1. Background
wget is a commonly used download tool in Linux systems, which can quickly download files, images and other resources. It has the characteristics of fast download speed, high reliability, and support for resumed downloads. Sometimes we need to use wget in other operating systems, but there is no similar tool.
In order to solve this problem, I tried to use golang to write a simple wget tool called golang-wget. It not only supports basic file downloads, but also supports breakpoint resume downloads, HTTP proxy and other functions.
This article will introduce the implementation principle, code structure and usage of golang-wget.
2. Implementation Principle
The implementation of golang-wget mainly relies on golang’s http package to implement HTTP protocol connection and file download.
The specific implementation principle is as follows:
- Get command line parameters
Get the url passed in from the command line, save path, and whether through golang's flag package Parameters such as the need to resume the download from a breakpoint and whether to use a proxy.
- Check whether breakpoint resumption is supported
If the user needs to perform breakpoint resumption, first check whether the target file exists, and if the file exists, obtain the file size. Use HTTP's Range request to get the starting position of the target file, which avoids downloading the entire file. If the file does not exist, the entire file is downloaded starting from 0.
- Configuring HTTP Client
Use golang's http package to create HTTP Client, and configure HTTP Transport according to whether the user needs to use a proxy. For specific configuration methods, please refer to golang official documentation.
- Download file
Use the Get, Head, Do and other methods of the http package to obtain the HTTP response, and use the Copy method in the io package to write the response body into the target file. . If you need to resume the transmission from a breakpoint, you need to locate the starting position before writing data.
3. Code Architecture
golang-wget is mainly divided into three parts: command line parameter analysis, HTTP client configuration, and file download.
Command line parameter parsing is implemented using the golang standard library flag, which can quickly parse command line parameters.
HTTP client configuration includes whether to use a proxy and HTTP Client configuration. Using a proxy requires more complex configuration.
File downloading includes checking whether breakpoint resumption is supported and using HTTP Client for file downloading.
The following is the framework of the main code:
func main() { // 解析命令行参数 flag.Parse() // 配置HTTP客户端 client := http.Client{ Transport: &http.Transport{ Proxy: http.ProxyFromEnvironment, TLSClientConfig: &tls.Config{InsecureSkipVerify: true}, }, } // 下载文件 download(&client, *url, *filePath, *isResume) } func download(client *http.Client, url, filePath string, isResume bool) error { // 检查是否支持断点续传 var startPosition int64 = 0 var err error if isResume { if isFileExists(filePath) { startPosition, err = getFileSize(filePath) } } // 创建HTTP请求 req, err := http.NewRequest("GET", url, nil) if err != nil { return err } if isResume { req.Header.Set("Range", fmt.Sprintf("bytes=%d-", startPosition)) } // 开始下载文件或续传文件 res, err := client.Do(req) if err != nil { return err } defer res.Body.Close() f, err := os.OpenFile(filePath, os.O_CREATE|os.O_WRONLY, os.ModePerm) if err != nil { return err } defer f.Close() // 如果需要续传,则先将位置定位到起始位置 if isResume { _, err = f.Seek(startPosition, io.SeekStart) if err != nil { return err } } _, err = io.Copy(f, res.Body) if err != nil { return err } return nil }
4. Usage method
Using golang-wget is very simple, just enter the corresponding command on the command line.
The command format is as follows:
golang-wget -url=URL -out=FILE_PATH [-resume=true|false] [-proxy=true|false] [-proxyAddr=PROXY_ADDR] [-debug=true|false]
Among them:
-url: Download URL address;
-out: Saved file path;
-resume: Whether to support breakpoint resume (optional, default false);
-proxy: whether to use a proxy (optional, default false);
-proxyAddr: proxy Address (optional, only needs to be set when -proxy=true);
-debug: whether to enable debugging mode (optional, default false).
For example, to download https://golang.org/doc/gopher/pkg.png and save it in the local /tmp/pkg.png file, you can use the following command:
golang-wget -url=https://golang.org/doc/gopher/pkg.png -out=/tmp/pkg.png
If you need to support breakpoint resumption, you can add the -resume=true parameter:
golang-wget -url=https://golang.org/doc/gopher/pkg.png -out=/tmp/pkg.png -resume=true
If you need to download from a proxy server, you can add the -proxy=true and -proxyAddr parameters:
golang-wget -url=https://golang.org/doc/gopher/pkg.png -out=/tmp/pkg.png -proxy=true -proxyAddr=http://proxyserver:port
5. Summary
Through the implementation of golang-wget, we can see that golang’s http package is very powerful and can easily implement HTTP protocol connections and file downloads. Through this example, we can learn how to use golang to implement our own download tool and implement some advanced functions, such as resumable downloads and proxy.
Writing your own download tools can not only increase your own technical implementation capabilities, but also reduce your dependence on other tools and improve efficiency.
The above is the detailed content of wget implemented by golang. For more information, please follow other related articles on the PHP Chinese website!
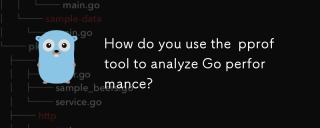
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
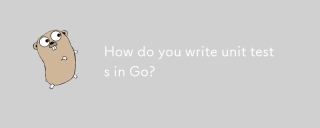
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
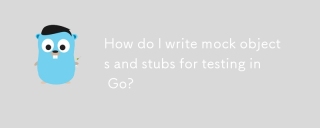
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
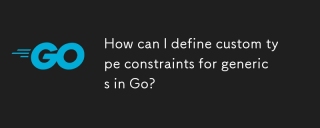
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
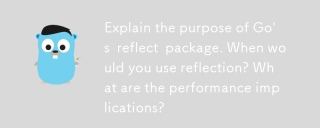
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
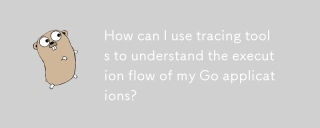
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
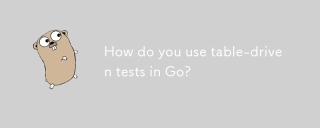
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
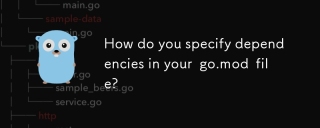
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
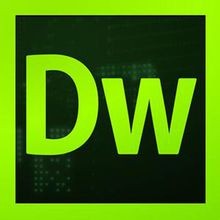
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
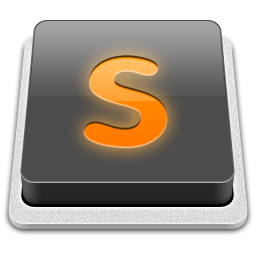
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
