


The System.currentTimeMillis() method provided in Java is used to obtain the current computer time. The expression format of the time is the current computer time and GMT time (Greenwich Mean Time) January 1, 1970 0:00:00 The difference in milliseconds.
The return type of the System.currentTimeMillis() method is long, which represents the current time in milliseconds.
During the development process, many people are usually accustomed to using new Date() to obtain the current time. What new Date() does is actually call the System.currentTimeMillis() method. If you only need the number of milliseconds, you can use System.currentTimeMillis() instead of new Date(), which will be more efficient.
[Example]Calculate the time-consuming situation of concatenating strings of String type and StringBuilder type.
/** * Java使用System.currentTimeMillis()方法计算程序运行时间 * @author pan_junbiao **/ public class CurrentTimeTest { /** * 使用String类型拼接字符串耗时 */ public static void testString() { String s = "Hello"; String s1 = "World"; long start = System.currentTimeMillis(); for(int i=0; i<10000; i++) { s+=s1; } long end = System.currentTimeMillis(); long runTime = (end - start); System.out.println("使用String类型拼接字符串耗时:" + runTime + "毫秒"); } /** * 使用StringBuilder类型拼接字符串耗时 */ public static void testStringBuilder() { StringBuilder s = new StringBuilder("Hello"); String s1 = "World"; long start = System.currentTimeMillis(); for(int i=0; i<10000; i++) { s.append(s1); } long end = System.currentTimeMillis(); long runTime = (end - start); System.out.println("使用StringBuilder类型拼接字符串耗时:" + runTime + "毫秒"); } public static void main(String[] args) { testString(); testStringBuilder(); } }
Running results:
Additional knowledge points:
From the picture above It can be seen from the running results that in the process of splicing strings, the StringBuilder object is used instead of the String object. This is because String is an immutable object, and a new String object is created every time the string is changed; while StringBuilder is a mutable character sequence, similar to String's string buffer. Therefore, using StringBuilder in places where strings are frequently modified will be more efficient than String.
The running speed in this regard is: StringBuilder > StringBuffer > String.
In terms of thread safety, StringBuilder is thread-unsafe, while StringBuffer is thread-safe.
The above is the detailed content of How to use System.currentTimeMillis() method to calculate program running time in Java. For more information, please follow other related articles on the PHP Chinese website!
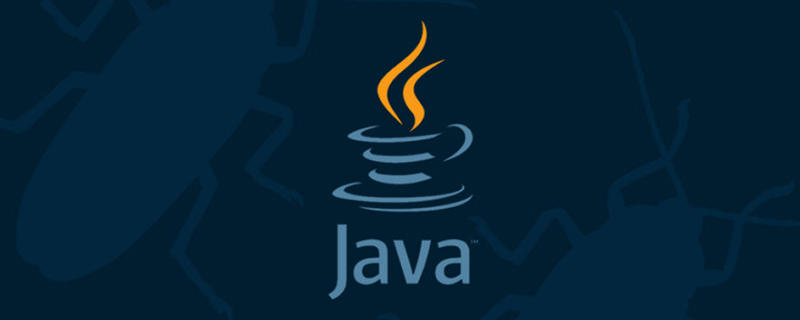
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
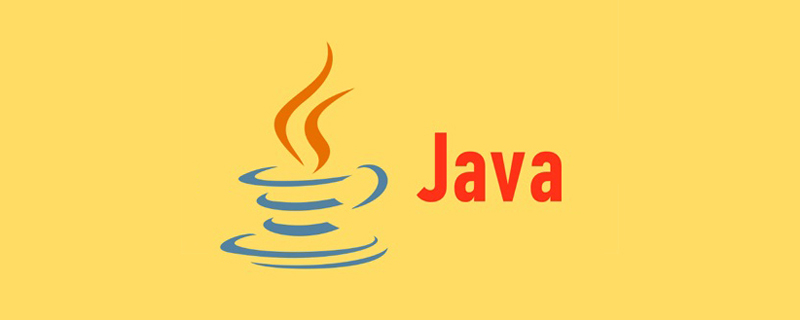
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
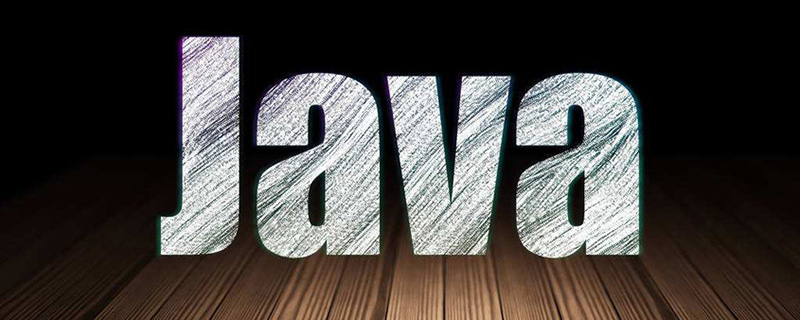
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
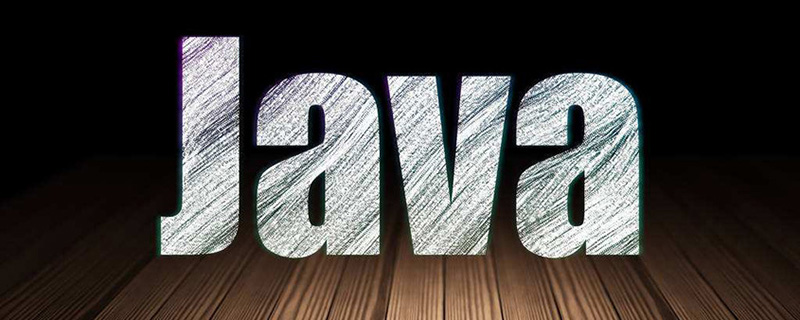
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
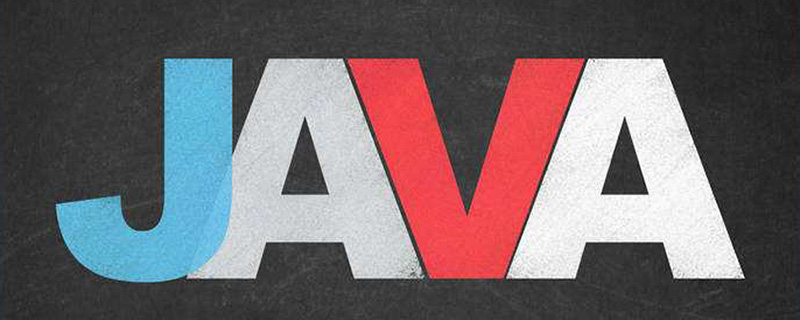
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
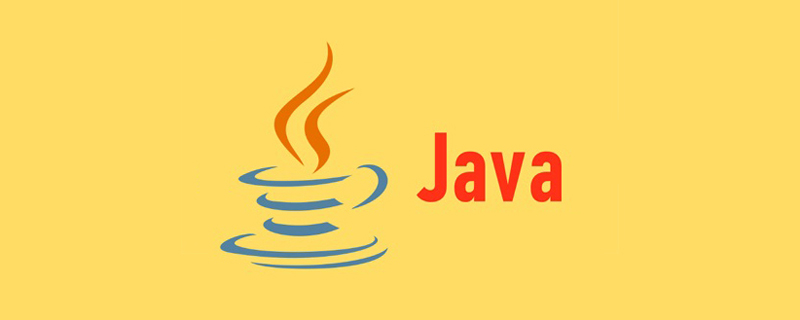
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
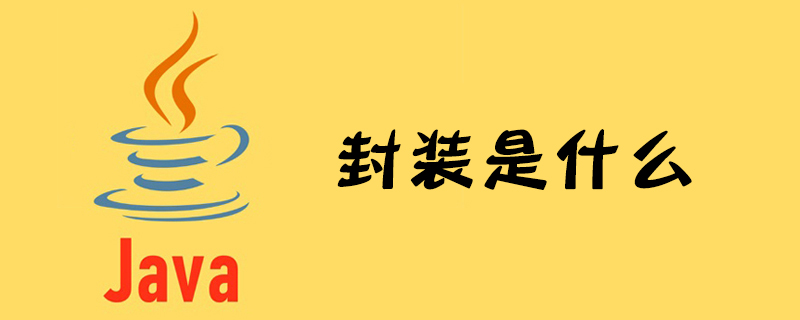
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
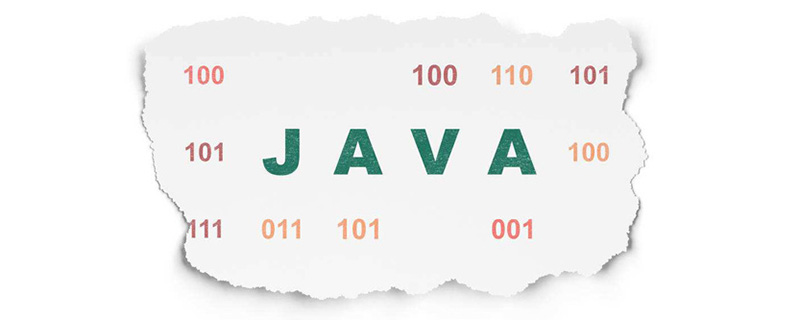
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于设计模式的相关问题,主要将装饰器模式的相关内容,指在不改变现有对象结构的情况下,动态地给该对象增加一些职责的模式,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
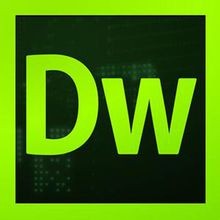
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment
