Data preprocessing function is a method for interaction between PHP and database. Since SQL is very vulnerable to injection attacks, the use of data preprocessing functions allows us to process data more securely. In this article, we will learn how to use data preprocessing functions in PHP.
What is the data preprocessing function?
Data preprocessing is a SQL statement execution technology that uses parameter placeholders to replace variables in dynamically generated SQL statements to avoid SQL injection attacks. Data preprocessing significantly improves the performance of SQL statements because it reduces the compilation time of SQL statements and executes them faster. The PHP database extension provides data preprocessing functions to help us precompile SQL statements more easily.
How to use data preprocessing function?
The first step to use the data preprocessing function is to connect to the database. In PHP we can interact with MySQL database using PDO (PHP Data Objects) extension or mysqli extension. Next, we'll use the PDO extension as an example.
Connect to the database
We use the PDO extension to interact with the database, first we need to create a PDO object. While creating a PDO object, we need to pass the necessary parameters such as database type, hostname, database name, username and password.
For example:
$dsn = 'mysql:host=hostname;dbname=database'; $username = 'username'; $password = 'password'; try { $pdo = new PDO($dsn, $username, $password); } catch (PDOException $e) { echo 'Connection failed: ' . $e->getMessage(); }
Preparing the SQL statement
Next, we need to prepare the SQL statement to be executed. We can use the placeholder "?" to indicate that we need to use parameters. For example:
$sql = 'SELECT * FROM users WHERE username = ? AND password = ?';
Note that parameter placeholders cannot be used for table names or column names. Only changing data can use parameter placeholders.
Bind parameters
Once we have the SQL statement, we need to bind the parameters to the placeholders. PDO provides two methods of binding parameters: named parameters and placeholder parameters.
The format of named parameters is ":name", for example:
$sql = 'SELECT * FROM users WHERE username = :username AND password = :password'; $stmt = $pdo->prepare($sql); $stmt->bindParam(':username', $username); $stmt->bindParam(':password', $password);
The format of placeholder parameters is "?", for example:
$sql = 'SELECT * FROM users WHERE username = ? AND password = ?'; $stmt = $pdo->prepare($sql); $stmt->bindParam(1, $username); $stmt->bindParam(2, $password);
In the above code , we use PDO's prepare() method to prepare the SQL statement to be executed. We then bind the parameter to the placeholder using the bindParam() method. Note that the bindParam() method requires the parameter name or position and the name of the variable to be bound as parameters.
Execute SQL statement
Now that we have prepared the SQL statement that needs to be executed and bound the parameters to the placeholders, we can use the execute() method of PDO to execute the SQL statement. For example:
$stmt->execute();
Get the results
After executing the SQL statement, the next step is to get the results. We can get the results using PDO's fetch() or fetchAll() methods.
fetch() method gets the results by rows:
while($row = $stmt->fetch()) { // 处理每行的结果 }
fetchAll() method gets all the results at once:
$rows = $stmt->fetchAll(); // 处理所有结果
Note that placeholders are now used in the SQL statement , the "prepare" method must be used, and the "query" method cannot be used, otherwise there will be the risk of SQL injection.
Summary
Using data preprocessing functions in PHP can greatly improve the security of the database and avoid SQL injection attacks. By using PDO extensions, we can easily precompile SQL statements and bind parameters to placeholders, thereby avoiding the risk of manually splicing SQL statements. It should be noted that in SQL statements that currently use placeholders, the "prepare" method must be used to execute the query SQL statement, and the "query" method cannot be used.
The above is the detailed content of How to use data preprocessing functions in PHP. For more information, please follow other related articles on the PHP Chinese website!
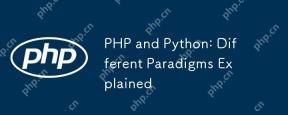
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
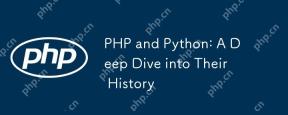
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
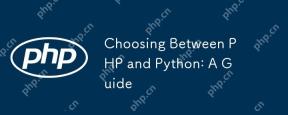
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
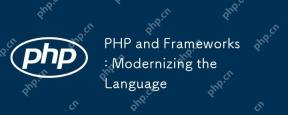
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
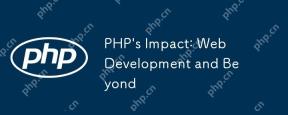
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
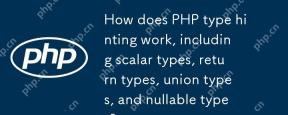
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
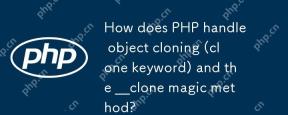
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
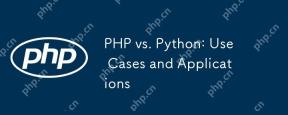
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
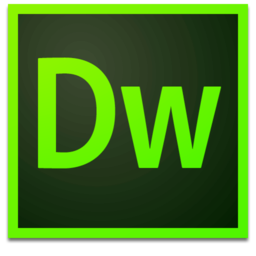
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
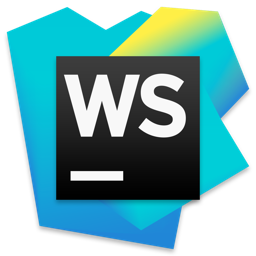
WebStorm Mac version
Useful JavaScript development tools