JavaScript is a commonly used programming language used to add dynamic content and interactivity to web pages. Along with HTML and CSS, it forms the basic building block of modern websites. If you want to add dynamic elements to your website, JavaScript is indispensable. In this article, we will introduce how to use JavaScript.
- Embed JavaScript
There are two ways to embed JavaScript code in HTML. First, you can place script tags in your HTML between the
or . This means that the code will be executed once when the page loads. For example:<!DOCTYPE html> <html> <head> <script> function displayMessage() { alert("Hello, World!"); } </script> </head> <body> <button onclick="displayMessage()">Click me!</button> </body> </html>
The above code defines a function named displayMessage(), which will display a simple warning box. The function will be called when the user clicks the button.
The second method is to create a JavaScript file outside of the HTML document. This makes the code more organized and easier to manage. To use an external script, set the src attribute in the <script> tag to the path to the JavaScript file. For example: </script>
<!DOCTYPE html> <html> <head> <script src="myscript.js"></script> </head> <body> <button onclick="displayMessage()">Click me!</button> </body> </html>
In this example, the JavaScript code is in a file called myscript.js.
- Variables and Data Types
JavaScript supports a variety of data types, including strings, numeric values, Boolean values, objects, arrays, null values, and undefined values. To declare a variable, use the var keyword.
var name = "John"; var age = 30; var isMale = true;
In the above example, we defined three variables to store strings, numeric values and Boolean values respectively. You can use the typeof operator to determine the data type of a variable.
alert(typeof name); // "string" alert(typeof age); // "number" alert(typeof isMale); // "boolean"
- Operators and logical expressions
JavaScript supports a variety of operators, including arithmetic operators, comparison operators, and logical operators. In the following example, we define two variables x and y and demonstrate some commonly used operators.
var x = 10; var y = 5; var z = x + y; // 加法运算 var w = x - y; // 减法运算 var q = x * y; // 乘法运算 var r = x / y; // 除法运算 var s = x % y; // 取模运算 var t = x == y; // 等于运算符(返回false) var u = x > y; // 大于运算符(返回true) var v = (x > y) && (x < 20); // 逻辑AND运算符(返回true)
In the above code, we also demonstrated how to use logical expressions. The AND operator links two expressions together and returns true when both expressions are true.
- Functions
Functions are one of the basic building blocks in JavaScript. Functions are reusable pieces of code that can be used with other code. To define a function, use the function keyword.
function addNumbers(x, y) { return x + y; }
In this example, we define a function called addNumbers that accepts two numeric parameters and returns their sum. To call a function, use the function name followed by parentheses and pass any arguments required.
var sum = addNumbers(5, 10); // sum等于15
- Events
Events are another important concept in JavaScript. Events are actions that can be triggered, such as clicking a button or submitting a form. To handle an event, set an event handler, which is a function that will be called when the event occurs.
<button onclick="displayMessage()">Click me!</button>
In the above example, we defined a button and called a function named displayMessage when the button is clicked.
- DOM operations
Finally, let’s take a look at DOM operations in JavaScript. DOM (Document Object Model) is the programming interface of web pages, which allows JavaScript to access and modify elements and attributes in web pages. For example, to access an element, use the document object and specify the element's ID.
<p id="myParagraph">This is a paragraph.</p>
In the above example, we defined a paragraph element with the ID "myParagraph". Here's an example of how to modify its content using JavaScript.
var paragraph = document.getElementById("myParagraph"); paragraph.innerHTML = "This is a new paragraph.";
In the above code, we use the getElementById method to get the element and use the innerHTML attribute to set its content.
Summary
JavaScript is a powerful and flexible programming language that can be used to create interactive and dynamic web pages. This article introduces the basic concepts of JavaScript, including variables, data types, operators, functions, events, and DOM operations. Now you're ready to start writing your own JavaScript code!
The above is the detailed content of c How to use javascript. For more information, please follow other related articles on the PHP Chinese website!
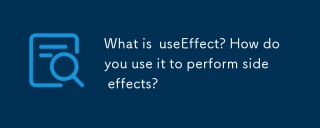
The article discusses useEffect in React, a hook for managing side effects like data fetching and DOM manipulation in functional components. It explains usage, common side effects, and cleanup to prevent issues like memory leaks.
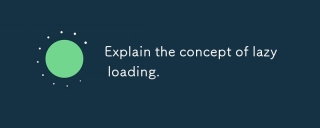
Lazy loading delays loading of content until needed, improving web performance and user experience by reducing initial load times and server load.
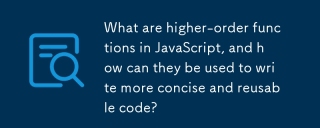
Higher-order functions in JavaScript enhance code conciseness, reusability, modularity, and performance through abstraction, common patterns, and optimization techniques.
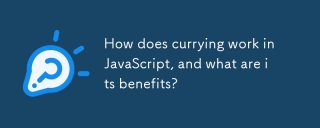
The article discusses currying in JavaScript, a technique transforming multi-argument functions into single-argument function sequences. It explores currying's implementation, benefits like partial application, and practical uses, enhancing code read
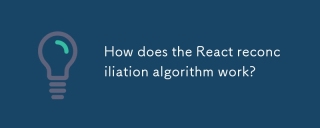
The article explains React's reconciliation algorithm, which efficiently updates the DOM by comparing Virtual DOM trees. It discusses performance benefits, optimization techniques, and impacts on user experience.Character count: 159
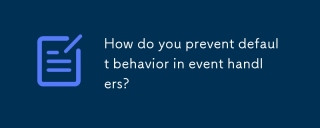
Article discusses preventing default behavior in event handlers using preventDefault() method, its benefits like enhanced user experience, and potential issues like accessibility concerns.
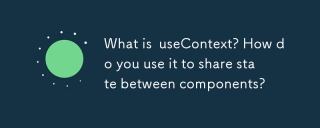
The article explains useContext in React, which simplifies state management by avoiding prop drilling. It discusses benefits like centralized state and performance improvements through reduced re-renders.
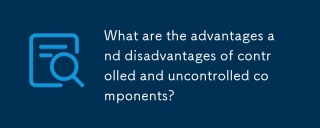
The article discusses the advantages and disadvantages of controlled and uncontrolled components in React, focusing on aspects like predictability, performance, and use cases. It advises on factors to consider when choosing between them.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
