With the development of the Internet, data processing and management have become a vital part of various industries. A database management system (DBMS) is a software system for managing and accessing databases that many businesses use in daily operations and management. MySQL is one of the commonly used relational database management systems. During development, we may need to use programming language to connect and operate MySQL. This article will introduce how to use go language to query MySQL database.
1. Install the MySQL driver
The official website of go language provides the MySQL database driver and supports many different MySQL versions. Installing the MySQL driver requires that the system has a go language development environment and a MySQL database.
- Open the command line and use the following command to install the MySQL driver:
go get github.com/go-sql-driver/mysql
The go-sql-driver package provides basic functions for MySQL queries. You can now use it to perform MySQL queries.
2. Establish a database connection
Before using the go language to perform MySQL queries, you need to establish a connection to the MySQL database. To connect to the MySQL database, you need to specify the user name, password, database name, host address and other information.
- Open the go language editor (for example: VSCode) and create a new file named db.go.
- Enter the following code in the file:
package main import ( "database/sql" "fmt" "log" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "username:password@tcp(127.0.0.1:3306)/databaseName") if err != nil { log.Fatal(err) } defer db.Close() err = db.Ping() if err != nil { log.Fatal(err) } fmt.Println("Successful database connection") }
In the above code, we need to specify the driver name and database connection string when we use the sql.Open() function to open the MySQL database connection. The connection string specifies the username and password, as well as the MySQL host address and port number. When connecting to MySQL, the MySQL driver will be responsible for processing the connection response. If the connection to this database is successful, a pointer to the database object will be returned. After all matters are resolved, you need to use db.Close() to close the connection to ensure that there is no excessive consumption of database resources due to connection leaks.
3. Execute the query statement
After obtaining the SQL database connection, you can start executing the query and operation statements. The following is a command example of a simple query statement:
rows, err := db.Query("SELECT id, name, age FROM user")
The above query statement can query the id, name and age fields in the table named user. Using the db.Query() method will execute the query and return the row objects (rows) of the query results and possible errors (err). The result data of the query is wrapped in row objects (rows).
Of course, if the SQL statement we need to execute contains some parameters, we need to use placeholders. An example is as follows:
rows, err := db.Query("SELECT id, name, age FROM user WHERE name = ? AND age = ?", "John", 30)
The placeholder is automatically converted to the correct SQL format before querying, and the parameters required for the query are passed to the SQL driver. This approach prevents SQL injection attacks and keeps query statements safe.
4. Processing query results
After querying the MySQL database, we can use the Rows object to read data from the result set. The Rows object provides a set of methods for manipulating the result data of the query. For example, you can use the Scan() method to extract each column (column) in each row (row) in the result set:
for rows.Next() { var id int var name string var age int err := rows.Scan(&id, &name, &age) if err != nil { log.Fatal(err) } fmt.Printf("ID:%d, Name:%s, Age:%d", id, name, age) }
In the above code, use rows.Next() and rows.Scan( ) method extracts each row of data and outputs it to the console. When the rows.Next() method points to the next row, you can use the rows.Scan() method to extract the row data in that row. The parameter of the Scan() method is the address of each column of data.
5. Complete code example
The following is a complete example program that uses go language to query MySQL, which can be saved as a db.go file:
package main import ( "database/sql" "fmt" "log" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "username:password@tcp(127.0.0.1:3306)/databaseName") if err != nil { log.Fatal(err) } defer db.Close() err = db.Ping() if err != nil { log.Fatal(err) } fmt.Println("Successful database connection") rows, err := db.Query("SELECT id, name, age FROM user") if err != nil { log.Fatal(err) } defer rows.Close() for rows.Next() { var id int var name string var age int err := rows.Scan(&id, &name, &age) if err != nil { log.Fatal(err) } fmt.Printf("ID:%d, Name:%s, Age:%d ", id, name, age) } err = rows.Err() if err != nil { log.Fatal(err) } }
Summary
In this article, we learned how to query the MySQL database in go language. First you need to install the MySQL driver and then establish a connection to the MySQL database. After obtaining a database connection, you can execute queries and operation statements and use Rows objects to read data from the result set. Finally, we provide a complete Go language program example that demonstrates how to connect, query and read the MySQL database.
The above is the detailed content of How to query mysql in golang. For more information, please follow other related articles on the PHP Chinese website!
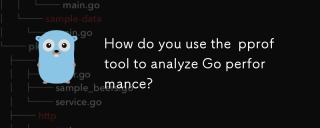
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
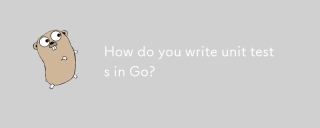
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
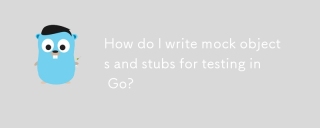
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
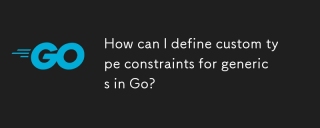
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
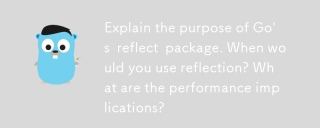
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
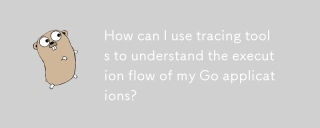
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
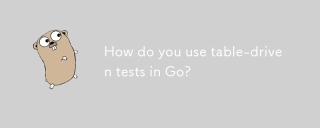
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
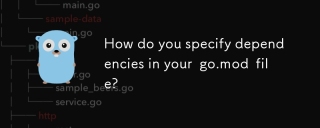
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
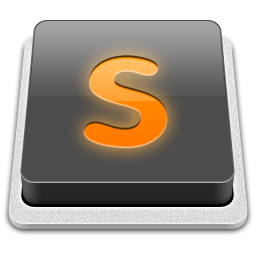
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
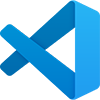
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
