In web development, Redis has become a very popular cache and database, and PHP is also a widely used web development language. Combining the two, we can use Redis in PHP to implement pattern matching functions, which greatly improves the performance and user experience of the website.
Redis is a memory-based data storage service, so its read and write speeds are very fast. At the same time, Redis also supports many advanced functions, such as publish/subscribe, transaction processing, Lua scripts, etc. In Redis, pattern matching is a very common and useful function. It can match qualified keys through template strings or regular expressions, making it easy to operate and manage data in applications.
In PHP, we can use the Redis extension to connect and operate the Redis server. The PHP Redis extension provides a rich API, including operation methods for basic data structures such as strings, hashes, lists, and sets. In these APIs, related methods for pattern matching are also provided. We can implement the pattern matching function through the methods provided by the Redis extension.
First, we need to use the method provided by the Redis extension to connect to the Redis server. The connection code is as follows:
$redis = new Redis(); $redis->connect('localhost', 6379);
After connecting to the Redis server, we can use the $redis
object to perform operations. For example, we can use the $redis->set()
method to set a key-value pair:
$redis->set('username', 'zhangsan');
At this time, the value of the 'username' key is set to 'zhangsan' . Correspondingly, we can also get the value of this key through the $redis->get()
method:
$value = $redis->get('username');
$value The value of the variable will be 'zhangsan'.
Next, we can start using the pattern matching method provided by the Redis extension. The most common pattern matching method is $redis->keys()
, which can be used to list keys that match a specified pattern. For example, we can use the following code to list all keys whose key names are prefixed with 'user:':
$keys = $redis->keys('user:*');
In the above code, 'user:' is the template for matching key names. Among them, '' is a wildcard character, which means matching any character. Therefore, 'user:*' will match any key name starting with 'user:'. In practical applications, we can also use regular expressions to match keys that meet conditions.
In addition to the $redis->keys()
method, the Redis extension also provides some other pattern matching methods, such as $redis->hScan()
, $redis->smembers()
, etc. They can be used to perform pattern matching operations on data structures such as hashes and sets.
Here is a complete example that demonstrates how to use the pattern matching method in the Redis extension:
$redis->set('user:1:name', '张三'); $redis->set('user:2:name', '李四'); $redis->set('user:3:name', '王五'); $keys = $redis->keys('user:*'); foreach ($keys as $key) { $name = $redis->get($key . ':name'); echo "用户名:$name "; }
In the above example, we stored the information of three users in Redis. Next, use the $redis->keys()
method to find all keys prefixed with 'user:', then iterate over these keys and retrieve them via $redis->get()
Method to get the value corresponding to the key name, that is, the user's name.
Through the above examples, I believe readers have a basic understanding of the pattern matching method in Redis extension. It should be noted that since Redis is a memory-based storage service, it is susceptible to problems such as memory leaks. In order to avoid these problems, we should design and use the Redis storage structure reasonably and clean up expired data regularly.
The above is the detailed content of Using Redis to implement pattern matching in PHP. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
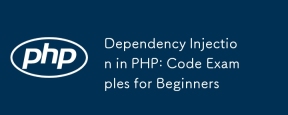
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
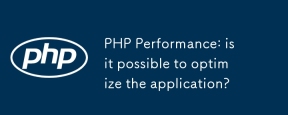
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
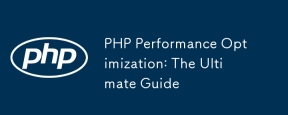
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
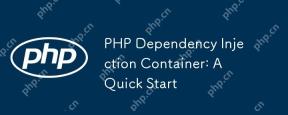
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
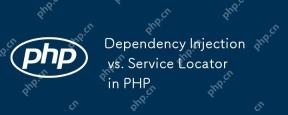
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
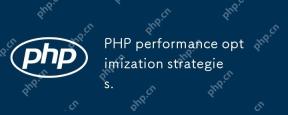
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
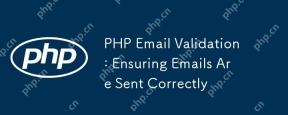
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
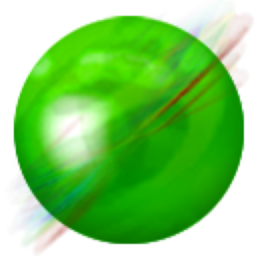
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
