Usage
In java, we often encounter scenarios that require type conversion of data. Converting String type data to Int type is a relatively common scenario. There are two main conversion methods:
1. Use the Integer.parseInt(String) method
2. Use the Integer.valueOf(String) method
The specific demo is as follows:
public void convert() { // 1.使用Integer.parseInt(String) String str1 = "31"; Integer num1 = Integer.parseInt(str1); System.out.print("字符串31转换为数字:"); System.out.println(num1); // 2.使用Integer.valueOf(String) String str2 = "32"; Integer num2 = Integer.valueOf(str2); System.out.print("字符串32转换为数字:"); System.out.println(num2); }
Execution results:
It can be seen according to the execution results, two Both methods can complete the conversion from string to integer.
Notes
But it should be noted that there is a prerequisite for using these two methods, that is, the content of the string to be converted must be pure numbers.
It is not difficult to find that the strings to be converted in the demo above are all strings composed of pure numbers such as "31" and "32". If other characters besides numbers appear in the string to be converted, , the program will throw an exception.
As shown in the demo below, add lowercase English letters to the string and wrap the code segment with try-catch statements to catch exceptions that may occur. (Because we already know that converting a string with letters into an integer will cause a digital format conversion exception, so we choose to catch NumberFormatException)
public void convert() { // 1.Integer.parseInt(String) try { String str1 = "31a"; Integer num1 = Integer.parseInt(str1); System.out.print("字符串31a转换为数字:"); System.out.println(num1); } catch (NumberFormatException e) { System.out.println("Integer.parseInt(String)方法执行异常"); e.printStackTrace(); } // 1.Integer.valueOf(String) try { String str2 = "32b"; Integer num2 = Integer.valueOf(str2); System.out.print("字符串32b转换为数字:"); System.out.println(num2); } catch (NumberFormatException e) { System.out.println("Integer.valueOf(String)方法执行异常"); e.printStackTrace(); } }
It can be seen from the execution results that this code is in Integer.parseInt(String ) method and the Integer.valueOf(String) position triggered NumberFormatException. The reason is that there are English letters in the converted string and cannot be converted into an integer type .
Performance comparison
We can use System.nanoTime() to view the time difference between the execution of the two methods
public static void convert() { // 1.Integer.parseInt(String) String str1 = "321"; long before1 = System.nanoTime(); Integer.parseInt(str1); long interval1 = System.nanoTime() - before1; System.out.print("Integer.parseInt(String)的执行时长(纳秒):"); System.out.println(interval1); // 1.Integer.valueOf(String) String str2 = "332"; long before2 = System.nanoTime(); Integer.valueOf(str2); long interval2 = System.nanoTime() - before2; System.out.print("Integer.valueOf(String)的执行时长(纳秒):"); System.out.println(interval2); }
Among them, interval1 The values of and interval2 respectively refer to the difference in system time before and after the execution of the two methods. The unit is nanoseconds. After executing it multiple times, it can be found that the execution time of the Integer.valueOf(String) method is shorter than the Integer.parseInt(String) method. Better performance
The above is the detailed content of How to convert String to Int in Java. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
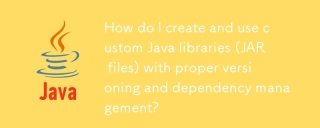
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
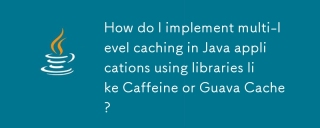
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
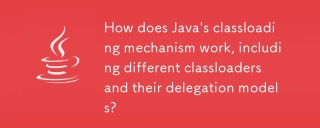
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
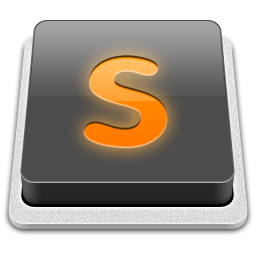
SublimeText3 Mac version
God-level code editing software (SublimeText3)