Golang is an open source programming language developed by Google. It uses static typing and garbage collection mechanisms, and supports concurrent programming. Functions are an important component in Golang, and map, slice, struct and interface are commonly used data types in functions. This article will introduce the use of these data types in Golang.
1. Map type
map is a key-value pair data structure. In Golang, its use is very simple. The following is an example:
package main import "fmt" func main() { m := make(map[string]int) m["apple"] = 1 m["banana"] = 2 m["orange"] = 3 fmt.Println(m) }
In the above example, we first use the make function to create an empty map, and then add key-value pairs to the map in sequence. Finally, the map is output using the println function in the fmt package.
In addition to using the make function to create a map, we can also use literals to create a non-empty map, as shown below:
package main import "fmt" func main() { m := map[string]int{"apple": 1, "banana": 2, "orange": 3} fmt.Println(m) }
We can also use map when declaring variables Declare them together as follows:
package main import "fmt" func main() { var m map[string]int m = make(map[string]int) m["apple"] = 1 m["banana"] = 2 m["orange"] = 3 fmt.Println(m) }
Of course, if a key-value pair does not exist in the map, we can use the delete function to delete it, as shown below:
package main import "fmt" func main() { m := make(map[string]int) m["apple"] = 1 m["banana"] = 2 m["orange"] = 3 delete(m, "banana") fmt.Println(m) }
2. slice Type
slice is a dynamic array where elements can be added or removed at any time. The following is an example:
package main import "fmt" func main() { s := make([]string, 3) s[0] = "apple" s[1] = "banana" s[2] = "orange" s = append(s, "grape") fmt.Println(s) }
In the above example, we use the make function to create a slice with an element type of string and a length of 3. Then, we added 3 elements to the slice, and finally added a new element to the slice using the append function.
Of course, we can also use literals to create a non-empty slice, as shown below:
package main import "fmt" func main() { s := []string{"apple", "banana", "orange"} s = append(s, "grape") fmt.Println(s) }
We can also declare the slice together when declaring variables, as shown below:
package main import "fmt" func main() { var s []string s = make([]string, 3) s[0] = "apple" s[1] = "banana" s[2] = "orange" s = append(s, "grape") fmt.Println(s) }
3. Struct type
struct is a custom composite type that can store multiple types of data. The following is an example:
package main import "fmt" type Person struct { Name string Age int } func main() { p := Person{"Tom", 18} fmt.Println(p) }
In the above example, we use the type keyword to create a struct type named Person. Then, we create a variable p of type Person and assign a value to it.
In addition, we can also use structure pointers to access fields in the struct type, as shown below:
package main import "fmt" type Person struct { Name string Age int } func main() { p := &Person{"Tom", 18} p.Name = "Jerry" fmt.Println(p) }
4. Interface type
Interface is an An abstract type that can be used to represent any type. The following is an example:
package main import "fmt" type Animal interface { Eat() } type Cat struct { Name string } func (c *Cat) Eat() { fmt.Printf("%s is eating ", c.Name) } func main() { var a Animal a = &Cat{"Tom"} a.Eat() }
In the above example, we used the type keyword to create an interface type named Animal, which has only one method Eat. Then, we use the type keyword to create a struct type named Cat, which implements the Eat method of the Animal type. Finally, we create a variable a whose type is Animal and assign it to a variable of type Cat. When we call the Eat method of a, a sentence is output indicating that Cat is eating.
In addition, we can also use type assertions to determine whether a variable implements a certain interface, as shown below:
package main import "fmt" type Animal interface { Eat() } type Cat struct { Name string } func (c *Cat) Eat() { fmt.Printf("%s is eating ", c.Name) } func main() { var a Animal a = &Cat{"Tom"} _, ok := a.(*Cat) fmt.Println(ok) }
In the above example, we use the type keyword to create There is an interface type named Animal, which has only one method Eat. Then, we use the type keyword to create a struct type named Cat, which implements the Eat method of the Animal type. Finally, we create a variable a whose type is Animal and assign it to a variable of type Cat. We use type assertions to determine whether a implements the Cat type and outputs true.
The above is the detailed content of How to use map, slice, struct and interface types of Golang functions. For more information, please follow other related articles on the PHP Chinese website!
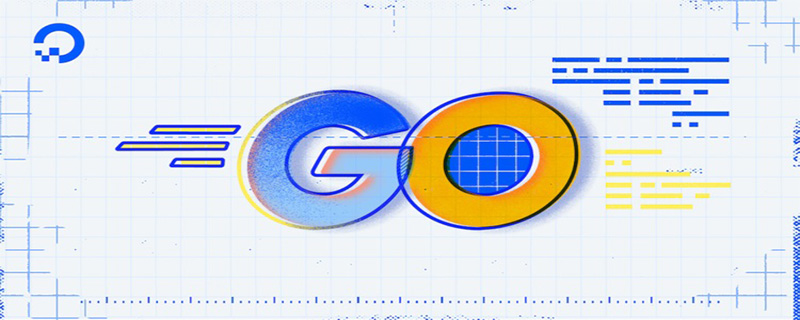
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
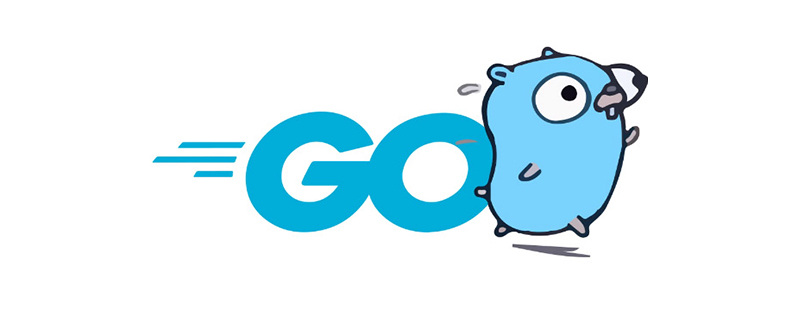
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
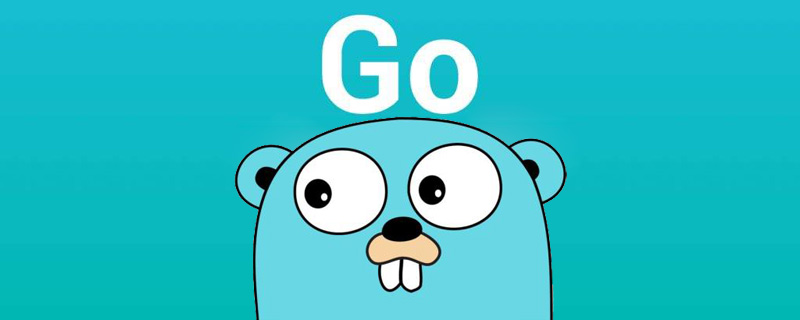
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
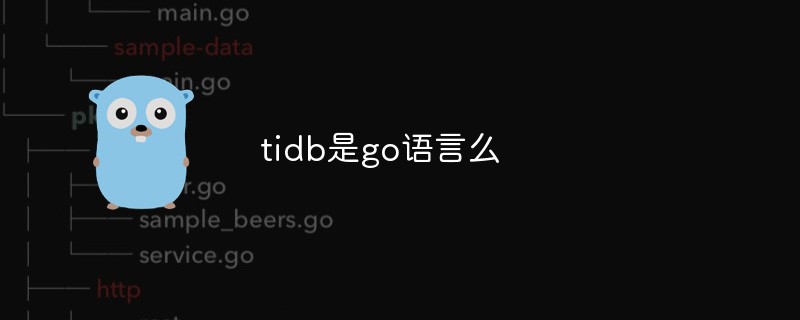
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
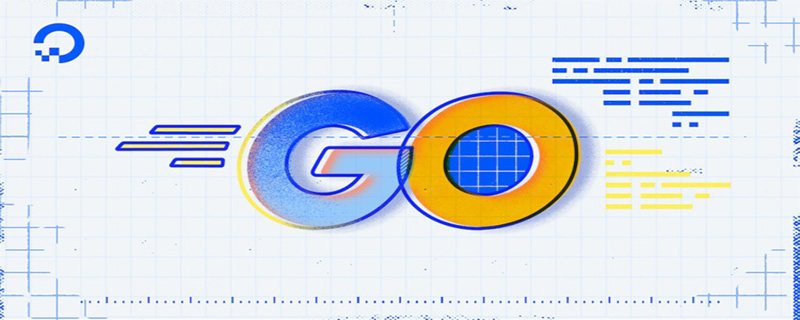
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
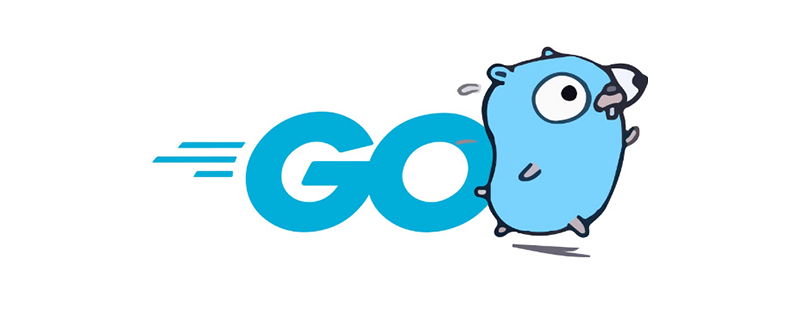
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
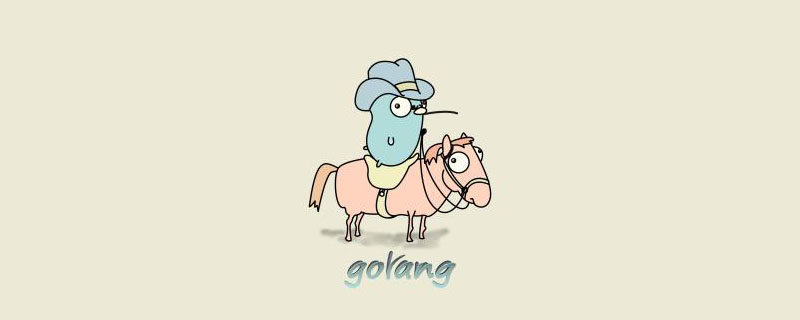
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
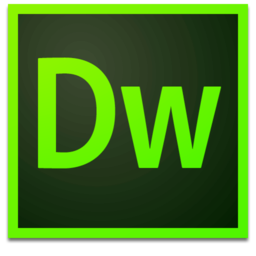
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use
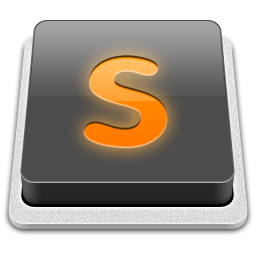
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
