In Golang, file opening is implemented through the built-in functions os.Open()
and os.Create()
. Closing a file is achieved through the Close()
method of the file object. After the file is processed, it is a good programming habit to close the file promptly. Closing a file can release the resources occupied by the file and avoid resource waste and subsequent impact of the file on the program.
The Close()
method of the file object is used to close the file. Its usage is very simple, just add a Close()
method after the file object. For example:
file, err := os.Open("./test.txt") if err != nil { panic(err) } // 处理文件 file.Close() // 关闭文件
In the above code, a file named test.txt
is first opened through os.Open()
. If an error occurs during the opening, throw an exception. Next, read and write files through the file
object. Finally, the file is closed through the file.Close()
method. Note that you must remember to close the file after all operations are completed, otherwise it will cause the leakage of file resources.
In addition to using the Close()
method to close the file, we can also use the defer
statement to delay closing the file. For example:
file, err := os.Open("./test.txt") if err != nil { panic(err) } defer file.Close() // 延迟关闭文件 // 处理文件
In this example, we use the defer
statement to delay the execution of the file.Close()
method until the end of the function, so that even if subsequent code appears If an exception occurs, there is no need to worry about the file not being closed. Using the defer
statement allows us to better organize the code and make the code more concise and readable.
Also note that the Close()
method will return a error
type value. If an error occurs when the file is closed, the Close()
method will return a non-empty error
value, and we can handle the exception by judging this value. For example:
file, err := os.Open("./test.txt") if err != nil { panic(err) } defer func() { if err := file.Close(); err != nil { panic(err) } }() // 处理文件
In this example, we use an anonymous function and the defer
statement to handle exceptions when the file is closed. Call the Close()
method in the anonymous function and determine whether an error occurs. If an error occurs, an exception is thrown. This allows us to better handle exceptions when files are closed.
In general, closing files is one of the skills that must be mastered in Golang programming. Closing files promptly can avoid resource waste and improve program security and robustness. Through the introduction of this article, I believe readers have a deeper understanding of how to close files. It is hoped that readers can operate files strictly according to the requirements in actual programming to ensure the normal operation of the program.
The above is the detailed content of golang close file. For more information, please follow other related articles on the PHP Chinese website!
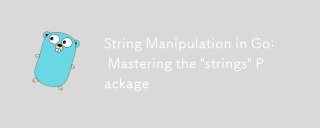
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
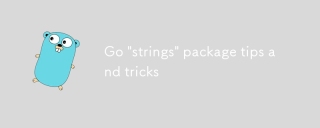
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
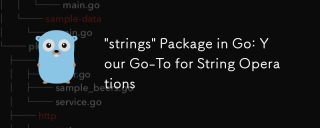
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
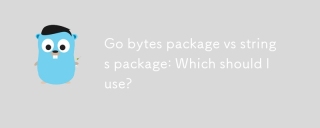
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
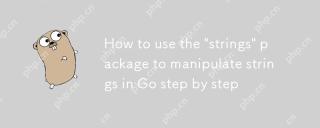
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
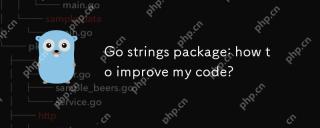
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
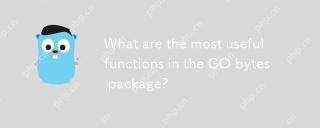
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
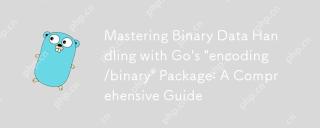
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
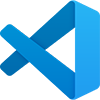
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
