Go is a programming language that supports pointers. Pointers are widely used in Go programming because many operations require direct manipulation of memory addresses. Learning how to use pointers correctly can make your code more efficient and help solve problems in certain scenarios.
What is a pointer
A pointer is a special variable that stores an address. This address points to the address of another variable stored in memory. In other words, a pointer is the memory address of a variable.
For example:
var a int = 10 var p *int = &a
Here, a
stores an integer value 10
, while p
is a ## A pointer variable of type #int stores the memory address of
a. By
&a taking out the address of
a and storing it to
p, we can access the address stored by
a through
p value.
&
You can get the pointer address of the variable by adding & before the variable. For example:
var a int = 10 var p *int = &aDereference
*
Pointer variables can be dereferenced by prefixing them with *. This converts the pointer variable to the original value pointed to by the pointer. For example:
var a int = 10 var p *int = &a fmt.Println(*p) // 输出:10Pointer arithmetic
and
-
Pointer variables can perform addition and subtraction operations. This operation will move the address pointed to by the pointer. For example: var a [3]int = [3]int{1, 2, 3} var p *int = &a[0] fmt.Println(*p) // 输出:1 p++ fmt.Println(*p) // 输出:2In this example, we define an integer array
a and store the address of its first element in the pointer variable
p. We first print out the value pointed to by
p, then move the pointer to the second element in the array via
p and print out the new pointer value again.
func swap(a, b *int) { temp := *a *a = *b *b = temp } func main() { x, y := 1, 2 swap(&x, &y) fmt.Println(x, y) // 输出:2 1 }In this example, we define a
swap function that accepts two integer pointers as parameters. Inside the function, we dereference the pointer and swap the values of the two variables. When we call the function, we pass the addresses of the two variables to the
swap function, so that the function can directly operate the memory addresses of the two variables.
new function to allocate memory and return the address of the newly allocated memory. For example:
p := new(int) *p = 10 fmt.Println(*p) // 输出:10In this example, we use the
new function to allocate a new
int type of memory space and store the address in
p middle. We then set the value of the newly allocated memory to
10 by
*p = 10. Finally, we use
*p to access the value and print it out.
func add(a, b int) (int, *error) { if a < 0 || b < 0 { err := errors.New("arguments must be non-negative") return 0, &err } return a + b, nil } func main() { result, err := add(1, -2) if err != nil { fmt.Println(err) return } fmt.Println(result) }In this example, we define the
add function to calculate the sum of the input parameters. If either argument is negative, we create an error object and return it as a pointer. In the
main function, we check whether the error pointer is
nil, and if not, print the error message, otherwise print the return value of the function.
The above is the detailed content of How to use golang pointers. For more information, please follow other related articles on the PHP Chinese website!
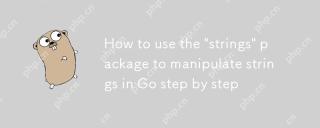
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
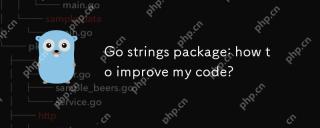
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
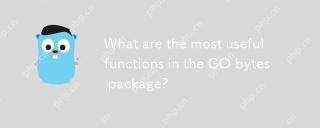
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
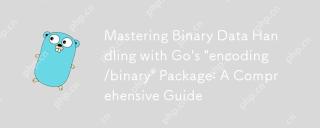
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
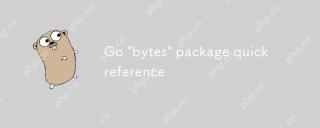
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
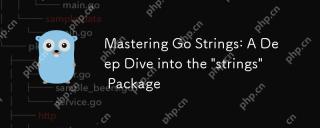
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
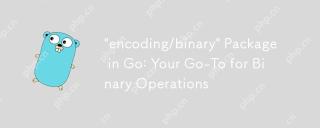
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
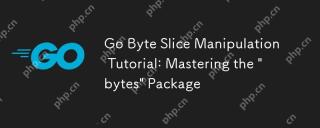
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
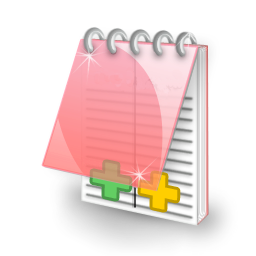
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
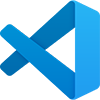
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
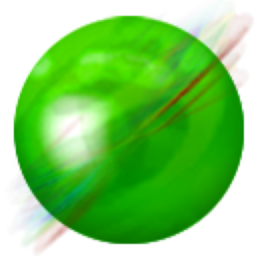
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
