Go language is often used to develop web applications. In web applications, routing and page jumps are very important functions. This article will introduce how golang implements page jumps.
1. Static page jump
In web development, we often need to redirect users from one page to another. In golang, redirection can be achieved through the http.Redirect function. The definition of this function is:
func Redirect(w http.ResponseWriter, r *http.Request, url string, code int)
Among them, w refers to the response object sent to the client, r refers to the request object sent by the client, url refers to the URL address that needs to be jumped, and code refers to the status code. .
For example, in the following code, we define a route for /login and redirect it to another page:
package main import( "net/http" ) func main(){ http.HandleFunc("/login",func(w http.ResponseWriter, r *http.Request){ http.Redirect(w, r, "/welcome", 301) }) http.HandleFunc("/welcome",func(w http.ResponseWriter, r *http.Request){ w.Write([]byte("Welcome!")) }) http.ListenAndServe(":8080", nil) }
In the above code, when the user accesses /login , it will automatically jump to the /welcome page and display "Welcome!".
2. Template-based page jump
In web development, we usually need to pass some data to the target page. In golang, you can use HTML templates to implement page jumps with data.
The following is a simple sample code, where Guest and User are structure types:
package main import ( "html/template" "net/http" ) type Guest struct { Name string } type User struct { Name string Age int } func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { tmplt := template.Must(template.ParseFiles("templates/index.html")) data := Guest{ Name: "Guest", } tmplt.Execute(w, data) }) http.HandleFunc("/profile", func(w http.ResponseWriter, r *http.Request) { tmplt := template.Must(template.ParseFiles("templates/profile.html")) data := User{ Name: "John", Age: 25, } tmplt.Execute(w, data) }) http.ListenAndServe(":8080", nil) }
In the above code, we define two routes, "/" and "/profile" . When the user accesses "/", the template "templates/index.html" will be loaded, the data of the Guest structure will be passed to the template for rendering and the result will be returned. When a user accesses "/profile", the template "templates/profile.html" will be loaded, the data of the User structure will be passed to the template for rendering and the result will be returned.
Go language template tags can be used in HTML templates to insert dynamic data into the page. For example: In the templates/index.html file, you can use the following code to output the name of the Guest:
<!DOCTYPE html> <html> <head> <title>Hello World!</title> </head> <body> <h1 id="Hello-Name">Hello, {{.Name}}!</h1> <a href="/profile">Enter Profile</a> </body> </html>
In the templates/profile.html file, you can also use similar code to output the User's name and age. :
<!DOCTYPE html> <html> <head> <title>User Profile</title> </head> <body> <h1 id="User-Profile">User Profile</h1> <ul> <li>Name: {{.Name}}</li> <li>Age: {{.Age}}</li> </ul> </body> </html>
Summary:
- In golang, page jump can be achieved through the http.Redirect function.
- Template-based page jumps can achieve dynamic data output through HTML templates.
- The template engine of Go language has powerful functions and can process HTML/XML documents, transforming from process-oriented to object-oriented.
The above is the detailed content of How to jump to the page in golang. For more information, please follow other related articles on the PHP Chinese website!
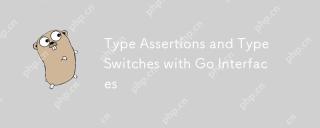
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
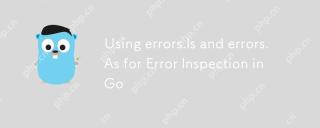
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
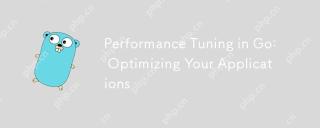
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement
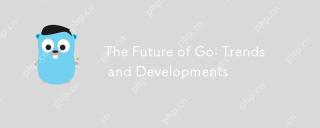
Go'sfutureisbrightwithtrendslikeimprovedtooling,generics,cloud-nativeadoption,performanceenhancements,andWebAssemblyintegration,butchallengesincludemaintainingsimplicityandimprovingerrorhandling.
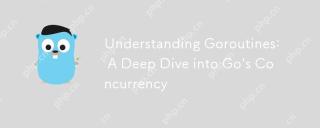
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
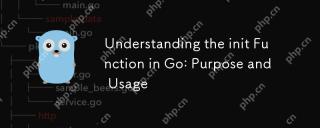
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
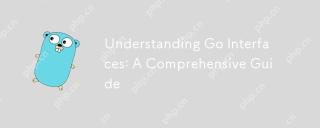
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
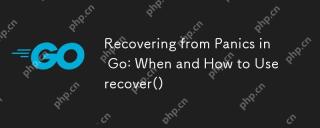
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
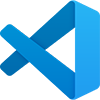
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use
