Golang is an efficient, concise and fast programming language, and has gradually become an important tool in the fields of Web development, network programming, big data processing and other fields. As a Golang developer, you need to understand how to use various component packages to simplify and speed up your development process.
This article will introduce some of Golang’s main component packages and their usage to help you better understand how to use them to develop efficient and stable applications.
- net/http
net/http is the most basic component in Golang. It provides many out-of-the-box methods and functions, including creating HTTP servers, handling HTTP requests and responses, etc. The following is a simple HTTP server example:
package main import ( "fmt" "net/http" ) func handleIndex(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello World!") } func main() { http.HandleFunc("/", handleIndex) http.ListenAndServe(":8080", nil) }
In this simple HTTP server example, we use the http.HandleFunc function to register a handler function handleIndex for the root route, and then use the http.ListenAndServe function Start the server. Once started, our server will listen for requests on localhost:8080.
- database/sql
Golang's database/sql package provides a common set of functions, allowing you to access common relational databases, such as MySQL, PostgreSQL, and SQLite. It provides a standard set of methods to perform queries, create and delete tables, and perform transaction management. Here is an example of connecting to a MySQL database and querying data:
package main import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/database") if err != nil { panic(err) } defer db.Close() rows, err := db.Query("SELECT * FROM users") if err != nil { panic(err) } defer rows.Close() for rows.Next() { var id int var name string var email string err = rows.Scan(&id, &name, &email) if err != nil { panic(err) } fmt.Println(id, name, email) } }
In this example, we use github.com/go-sql-driver/mysql driver to connect to the MySQL database. We then executed a SELECT statement and iterated through each row of data. Note that we use a defer statement to close the database connection and result set.
- encoding/json
Golang's encoding/json package provides a set of functions for encoding data into JSON format, or decoding from JSON format into Golang data structures. It is a widely used component for RESTful API, front-end development and data exchange scenarios. Here is an example of encoding a struct into JSON format and sending it to an HTTP response:
package main import ( "encoding/json" "net/http" ) type Person struct { Name string `json:"name"` Age int `json:"age"` } func handleJSON(w http.ResponseWriter, r *http.Request) { person := &Person{"Alice", 25} json, err := json.Marshal(person) if err != nil { panic(err) } w.Header().Set("Content-Type", "application/json") w.Write(json) } func main() { http.HandleFunc("/", handleJSON) http.ListenAndServe(":8080", nil) }
In this example, we define a Person struct and use the json.Marshal function to It is encoded in JSON format. We then send this JSON data in the HTTP response. The response received by the client will be a JSON object containing a string named "name" and an integer named "age".
- crypto
Golang’s crypto package provides a set of encryption and hashing algorithms for data protection and secure storage. It includes encryption and hashing algorithms such as AES, DES, RSA, SHA and MD5 to help you protect sensitive data and ensure it cannot be tampered with. Here is an example of using AES to encrypt and decrypt data:
package main import ( "crypto/aes" "crypto/cipher" "crypto/rand" "encoding/base64" "fmt" "io" ) func main() { key := []byte("super-secret-key") plaintext := []byte("Hello World!") block, err := aes.NewCipher(key) if err != nil { panic(err) } ciphertext := make([]byte, aes.BlockSize+len(plaintext)) iv := ciphertext[:aes.BlockSize] if _, err := io.ReadFull(rand.Reader, iv); err != nil { panic(err) } stream := cipher.NewCFBEncrypter(block, iv) stream.XORKeyStream(ciphertext[aes.BlockSize:], plaintext) fmt.Printf("Ciphertext: %s ", base64.StdEncoding.EncodeToString(ciphertext)) decrypted := make([]byte, len(plaintext)) stream = cipher.NewCFBDecrypter(block, iv) stream.XORKeyStream(decrypted, ciphertext[aes.BlockSize:]) fmt.Printf("Decrypted: %s ", decrypted) }
In this example, we create an AES encryption block using the aes.NewCipher function and initialize the stream cipher with a randomly generated IV . We then encrypt Plaintext using a stream cipher and save the result into Ciphertext. Finally, we use the same encrypted block and IV to decrypt the data.
This is a simpler example, but it can help you understand how to use crypto packages to protect your data.
Summary
Golang provides many powerful component packages that can help you write efficient applications faster and easier. In this article, we introduce some commonly used component packages and their usage, including HTTP servers, databases, JSON encoding and decoding, and data encryption.
By mastering the usage of these component packages, you can better utilize the advantages of Golang, improve development efficiency and code quality, and make your applications faster, more stable, and safer.
The above is the detailed content of Golang component package usage. For more information, please follow other related articles on the PHP Chinese website!
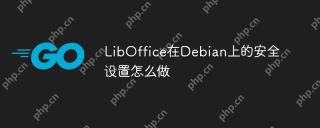
Ensuring overall security on Debian systems is crucial to protecting the running environment of applications such as LibOffice. Here are some general recommendations for improving system security: System updates regularly update the system to patch known security vulnerabilities. Debian12.10 released security updates that fixed a large number of security vulnerabilities, including some critical software packages. User permission management avoids the use of root users for daily operations to reduce potential security risks. It is recommended to create a normal user and join the sudo group to limit direct access to the system. The SSH service security configuration uses SSH key pairs to authenticate, disable root remote login, and restrict login with empty passwords. These measures can enhance the security of SSH services and prevent
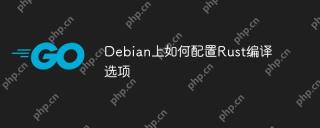
Adjusting Rust compilation options on Debian system can be achieved through various ways. The following is a detailed description of several methods: Use the rustup tool to configure and install rustup: If you have not installed rustup yet, you can use the following command to install: curl--proto'=https'--tlsv1.2-sSfhttps://sh.rustup.rs|sh Follow the prompts to complete the installation process. Set compilation options: rustup can be used to configure compilation options for different toolchains and targets. You can set compilation options for a specific project using the rustupoverride command. For example, if you want to set a specific Rust version for a project
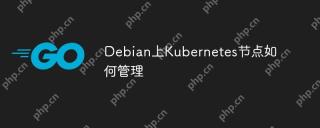
Managing Kubernetes (K8S) nodes on a Debian system usually involves the following key steps: 1. Installing and configuring Kubernetes components preparation: Make sure that all nodes (including master nodes and worker nodes) have the Debian operating system installed and meet the basic requirements for installing a Kubernetes cluster, such as sufficient CPU, memory and disk space. Disable swap partition: In order to ensure that kubelet can run smoothly, it is recommended to disable swap partition. Set firewall rules: allow necessary ports, such as ports used by kubelet, kube-apiserver, kube-scheduler, etc. Install container
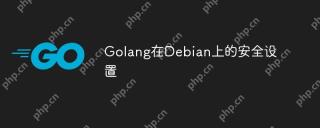
When setting up a Golang environment on Debian, it is crucial to ensure system security. Here are some key security setup steps and suggestions to help you build a secure Golang development environment: Security setup steps System update: Make sure your system is up to date before installing Golang. Update the system package list and installed packages with the following command: sudoaptupdatesudoaptupgrade-y Firewall Configuration: Install and configure a firewall (such as iptables) to limit access to the system. Only necessary ports (such as HTTP, HTTPS, and SSH) are allowed. sudoaptininstalliptablessud
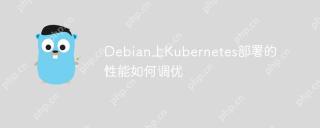
Optimizing and deploying Kubernetes cluster performance on Debian is a complex task involving multiple aspects. Here are some key optimization strategies and suggestions: Hardware resource optimization CPU: Ensure that sufficient CPU resources are allocated to Kubernetes nodes and pods. Memory: Increases the memory capacity of the node, especially for memory-intensive applications. Storage: Use high-performance SSD storage and avoid using network file systems (such as NFS) as they may introduce latency. Kernel parameter optimization edit /etc/sysctl.conf file, add or modify the following parameters: net.core.somaxconn: 65535net.ipv4.tcp_max_syn
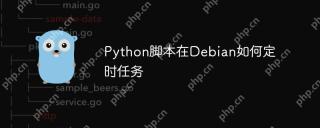
In the Debian system, you can use cron to arrange timed tasks and realize the automated execution of Python scripts. First, start the terminal. Edit the crontab file of the current user by entering the following command: crontab-e If you need to edit the crontab file of other users with root permissions, please use: sudocrontab-uusername-e to replace username with the username you want to edit. In the crontab file, you can add timed tasks in the format as follows: *****/path/to/your/python-script.py These five asterisks represent minutes (0-59) and small
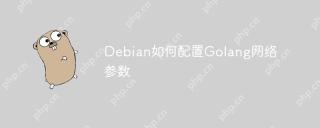
Adjusting Golang's network parameters in Debian system can be achieved in many ways. The following are several feasible methods: Method 1: Temporarily set environment variables by setting environment variables: Enter the following command in the terminal to temporarily set environment variables, and this setting is only valid in the current session. exportGODEBUG="gctrace=1netdns=go" where gctrace=1 will activate garbage collection tracking, and netdns=go will make Go use its own DNS resolver instead of the system default. Set environment variables permanently: add the above command to your shell configuration file, such as ~/.bashrc or ~/.profile
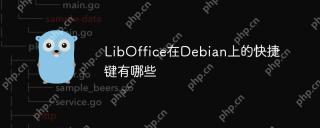
The shortcut keys for customizing LibOffice on Debian systems can be adjusted through system settings. Here are some commonly used steps and methods to set LibOffice shortcut keys: Basic steps to set LibOffice shortcut keys Open system settings: In the Debian system, click the menu in the upper left corner (usually a gear icon), and select "System Settings". Select a device: In the system settings window, select "Device". Select a keyboard: On the Device Settings page, select Keyboard. Find the command to the corresponding tool: In the keyboard settings page, scroll down to the bottom to see the "Shortcut Keys" option. Clicking it will bring a window to a pop-up. Find the corresponding LibOffice worker in the pop-up window


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
