With the rapid development and popularization of the Internet, the importance of network applications continues to rise. And all of this is inseparable from the HTTP protocol, because it is one of the cornerstones of the World Wide Web. In the development process of network applications, we often encounter situations where HTTP request forwarding is required. This article introduces how to use Golang to implement HTTP request forwarding.
1. Principle of HTTP request forwarding
HTTP request forwarding, as the name suggests, is to forward some client requests to other servers for processing. This process requires multiple HTTP protocol interactions, so you need to understand the principle of HTTP request forwarding.
The principle of HTTP request forwarding is mainly divided into the following steps:
1. The client sends an HTTP request to the forwarding server.
2. After the forwarding server receives the request, it determines whether the request needs to be forwarded to other servers for processing according to certain rules. If so, enter the third step, otherwise it directly returns a response to the client.
3. The forwarding server forwards the request to the target server. After multiple HTTP protocol interactions (including requests and responses), the target server finally returns the response result to the forwarding server.
4. The forwarding server returns the response result to the client.
2. Use Golang to implement HTTP request forwarding
In Golang, you can use the ReverseProxy type provided by the net/http package to implement HTTP request forwarding. ReverseProxy is a structure type that can proxy a set of HTTP requests to another server and return the corresponding response results. The main method of ReverseProxy is the ServeHTTP method, which accepts two parameters, one is the Writer type of the response result, and the other is the pointer type of the HTTP request.
The code for using Golang to implement HTTP request forwarding is as follows:
package main import ( "net/http" "net/http/httputil" "net/url" ) func main() { targetUrl, _ := url.Parse("http://www.example.com/") proxy := httputil.NewSingleHostReverseProxy(targetUrl) http.ListenAndServe(":8080", proxy) }
The above code first defines a target URL and parses it into a URL object; then uses the NewSingleHostReverseProxy method to create a ReverseProxy instance, And pass in the target URL as a parameter; finally use the ListenAndServe method to start an HTTP server and pass in the ReverseProxy instance created above.
3. Optional configuration of ReverseProxy
In addition to using the default configuration, ReverseProxy also supports some optional configurations, such as:
1. Modify the request body or response body: ReverseProxy provides the function of modifying the request body or response body, which can be achieved by modifying the Director and ModifyResponse methods.
2. Modify the requested URL: This can be achieved by modifying the Director method.
3. Custom error handling: Custom error handling can be implemented by modifying the ErrorLog field.
The following are some sample codes:
package main import ( "log" "net/http" "net/http/httputil" "net/url" ) func main() { targetUrl, _ := url.Parse("http://www.example.com/") proxy := httputil.NewSingleHostReverseProxy(targetUrl) // 修改请求体或者响应体 proxy.Director = func(req *http.Request) { req.Header.Set("Content-Type", "application/json") } proxy.ModifyResponse = func(response *http.Response) error { response.Header.Set("Content-Type", "application/json") return nil } // 修改请求的URL proxy.Director = func(req *http.Request) { req.Host = "www.anotherexample.com" req.URL.Scheme = "https" } // 自定义错误处理 logFile, _ := os.OpenFile("access.log", os.O_WRONLY|os.O_CREATE, 0755) errorLog := log.New(logFile, "proxy error: ", log.LstdFlags) proxy.ErrorLog = errorLog http.ListenAndServe(":8080", proxy) }
4. HTTP request forwarding practice case
A common HTTP request forwarding application scenario is load balancing. Load balancing is an important technology in distributed computing systems. Its purpose is to process requests through multiple servers to achieve high availability and high throughput of services. Common load balancing strategies include round robin, weighted round robin, minimum number of connections, etc.
The following is a simple load balancer implemented using Golang:
package main import ( "fmt" "log" "math/rand" "net/http" "net/http/httputil" "net/url" "strconv" "strings" "time" ) func main() { targetUrls := []string{"http://localhost:8001/", "http://localhost:8002/", "http://localhost:8003/"} // 随机数生成器 rand.Seed(time.Now().Unix()) balancedHandler := func(rw http.ResponseWriter, req *http.Request) { // 随机选择一个目标URL targetUrl := targetUrls[rand.Intn(len(targetUrls))] // 创建ReverseProxy target, _ := url.Parse(targetUrl) proxy := httputil.NewSingleHostReverseProxy(target) // 修改请求的Host req.URL.Host = target.Host req.URL.Scheme = target.Scheme req.Header.Set("X-Forwarded-Host", req.Header.Get("Host")) req.Host = target.Host // 记录日志 log.Printf("%s %s %s ", req.RemoteAddr, req.Method, req.URL) proxy.ServeHTTP(rw, req) } http.HandleFunc("/", balancedHandler) if err := http.ListenAndServe(":8080", nil); err != nil { fmt.Println(err) } } func getBalance(targetUrls []string) func() string { var last int32 = -1 return func() string { if len(targetUrls) == 0 { return "" } if len(targetUrls) == 1 { return targetUrls[0] } next := last for next == last { next = rand.Int31n(int32(len(targetUrls))) } last = next return targetUrls[last] } }
The above code first defines the target URL array, and then uses the random number generator provided by the rand package to randomly select a target URL. ; Then create a ReverseProxy instance, and use the Director method to modify the Host and other parameters of the request; Finally, record the request log, and call the ServeHTTP method of ReverseProxy to forward the request.
5. Summary
Through the introduction of this article, you have a preliminary understanding of the basic principles of HTTP request forwarding and its implementation in Golang. At the same time, we have given a simple load balancer example, hoping to be helpful to your development work.
Implementing an efficient, flexible and robust HTTP request forwarding program requires consideration of many aspects, including flow control, error handling, security, etc. We can achieve different needs by using the optional configurations provided by ReverseProxy .
The above is the detailed content of golang forward http. For more information, please follow other related articles on the PHP Chinese website!
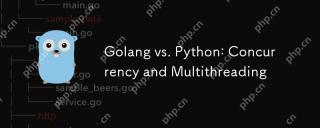
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
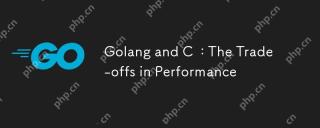
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
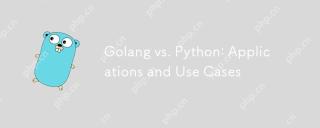
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
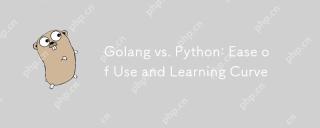
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
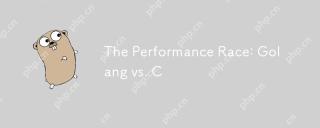
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
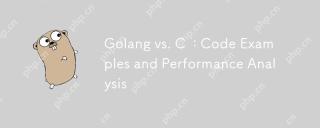
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
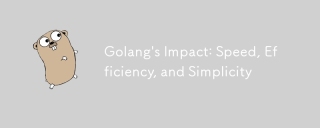
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
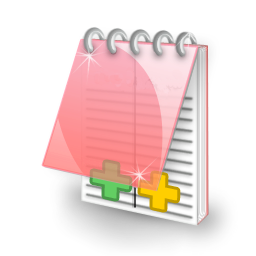
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment