With the development of front-end technology, the usage of jQuery is getting higher and higher. Among them, it is very common to use ajax requests in jQuery to obtain data. However, in actual development, some problems will occur when encountering cross-domain requests. This article will introduce how to solve cross-domain request errors.
1. What is a cross-domain request
Under the same domain name, the browser's ajax request can send and receive data freely. However, under different domain names, ajax requests in the browser are restricted by security policies, and data cannot be sent and received at will. In this way, cross-domain requests occur.
2. Why cross-domain request errors occur
For security reasons, the browser adopts the same-origin policy. The same-origin policy means that client scripts from different sources cannot read each other's resources without explicit authorization. Under the same-origin policy, client scripts from different sources cannot interfere with each other, thus ensuring the security of web applications.
Specifically speaking, homology means that the protocol, domain name, and port number are completely the same.
3. How to solve cross-domain request errors
With the above background knowledge, we can solve this problem for cross-domain request errors.
- JSONP cross-domain request
JSONP (JSON with Padding) is a solution for cross-domain requests. Its principle is that when making a request, the front end dynamically creates a script tag and passes a callback function name, and the data returned by the server will be executed in the callback function.
The specific steps are as follows:
1) Front-end code
$.ajax({ url:'/api/get-data', dataType:'jsonp', jsonp:'callback', success:function (data) { console.log(data); } });
Description:
url: Requested interface address
dataType: Data type, here is jsonp
jsonp: Client request function, the function name will be used as the name of the callback function
success: Request success
2) Backend code
(1) Add the request parameter callback before the return value
{
'name':'Tom',
'age':23,
'sex' :'Male',
'callbackCode':1
}
(2) Return result
callbacks.callbackCode({"name":"Tom","age" :23,"sex":"male"});
Among them, callbackCode is the request method name set by the front end.
Another example:
(1) The request parameter callback is added before the return value
{
'code':'0',
'data' :{
'name':'Tom', 'age':23, 'sex':'男',
},
'message': 'The request was successful! ',
'callbackCode':1
}
(2) The backend splices the callback function through the callbackCode parameter.
callbacks.callbackCode({"code":"0","data":{"name":"Tom","age":23,"sex":"male"},"message" :"Request successful!"});
Through the above steps, we can use JSONP to make cross-domain requests. However, JSONP also has some limitations, such as only supporting GET requests and being unable to obtain responses to POST requests.
- Add the response header Access-Control-Allow-Origin
Another way is to set it at the backend interface and add Access-Control- in the response header. The Allow-Origin field tells the requesting browser to allow this cross-origin request.
Code example:
@RequestMapping("/api/get-data")
@RestController
public class GetDataController {
@GetMapping public String getData(HttpServletRequest request) { String callback = request.getParameter("callback"); //模拟后端返回数据 String json = "{"name":"Tom","age":23,"sex":"男"}"; //跨域请求响应头设置 String result = callback + "(" + json + ")"; return result; }
}
Among them, the method is mapped to the /api/get-data interface through the @GetMapping annotation, then the request parameter callback is obtained, the backend is simulated to return data, and finally the json data is returned in the form of a callback function through the result variable.
Through the above two methods, we can solve the problem of cross-domain request errors.
Summary:
Through the introduction of this article, we understand what cross-domain requests are, why cross-domain request errors occur, and how to solve cross-domain request errors. Among them, we mainly introduced two methods: JSONP cross-domain request and adding response header Access-Control-Allow-Origin. Of course, choosing different methods to solve problems in different scenarios can truly meet the needs.
The above is the detailed content of jquery ajax cross-domain request error reporting. For more information, please follow other related articles on the PHP Chinese website!

KeysinReactarecrucialforoptimizingperformancebyaidinginefficientlistupdates.1)Usekeystoidentifyandtracklistelements.2)Avoidusingarrayindicesaskeystopreventperformanceissues.3)Choosestableidentifierslikeitem.idtomaintaincomponentstateandimproveperform

Reactkeysareuniqueidentifiersusedwhenrenderingliststoimprovereconciliationefficiency.1)TheyhelpReacttrackchangesinlistitems,2)usingstableanduniqueidentifierslikeitemIDsisrecommended,3)avoidusingarrayindicesaskeystopreventissueswithreordering,and4)ens

UniquekeysarecrucialinReactforoptimizingrenderingandmaintainingcomponentstateintegrity.1)Useanaturaluniqueidentifierfromyourdataifavailable.2)Ifnonaturalidentifierexists,generateauniquekeyusingalibrarylikeuuid.3)Avoidusingarrayindicesaskeys,especiall

Using indexes as keys is acceptable in React, but only if the order of list items is unchanged and not dynamically added or deleted; otherwise, a stable and unique identifier should be used as the keys. 1) It is OK to use index as key in a static list (download menu option). 2) If list items can be reordered, added or deleted, using indexes will lead to state loss and unexpected behavior. 3) Always use the unique ID of the data or the generated identifier (such as UUID) as the key to ensure that React correctly updates the DOM and maintains component status.

JSXisspecialbecauseitblendsHTMLwithJavaScript,enablingcomponent-basedUIdesign.1)ItallowsembeddingJavaScriptinHTML-likesyntax,enhancingUIdesignandlogicintegration.2)JSXpromotesamodularapproachwithreusablecomponents,improvingcodemaintainabilityandflexi
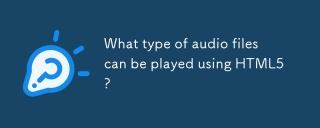
The article discusses HTML5 audio formats and cross-browser compatibility. It covers MP3, WAV, OGG, AAC, and WebM, and suggests using multiple sources and fallbacks for broader accessibility.
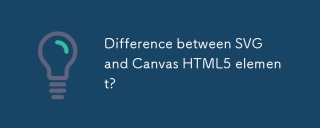
SVG and Canvas are HTML5 elements for web graphics. SVG, being vector-based, excels in scalability and interactivity, while Canvas, pixel-based, is better for performance-intensive applications like games.
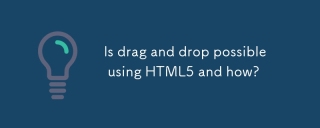
HTML5 enables drag and drop with specific events and attributes, allowing customization but facing browser compatibility issues on older versions and mobile devices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
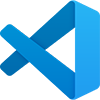
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
