In the Go language, channel (channel) is a very important concept. Channels provide a way to safely pass data between different Goroutines. By using channels, we can prevent multiple Goroutines from safely accessing shared memory space, thereby reducing the probability of race conditions in the program.
We know that when using a channel, the sender needs to write data to the channel, and then the receiver reads data from the channel. However, when the data in the channel has been received, how to ensure that the channel is closed normally? In this article, we will discuss how to close a channel and what you need to consider when closing a channel.
1. Why do you need to close the channel?
When using a channel, we usually use a for range loop to iterate the elements in the channel. For example, here is an example of reading data from a channel:
func readData(ch chan int) { for data := range ch { fmt.Println(data) } }
In the above code, we used a for range loop to iterate over the elements in the channel. But what if the channel never has data to read? In this case, the for range loop will always block waiting for the arrival of data, which will cause the program to fail to exit normally.
Therefore, we need a way to close the channel so that the program can exit normally after reading all the data. In addition, closing the channel can also remind the receiver that the channel has no data available, thereby preventing some unnecessary blocking or deadlock situations from occurring.
2. How to close the channel
In the Go language, you can use the built-in close function to close the channel. The signature of the close function is as follows:
func close(ch chan<- Type)
In the above signature, the
The following is an example of using the close function to close a channel:
func main() { ch := make(chan int) go func() { for i := 0; i < 10; i++ { ch <- i } close(ch) }() for data := range ch { fmt.Println(data) } }
In the above code, we first create an integer channel ch. Then, we use a Goroutine to continuously send data to the channel. After sending 10 numbers, we call the close function to close the channel.
Next, we use a for range loop to iterate through the elements in the channel and print them out. After all the data in the channel has been read, the for range loop will automatically exit.
3. Close unbuffered channels and buffered channels
In the above example, we demonstrate how to use the close function to close the unbuffered channel. When closing an unbuffered channel, all data must be read, otherwise blocking will occur. If there are goroutines blocking on the channel before it is closed, they will get the channel closed signal and exit.
When we close the buffered channel, there may be some data that has not been read. When closing a buffered channel, all data in the channel will be read first, and then a closing signal will be sent to all Goroutines.
We can demonstrate how to close a buffered channel by modifying the above example:
func main() { ch := make(chan int, 10) go func() { for i := 0; i < 10; i++ { ch <- i } close(ch) }() for data := range ch { fmt.Println(data) } }
In the above code, we declare the channel ch as a buffered channel and set the buffered channel to The length is set to 10. We use a Goroutine to continuously send data to the channel. The same operation as closing the unbuffered channel, we use the close function to close the channel after sending 10 numbers. In the main Goroutine, we use a for range loop to iterate through the data in the channel and output it.
If there are Goroutines that have been blocked on the channel after the channel is closed, they will get the channel closed signal and therefore exit. In addition, if there is unread data in the channel, these data will be automatically discarded after closing the channel.
4. Close the channel safely
When closing the channel, we need to pay attention to some things to ensure the normal operation of the program:
1. Do not perform concurrent read and write operations Close the channel
If the same channel is read and written in multiple Goroutines at the same time, and the close function is called in one of the Goroutines, it may cause exceptions in the read and write operations of other Goroutines on the channel.
Therefore, when using channels, we should try our best to avoid reading and writing the same channel in multiple Goroutines at the same time. If we must read and write to the same channel at the same time, we should use locks or other synchronization primitives to ensure concurrency safety.
2. Do not close the channel repeatedly
If we try to close the same channel multiple times, a panic exception will occur. Therefore, before closing the channel, we should make sure that the channel has not been closed yet.
A given channel can be checked using the ok-idit value:
v, ok := <- ch if ok { // ch 未关闭,执行读取操作 } else { // ch 已关闭,执行相应的操作 }
If the channel has been closed, the ok value will be false. We can use this way to check if the channel is closed before reading from it.
3. Do not close the channel in the Goroutine that receives the channel
Normally, we should use the close function in the Goroutine that sends the data to close the channel. Closing the channel in the Goroutine that receives the data may cause the above problems, such as exceptions in the read and write operations of other Goroutines on the channel, etc.
Therefore, when using channels, we should ensure that the channels are used correctly to avoid similar problems.
5. Summary
In this article, we discussed how to close a channel and what to consider when closing a channel. Channels are a very important concurrency primitive in the Go language. The correct way to use channels can effectively avoid problems such as race conditions and deadlocks in the program.
Therefore, when writing code, we should fully understand the principles and usage of channels, and use channels reasonably to achieve concurrent operations. When closing the channel, we need to pay attention to issues such as concurrent operations of the channel in multiple Goroutines and repeated channel closing to ensure the correct operation of the program.
The above is the detailed content of golang close chan. For more information, please follow other related articles on the PHP Chinese website!
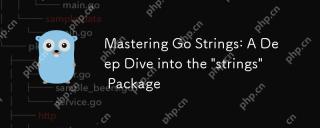
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
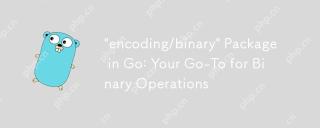
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
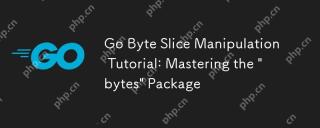
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.
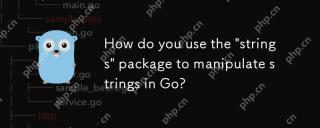
You can use the "strings" package in Go to manipulate strings. 1) Use strings.TrimSpace to remove whitespace characters at both ends of the string. 2) Use strings.Split to split the string into slices according to the specified delimiter. 3) Merge string slices into one string through strings.Join. 4) Use strings.Contains to check whether the string contains a specific substring. 5) Use strings.ReplaceAll to perform global replacement. Pay attention to performance and potential pitfalls when using it.
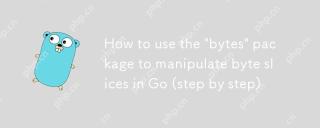
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli
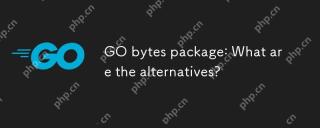
ThealternativestoGo'sbytespackageincludethestringspackage,bufiopackage,andcustomstructs.1)Thestringspackagecanbeusedforbytemanipulationbyconvertingbytestostringsandback.2)Thebufiopackageisidealforhandlinglargestreamsofbytedataefficiently.3)Customstru
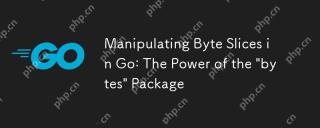
The"bytes"packageinGoisessentialforefficientlymanipulatingbyteslices,crucialforbinarydata,networkprotocols,andfileI/O.ItoffersfunctionslikeIndexforsearching,Bufferforhandlinglargedatasets,Readerforsimulatingstreamreading,andJoinforefficient
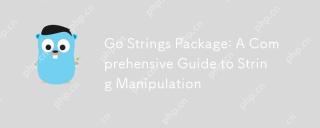
Go'sstringspackageiscrucialforefficientstringmanipulation,offeringtoolslikestrings.Split(),strings.Join(),strings.ReplaceAll(),andstrings.Contains().1)strings.Split()dividesastringintosubstrings;2)strings.Join()combinesslicesintoastring;3)strings.Rep


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
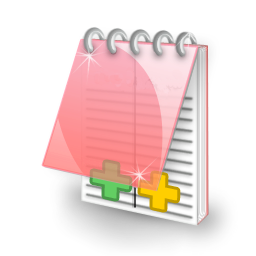
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
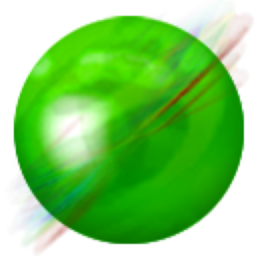
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
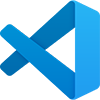
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
