When using golang to write files, sometimes the file content will be garbled. This is because golang uses UTF-8 encoding to write files by default. If you use other encodings when writing files, garbled characters will occur.
So, how can we avoid the problem of garbled files written in golang? Here are some solutions:
- Use UTF-8 encoding
If you want to avoid garbled characters, the easiest way is to use UTF-8 encoding. In golang, you can set the encoding when writing files through the following code:
file, err := os.Create("test.txt") if err != nil { log.Fatal(err) } defer file.Close() file.WriteString("") // 写入UTF-8的BOM字符 writer := bufio.NewWriter(file) encoder := unicode.UTF8.NewEncoder() writer = transform.NewWriter(writer, encoder) _, err = writer.WriteString("这是一段中文文本") if err != nil { log.Fatal(err) }
The BOM character is used in this code to indicate that the file uses UTF-8 encoding. Ensure that the written text is UTF-8 encoded by using the UTF8 encoder encoder and converter transform on the write stream.
- Use the specified encoding
In addition to using UTF-8 encoding, golang also supports many other encodings. You can use the encoding/pinyin package to convert text to a specific encoding, such as GBK or Big5.
The following is a sample code that uses GBK encoding to write text to a file:
file, err := os.Create("test.txt") if err != nil { log.Fatal(err) } defer file.Close() writer := bufio.NewWriter(file) encoder := simplifiedchinese.GBK.NewEncoder() writer = transform.NewWriter(writer, encoder) _, err = writer.WriteString("这是一段中文文本") if err != nil { log.Fatal(err) }
This code uses simplifiedchinese.GBK.NewEncoder() to encode the written text. You can choose other encoding formats as needed.
- Use third-party libraries
In addition to using golang’s standard library to write files, you can also use third-party libraries, such as github.com/axgle/mahonia. Solve the problem of garbled characters.
The following is a sample code that uses the mahonia library to write text to a file:
import ( "github.com/axgle/mahonia" ) file, err := os.Create("test.txt") if err != nil { log.Fatal(err) } defer file.Close() writer := bufio.NewWriter(file) enc := mahonia.NewEncoder("gbk") writer.WriteString(enc.ConvertString("这是一段中文文本")) writer.Flush()
This code uses mahonia.NewEncoder("gbk") to encode the written text.
Summary
The best way to avoid garbled characters when writing files in golang is to specify the correct encoding format when writing code. UTF-8 encoding is used by default. If you want to use other encoding formats, you can use an encoder or a third-party library to do so.
The above is the detailed content of Golang writes garbled files. For more information, please follow other related articles on the PHP Chinese website!
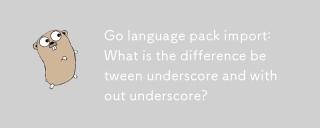
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
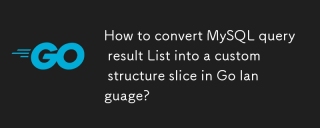
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
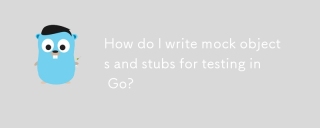
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
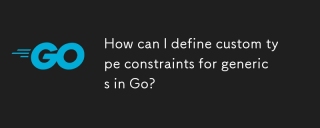
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
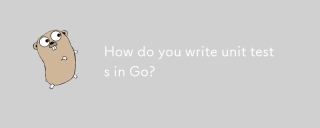
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
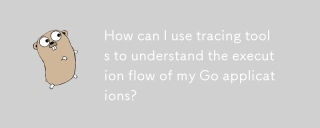
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
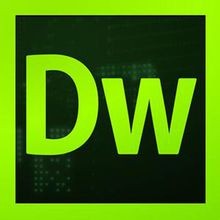
Dreamweaver CS6
Visual web development tools
