JavaScript is a widely used programming language that enables interactivity and dynamic functionality on websites. One problem that many developers may face is that JavaScript parameters are missing. This article will explore this problem and provide a solution.
First, let’s understand what JavaScript parameters are. In JavaScript, we can pass parameters when calling a function to perform the corresponding operation. For example:
function greet(name) { console.log("Hello, " + name + "!"); } greet("Tom");
In the above code, we declared a function named "greet" which has a parameter named "name". When calling the function, we pass the parameter "Tom" and the function will output "Hello, Tom!".
However, sometimes when we use JavaScript, we will find that parameters are missing. This will prevent our function from working properly, causing all kinds of unexpected errors. So why does this happen?
There may be many reasons for parameter loss. Below we will introduce some of the reasons and solutions.
- No parameters are passed in when the function is called
This is the most common situation. When we do not pass in parameters when calling the function, the function will use the default value or undefined. For example:
function greet(name) { console.log("Hello, " + name + "!"); } greet(); // 输出"Hello, undefined!"
In the above code, we did not pass parameters to the "greet" function, so the value of the "name" parameter is undefined.
The solution is to pass the correct parameters when calling the function, or set a default value for the parameter:
function greet(name = "world") { console.log("Hello, " + name + "!"); } greet(); // 输出"Hello, world!"
In the above code, we set the default value "world" for the "name" parameter ", so the function will still work even if no parameters are passed.
- Parameter name error
When we pass parameters in a function call, we must ensure that the parameter name matches the name in the function definition. If the names do not match, parameter values cannot be passed to the function. For example:
function greet(name) { console.log("Hello, " + name + "!"); } greet("Tom"); // 输出"Hello, Tom!" greet("Jerry"); // 输出"Hello, Jerry!" greet("Tom", "Jerry"); // 输出"Hello, Tom!"
In the above code, when we pass two parameters, only the first parameter "name" is passed to the function.
The solution is to ensure that the parameter name is correct, or use an object to pass as a parameter:
function greet(obj) { console.log("Hello, " + obj.name + "!"); } greet({ name: "Tom" }); // 输出"Hello, Tom!" greet({ name: "Jerry" }); // 输出"Hello, Jerry!"
In the above code, we use an object to pass as a parameter, ensuring the correctness of the parameter name, And parameter values can be accessed through property names.
- Parameter loses scope
When the variable name used inside the function is the same as the variable name in the external environment, and "var" or "let" is not used inside the function When a variable is declared using the "etc" keyword, the function will use the variable from the external environment. For example:
var name = "Tom"; function greet() { console.log("Hello, " + name + "!"); } greet(); // 输出"Hello, Tom!"
In the above code, the variable "name" is used internally in the function "greet". Since the variable is not declared using keywords, the function will use the "name" variable in the external environment.
But what happens if we declare a variable with the same name inside a function?
var name = "Tom"; function greet() { var name = "Jerry"; console.log("Hello, " + name + "!"); } greet(); // 输出"Hello, Jerry!" console.log(name); // 输出"Tom"
In the above code, we declared the variable "name" with the same name inside the function. Since JavaScript uses function scope, the "name" variable inside the function will shield variables in the external environment. Therefore, what is output inside the function is the "name" variable inside the function, not the variable in the external environment.
The solution is to avoid variable name conflicts, or use function parameters instead of global variables.
Summary: When JavaScript parameters are missing, we should first check whether the parameter names in the function definition are followed, whether the parameters are passed correctly, and whether there is a variable name conflict. Understanding the causes and solutions to these problems can help us use JavaScript better.
The above is the detailed content of javascript parameter missing. For more information, please follow other related articles on the PHP Chinese website!

React’s popularity includes its performance optimization, component reuse and a rich ecosystem. 1. Performance optimization achieves efficient updates through virtual DOM and diffing mechanisms. 2. Component Reuse Reduces duplicate code by reusable components. 3. Rich ecosystem and one-way data flow enhance the development experience.

React is the tool of choice for building dynamic and interactive user interfaces. 1) Componentization and JSX make UI splitting and reusing simple. 2) State management is implemented through the useState hook to trigger UI updates. 3) The event processing mechanism responds to user interaction and improves user experience.

React is a front-end framework for building user interfaces; a back-end framework is used to build server-side applications. React provides componentized and efficient UI updates, and the backend framework provides a complete backend service solution. When choosing a technology stack, project requirements, team skills, and scalability should be considered.

The relationship between HTML and React is the core of front-end development, and they jointly build the user interface of modern web applications. 1) HTML defines the content structure and semantics, and React builds a dynamic interface through componentization. 2) React components use JSX syntax to embed HTML to achieve intelligent rendering. 3) Component life cycle manages HTML rendering and updates dynamically according to state and attributes. 4) Use components to optimize HTML structure and improve maintainability. 5) Performance optimization includes avoiding unnecessary rendering, using key attributes, and keeping the component single responsibility.

React is the preferred tool for building interactive front-end experiences. 1) React simplifies UI development through componentization and virtual DOM. 2) Components are divided into function components and class components. Function components are simpler and class components provide more life cycle methods. 3) The working principle of React relies on virtual DOM and reconciliation algorithm to improve performance. 4) State management uses useState or this.state, and life cycle methods such as componentDidMount are used for specific logic. 5) Basic usage includes creating components and managing state, and advanced usage involves custom hooks and performance optimization. 6) Common errors include improper status updates and performance issues, debugging skills include using ReactDevTools and Excellent

React is a JavaScript library for building user interfaces, with its core components and state management. 1) Simplify UI development through componentization and state management. 2) The working principle includes reconciliation and rendering, and optimization can be implemented through React.memo and useMemo. 3) The basic usage is to create and render components, and the advanced usage includes using Hooks and ContextAPI. 4) Common errors such as improper status update, you can use ReactDevTools to debug. 5) Performance optimization includes using React.memo, virtualization lists and CodeSplitting, and keeping code readable and maintainable is best practice.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

React components can be defined by functions or classes, encapsulating UI logic and accepting input data through props. 1) Define components: Use functions or classes to return React elements. 2) Rendering component: React calls render method or executes function component. 3) Multiplexing components: pass data through props to build a complex UI. The lifecycle approach of components allows logic to be executed at different stages, improving development efficiency and code maintainability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
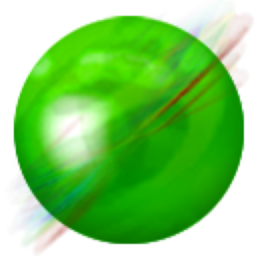
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
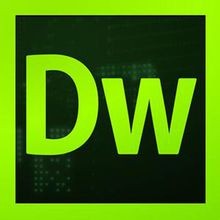
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version