Golang function switch statement application skills
As Golang continues to develop rapidly in recent years, it has become one of the preferred programming languages for many developers. Among Golang's many grammatical structures, the switch statement is undoubtedly a very important part. However, many developers may only use the simplest switch statement, but do not know much about the further application skills of the switch statement. This article will introduce some common application techniques of switch statements in Golang functions, in order to help readers better understand and apply switch statements.
- No expression required
In a general switch statement, we will provide an expression, so that the program can determine which case to execute based on the value of the expression statement. However, in Golang, we can use a switch statement without an expression to let the program jump to the first case statement that satisfies the condition. The following is an example:
package main import "fmt" func main() { i := 3 switch { case i < 3: fmt.Println("i is less than 3") case i == 3: fmt.Println("i is equal to 3") case i > 3: fmt.Println("i is greater than 3") } }
According to the value of i, the program will output "i is equal to 3". This feature is very useful when you need to evaluate multiple conditions in sequence, but don't want to use multiple if statements.
- You can use multiple expressions
In a general switch statement, we can only use one expression. However, in Golang, we can use multiple expressions separated by commas. The following is an example:
package main import "fmt" func main() { i, j := 3, 4 switch i, j { case 1, 2: fmt.Println("i is either 1 or 2") case 3, 4: fmt.Println("i is either 3 or 4") } }
According to the values of i and j, the program will output "i is either 3 or 4". This feature is very useful when multiple conditions need to be judged, but each condition is not mutually exclusive.
- You can use type assertions
In Golang, we can use type assertions to determine the type of a value. Therefore, in the switch statement, we can also use type assertions to perform type judgment. The following is an example:
package main import "fmt" func main() { var i interface{} = 1 switch i.(type) { case int: fmt.Println("i is an int") case float64: fmt.Println("i is a float64") case string: fmt.Println("i is a string") } }
The program will output "i is an int". This feature is very useful when you need to judge different types of values.
- You can use the fallthrough keyword
In Golang, we can use the fallthrough keyword to let the program execute the next case statement without conditional judgment. The following is an example:
package main import "fmt" func main() { i := 1 switch i { case 1: fmt.Println("i is 1") fallthrough case 2: fmt.Println("i is 2") } }
The program will output "i is 1" and "i is 2". This feature is very useful when multiple case statements need to be executed.
- You can use the default statement
In a general switch statement, if none of the conditions of a case statement is met, the program will exit the switch statement. However, in Golang, we can use default statement in switch statement to handle such situations. The following is an example:
package main import "fmt" func main() { i := 5 switch i { case 1: fmt.Println("i is 1") case 2: fmt.Println("i is 2") default: fmt.Println("i is neither 1 nor 2") } }
The program will output "i is neither 1 nor 2". This feature is very useful when a type of situation needs to be processed but there is no specific conditional judgment.
- You can use the switch statement as a function return value
In Golang, the switch statement can be used as a function return value. This feature is very useful because it makes programs more concise and readable. The following is an example:
package main import "fmt" func main() { i, j := 1, 2 switch { case i < j: fmt.Printf("%d is less than %d ", i, j) case i == j: fmt.Printf("%d is equal to %d ", i, j) case i > j: fmt.Printf("%d is greater than %d ", i, j) } }
The program will output "1 is less than 2". This feature is very useful in situations where various types of values need to be returned.
To sum up, the application skills of switch statements in Golang functions are very rich. We can flexibly use these skills when needed to make the program more streamlined, efficient and easy to read.
The above is the detailed content of Golang function switch statement application skills. For more information, please follow other related articles on the PHP Chinese website!
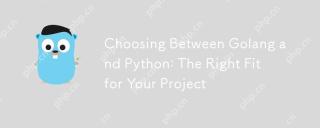
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
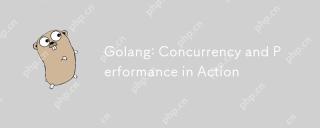
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
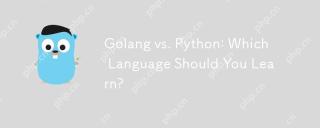
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
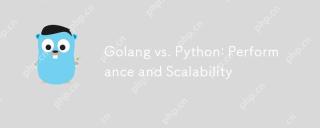
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
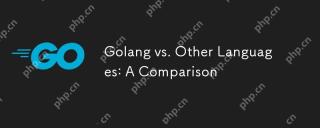
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
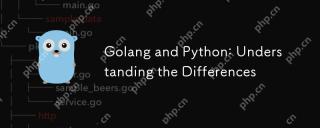
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
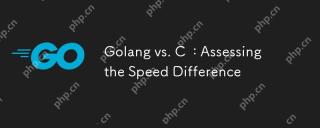
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
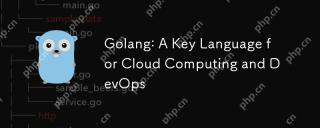
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
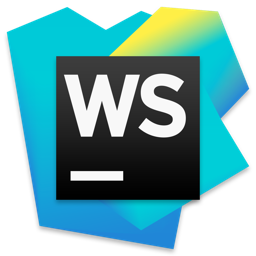
WebStorm Mac version
Useful JavaScript development tools
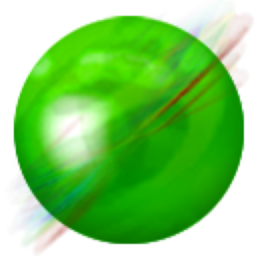
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.