Golang is a high-performance, distributed, and concurrent programming language that can be used to develop various types of applications. In a corporate environment, many companies use Microsoft Office to create and edit documents, spreadsheets, presentations, and other documents. However, Microsoft Office is commercial software and requires payment to use. So, is there an open source alternative?
In this article, we will explore how to implement an application similar to Microsoft Office using Golang. We'll cover how to use Golang to create and edit documents, spreadsheets, and presentations, as well as how to save them in different file formats.
1. Implement the Word document editor
First, we will implement an editor that can create and edit Word documents. This editor will use Golang's third-party library to read and write Word document files. We use the following code to import the relevant libraries:
import ( "fmt" "github.com/unidoc/unioffice/document" )
Next, we can open a Word document using the following code:
doc, err := document.Open("document.docx") if err != nil { fmt.Println(err.Error()) return }
Now, we can start editing the document. We can use the following code to read all paragraphs in the document:
for _, p := range doc.Paragraphs() { fmt.Println(p.Text()) }
To add a new paragraph in the document, we can use the following code:
p := doc.AddParagraph() t := p.AddRun() t.AddText("Hello, World!")
Add styles and formatting:
style := doc.StyleByName(document.StyleTypeDefault) p.SetStyle(style) t := p.AddRun() t.Properties().SetItalic(true) t.AddText("Hello, World!")
Finally, we can use the following code to save the modified Word document as a new file:
doc.SaveToFile("new_doc.docx")
2. Implement the Excel spreadsheet editor
Next, we will implement An Excel spreadsheet editor. This editor will use Golang's third-party libraries to read and write Excel files. We use the following code to import the relevant libraries:
import ( "fmt" "github.com/tealeg/xlsx" )
Next, we can open an Excel file using the following code:
xlsxFile, err := xlsx.OpenFile("file.xlsx") if err != nil { fmt.Println(err.Error()) return }
Now, we can start editing the spreadsheet. We can use the following code to read all cells in all worksheets:
for _, sheet := range xlsxFile.Sheets { for _, row := range sheet.Rows { for _, cell := range row.Cells { fmt.Println(cell.String()) } } }
To add a new cell in the spreadsheet, we can use the following code:
row := sheet.AddRow() cell := row.AddCell() cell.SetValue("Hello, World!")
Add format And style:
cell := row.AddCell() cell.SetStyle(style) cell.SetValue("Hello, World!")
Finally, we can use the following code to save the modified Excel file as a new file:
xlsxFile.Save("new_file.xlsx")
3. Implement the PowerPoint presentation editor
Finally , we will implement an editor that can create and edit PowerPoint presentations. This editor will use Golang's third-party library to read and write PowerPoint files. We use the following code to import the relevant libraries:
import ( "fmt" "github.com/umpc/go-sld" "github.com/umpc/go-sld/opts" )
Next, we can open a PowerPoint file using the following code:
presentation, err := sld.Open("presentation.pptx") if err != nil { fmt.Println(err.Error()) return }
Now, we can start editing the presentation. We can create a new slide and add some text to it using the following code:
slide, err := presentation.NewSlideWithLayout(opts.Custom) // 创建新幻灯片 if err != nil { fmt.Println(err.Error()) return } textbox := slide.NewTextboxWithSize("Hello, World!", 100, 100, 250, 100) // 添加文本框 textbox.Properties().SetFont("Arial", 36) // 修改字体
Finally, we can save the modified PowerPoint file as a new file using the following code:
presentation.Save("new_presentation.pptx")
Conclusion
By using Golang’s third-party libraries, we can easily implement an application similar to Microsoft Office. We can use Golang to create and edit Word documents, Excel spreadsheets, and PowerPoint presentations and save them in different file formats. By using these libraries, we can quickly develop high-performance and distributed applications that can handle large amounts of documents and data.
Of course, this is just a demonstration version implementation. Compared with Microsoft Office, there are still many shortcomings, such as interface design, more complete functions, etc. But this article enlightens us that Golang, an efficient programming language, can be used to develop various types of applications, whether it is commercial software or open source projects.
The above is the detailed content of Golang implements office. For more information, please follow other related articles on the PHP Chinese website!
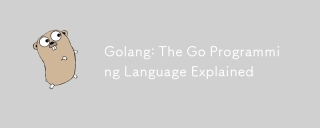
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
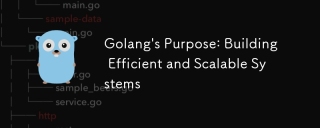
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
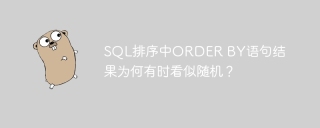
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
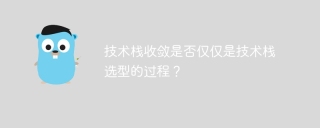
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
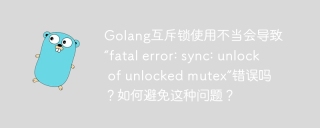
Golang ...
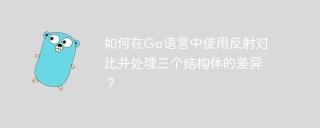
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...
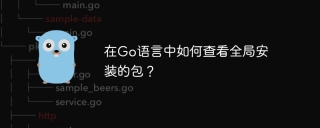
How to view globally installed packages in Go? In the process of developing with Go language, go often uses...
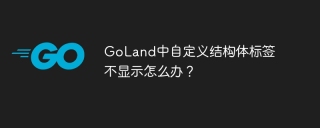
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor
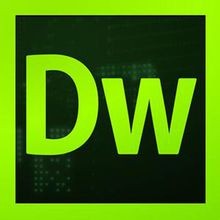
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use