


How to implement springboot verification code generation and verification functions
1.easy-captcha toolkit
There are many ways to generate verification codes. The easy-captcha toolkit is chosen here.
The original address of github is: easy-captcha tool kit
This module provides Java graphical verification code support, including gif, Chinese, arithmetic and other types, and is suitable for projects such as Java Web and JavaSE.
2 Add dependencies
First, you need to add the dependency configuration file of easy-captcha to the pom.xml file.
The import dependency statement has been written in the open source project, just copy and paste it.
<dependency> <groupId>com.github.whvcse</groupId> <artifactId>easy-captcha</artifactId> <version>1.6.2</version> </dependency>
3. Verification code character type
//生成验证码对象 SpecCaptcha captcha = new SpecCaptcha(130, 48, 5); //设置验证码的字符类型 captcha.setCharType(Captcha.TYPE_ONLY_NUMBER);4. Font Setting
//生成验证码对象 SpecCaptcha captcha = new SpecCaptcha(130, 48, 5); // 设置内置字体 captcha.setFont(Captcha.FONT_1); // 设置系统字体 captcha.setFont(new Font("楷体", Font.PLAIN, 28));5 Verification code image outputHere you can choose to output as a file stream, which is a common processing method. Of course, there are also some web projects that use base64-encoded images. Easy-captcha supports both methods.
The output method of base64 encoding is as follows:
SpecCaptcha specCaptcha = new SpecCaptcha(130, 48, 5); specCaptcha.toBase64(); // 如果不想要base64的头部data:image/png;base64, specCaptcha.toBase64(""); // 加一个空的参数即可The method of output to disk is as follows:
FileOutputStream outputStream = new FileOutputStream(new File("C:/captcha.png")) SpecCaptcha specCaptcha = new SpecCaptcha(130, 48, 5); specCaptcha.out(outputStream);This code generates a picture and saves it to the disk directory , here you can use the out() method that comes with the easy-captcha tool to output. When developing a web project, developers will use the output stream of the Response object to output the verification code. 6. Generate and display verification code6.1 BackendYou can create a KaptchaController class by creating a new method, which needs to be located in the controller package. Using GifCaptcha in the method can generate a PNG type verification code object and output it to the front end in the form of a picture stream for display. The code is as follows:
@Controller public class KaptchaController { @GetMapping("/kaptcha") public void defaultKaptcha(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse) throws Exception{ httpServletResponse.setHeader("Cache-Control","no-store"); httpServletResponse.setHeader("Pragma","no-cache"); httpServletResponse.setDateHeader("Expires",0); httpServletResponse.setContentType("image/gif"); //生成验证码对象,三个参数分别是宽、高、位数 SpecCaptcha captcha = new SpecCaptcha(130, 48, 5); //设置验证码的字符类型为数字和字母混合 captcha.setCharType(Captcha.TYPE_DEFAULT); // 设置内置字体 captcha.setCharType(Captcha.FONT_1); //验证码存入session httpServletRequest.getSession().setAttribute("verifyCode",captcha.text().toLowerCase()); //输出图片流 captcha.out(httpServletResponse.getOutputStream()); } }Add the defaultKaptcha() method in the controller , the interception processing path of this method is /kaptcha. After accessing the path on the front end, an image stream can be received and displayed on the browser page. 6.2 Front-endCreate a new kaptcha.html page in the static directory, and display the verification code in the page. The code is as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>验证码显示</title> </head> <body> <img src="/static/imghwm/default1.png" data-src="/kaptcha" class="lazy" onclick="this.src='/kaptcha?d='+new Date()*1" / alt="How to implement springboot verification code generation and verification functions" > </body> </html>By accessing the back-end/kaptcha Path, get the verification code image, and then display it in the img tag. Then write an onclick function that can dynamically switch and display a new verification code when the img tag is clicked. The path accessed when clicking is ’/kaptcha?d=' new Date()*1, that is, the original verification code path is followed by a timestamp parameter d. The timestamp will change, so each click will be a different request than the previous one. If this is not done, due to the browser's caching mechanism, the request may not be resent after clicking to refresh the verification code, which will result in the same verification code image being displayed for a period of time. After the coding is completed, start the Spring Boot project. After successful startup, open the browser and enter the test page address displayed by the verification code
http://localhost:8080/kaptchaThe effect is as follows:
The general approach is to save the currently generated verification code content after the backend generates the verification code. You can choose to save it in the session object, in the cache, or in the database. Then, return the verification code image and display it to the front-end page. After the user recognizes the verification code, he fills in the verification code in the corresponding input box on the page and sends a request to the backend. After receiving the request, the backend will verify the verification code entered by the user. If the verification code entered by the user is not equal to the previously saved verification code, a "verification code error" message will be returned and the subsequent process will not proceed. Only if the verification is successful will the subsequent process continue. 7.1 BackendAdd a new verify() method in the KaptchaController class, the code is as follows:
public String verify(@RequestParam("code") String code, HttpSession session){ if (!StringUtils.hasLength(code)){ return "验证码不能为空"; } String kaptchaCode = session.getAttribute("verifyCode")+""; if (!StringUtils.hasLength(kaptchaCode)||!code.toLowerCase().equals(kaptchaCode)){ return "验证码错误"; } return "验证成功"; }The verification code request parameter entered by the user is code,/verify The path intercepted and processed by this method. After basic non-empty verification, compare it with the verifyCode value previously saved in the session. If the two strings are not equal, a "verification code error" prompt will be returned. If they are the same, a "verification code successful" prompt will be returned. . 7.2 Front endCreate a new verify.html in the static directory. This page will display the verification code and also contains an input box and submit button for users to enter the verification code. The code is as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>验证码测试</title> </head> <body> <img src="/static/imghwm/default1.png" data-src="/kaptcha" class="lazy" onclick="this.src='/kaptcha?d='+new Date()*1" / alt="How to implement springboot verification code generation and verification functions" > <br> <input type="text" maxlength="5" id="code" placeholder="请输入验证码" /> <button id="verify">验证</button> <br> <p id="verifyResult"> </p> </body> <!--jqGrid依赖jQuery,因此需要先引入jquery.min.js文件,下方地址为字节跳动提供的cdn地址--> <script src="http://s3.pstatp.com/cdn/expire-1-M/jquery/3.3.1/jquery.min.js"></script> <script type="text/javascript"> $(function () { //验证按钮的点击事件 $('#verify').click(function () { var code = $('#code').val(); $.ajax({ type: 'GET',//方法类型 url: '/verify?code='+code, success: function (result) { //将验证结果显示在p标签中 $('#verifyResult').html(result); }, error:function () { alert('请求失败'); }, }); }); }); </script> </html>
在用户识别并呈现在页面上的验证码之后,他们可以在输入框中输入验证码并点击“验证”按钮。在JS代码中已经定义了“验证”按钮的点击事件,一旦点击,就会获取用户在输入框中输入的内容,并将其作为请求参数向后端发送请求,验证用户输入的验证码是否正确,后端在处理完成后会返回处理结果,拿到处理结果就显示在id为verifyResult的p标签中。
The above is the detailed content of How to implement springboot verification code generation and verification functions. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
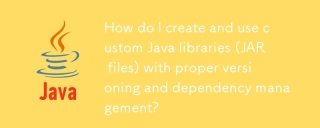
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
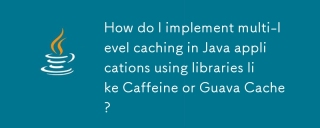
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
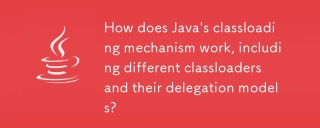
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
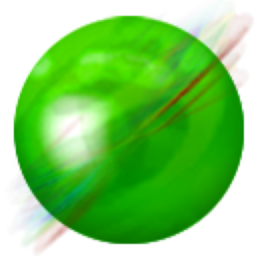
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
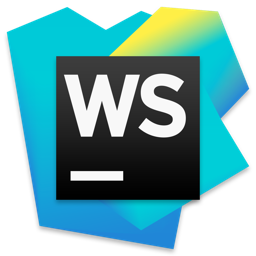
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version