Converting text encoding in Golang is a common requirement. Especially when processing Chinese data, encoding conversion is often required to ensure data correctness, readability, and operability. Golang provides UTF-8 encoding support in the standard library, and for other encodings, you can use third-party libraries such as iconv and golang.org/x/text/encoding.
This article will introduce how to perform encoding conversion in Golang, as well as common encoding problems and solutions.
- UTF-8 encoding
UTF-8 is a Unicode character encoding method used to encode and save Unicode characters. In Golang, string types use UTF-8 encoding by default.
The Unicode package provides functions for converting between UTF-8 encoding and Unicode code points. For example, to convert a string into a slice of Unicode code points, you can use the following function:
func []rune(s string) []rune
This function returns a slice containing all Unicode code points in the source string.
- Other encoding formats
For other encoding formats, the Golang official library does not provide a direct conversion method. We can use third-party libraries for encoding conversion.
iconv is an open source character encoding conversion library that supports conversion between multiple character set encodings. Install iconv:
go get github.com/djimenez/iconv-go
To use iconv for encoding conversion, you can refer to the following code:
package main import ( "github.com/djimenez/iconv-go" "fmt" ) func main() { // 转换 gbk 编码到 utf-8 converted, err := iconv.ConvertString(original, "gbk", "utf-8") if err != nil { fmt.Println("转换失败:", err) } else { fmt.Println(converted) // 爱奇艺 } }
We can also use the golang.org/x/text/encoding package for encoding conversion. This package provides standard character set encodings and decoders, supporting UTF-8, UTF-16, GBK, GB18030, and various other character set encodings.
Using the golang.org/x/text/encoding package for encoding conversion, you can refer to the following code:
package main import ( "golang.org/x/text/encoding/simplifiedchinese" "golang.org/x/text/transform" "bytes" "fmt" ) func main() { // 转换 gbk 编码到 utf-8 decoder := simplifiedchinese.GBK.NewDecoder() converted, _, _ := transform.Bytes(decoder, []byte(original)) fmt.Println(string(converted)) // 爱奇艺 }
- Solving encoding problems
In actual programming , sometimes you will encounter encoding problems, such as garbled characters, etc. These issues are usually caused by inconsistent encoding or incorrect conversions.
How to solve the encoding problem? Below we introduce common solutions:
(1) Confirm the encoding format of the source string
Before performing encoding conversion, you first need to confirm the encoding format of the original string, such as whether it is GBK , UTF-8 or other encoding formats. If you are not sure about the encoding format, you can try converting using multiple encoding formats until you get the correct result.
(2) Confirm the target encoding format
Before performing encoding conversion, you need to confirm the target encoding format, such as converting to UTF-8 or GBK. If you are not sure about the encoding format, you can try converting the data into multiple encoding formats until you get the correct result.
(3) Use the correct converter
When performing encoding conversion, the correct converter should be used. For example, if you convert GBK to UTF-8, you should use a GBK decoder and a UTF-8 encoder. If you use the wrong decoder or encoder, it will cause problems such as data corruption or garbled characters.
(4) Use standard libraries and third-party libraries
In Golang, both the standard library and third-party libraries provide a wealth of encoding conversion methods and tools, which can be used when encountering encoding problems. use.
- Summary
Encoding conversion in Golang is a common need, especially when processing Chinese data. Through the introduction of this article, we can have a preliminary understanding of how to perform encoding conversion in Golang and how to solve common encoding problems. In the actual development process, it is necessary to select appropriate encoding conversion methods and tools based on specific scenarios and needs to achieve the best encoding conversion effect.
The above is the detailed content of How to convert Chinese in golang. For more information, please follow other related articles on the PHP Chinese website!
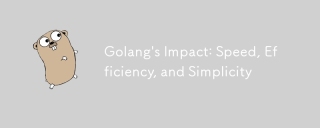
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
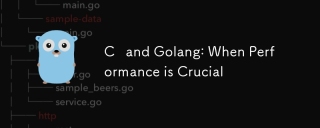
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
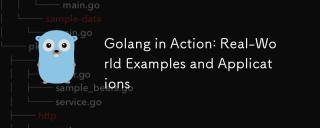
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
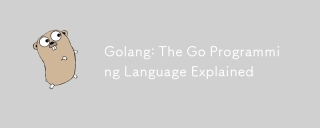
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
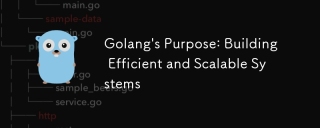
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
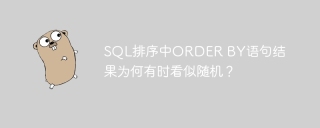
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
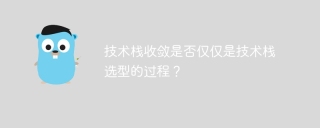
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
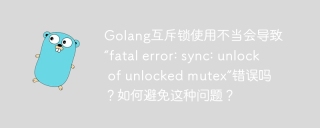
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
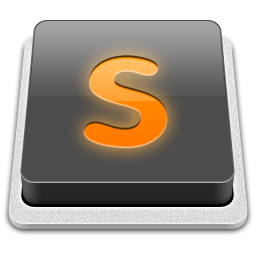
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
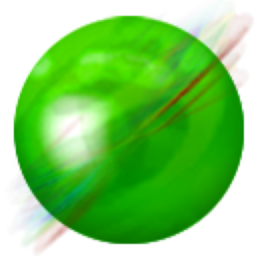
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment