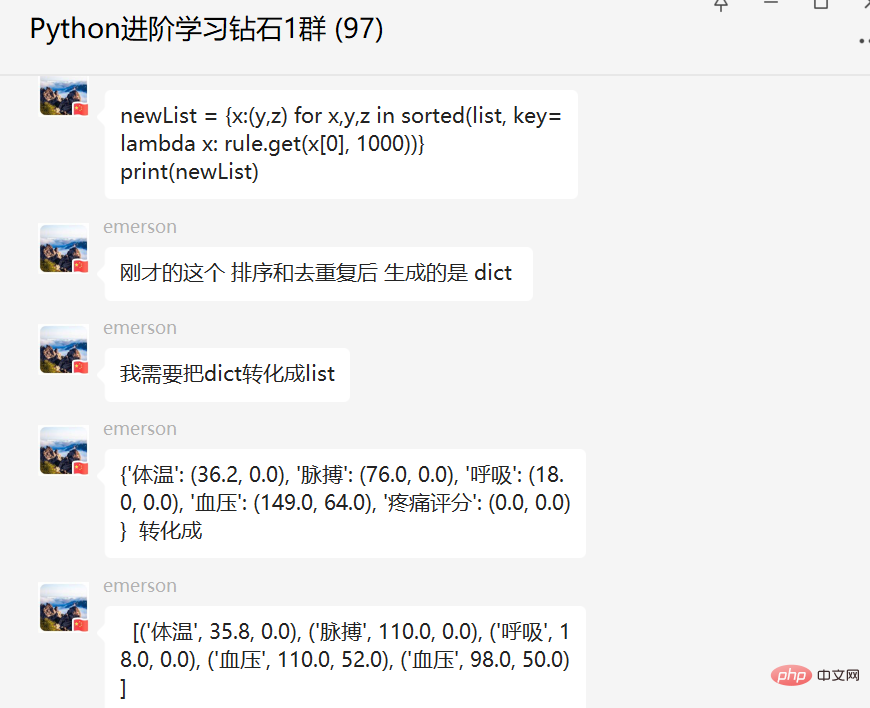
一、前言前几天在Python钻石交流群有个叫【emerson】的粉丝问了一个Python排序的问题,这里拿出来给大家分享下,一起学习下。其实这里【瑜亮老师】、【布达佩斯的永恒】等人讲了很多,只不过对于基础不太好的小伙伴们来说,还是有点难的。不过在实际应用中内置函数sorted()用的还是蛮多的,这里也单独拿出来讲一下,希望下次再有小伙伴遇到的时候,可以不慌。二、基础用法内置函数sorted()可以用来做排序,基础的用法很简单,看个例子,如下所示。lst=[3,28,18,29,2,5,88
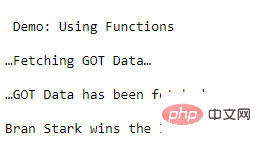
Python 中的 main 函数充当程序的执行点,在 Python 编程中定义 main 函数是启动程序执行的必要条件,不过它仅在程序直接运行时才执行,而在作为模块导入时不会执行。要了解有关 Python main 函数的更多信息,我们将从如下几点逐步学习:什么是 Python 函数Python 中 main 函数的功能是什么一个基本的 Python main() 是怎样的Python 执行模式Let’s get started什么是 Python 函数相信很多小伙伴对函数都不陌生了,函数是可

好嘞,今天我们继续剖析下Python里的类。[[441842]]先前我们定义类的时候,使用到了构造函数,在Python里的构造函数书写比较特殊,他是一个特殊的函数__init__,其实在类里,除了构造函数还有很多其他格式为__XXX__的函数,另外也有一些__xx__的属性。下面我们一一说下:构造函数Python里所有类的构造函数都是__init__,其中根据我们的需求,构造函数又分为有参构造函数和无惨构造函数。如果当前没有定义构造函数,那么系统会自动生成一个无参空的构造函数。例如:在有继承关系
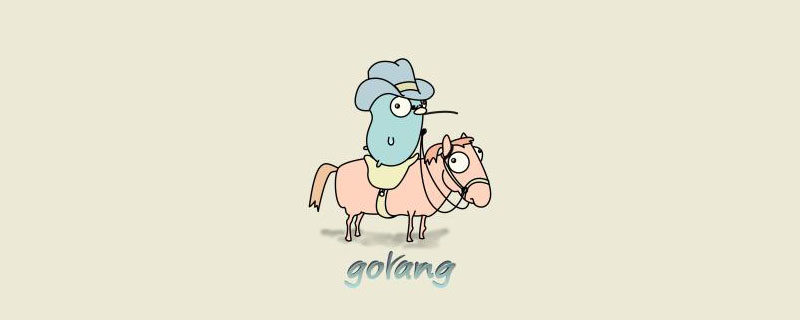
形参变量在未出现函数调用时并不占用内存,只在调用时才占用,调用结束后将释放内存。形参全称“形式参数”,是函数定义时使用的参数;但函数定义时参数是没有任实际何数据的,因而在函数被调用前没有为形参分配内存,其作用是说明自变量的类型和形态以及在过程中的作用。
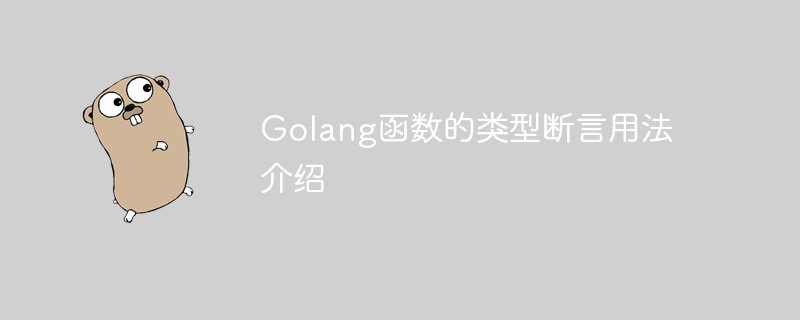
Golang的函数类型断言是一个非常重要的特性,它可以让我们在函数中精细地控制变量的类型,从而更加方便地进行数据处理和转换。本文将介绍Golang函数的类型断言用法,希望能够对大家的学习有所帮助。一、什么是Golang函数的类型断言?Golang函数的类型断言可以理解为函数参数中所声明变量的类型具有多态性,这使得一个函数在不同的参数传递下可以灵活


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
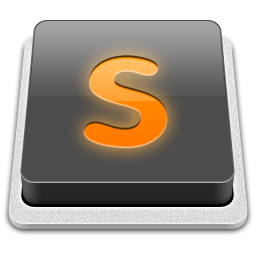
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version
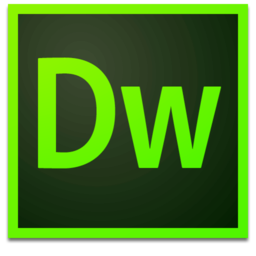
Dreamweaver Mac version
Visual web development tools
