C code to golang: a beginner’s attempt
In the field of computer science, C language has always been a very popular programming language and is widely used in embedded systems, operating systems, and networks. Programming and other fields. Golang is a programming language that has emerged in recent years and has attracted more and more people's attention and love. As a C language developer, I have also developed a strong interest in golang, and want to convert algorithms previously implemented in C language into golang code. This article will record my thoughts, experiences and shortcomings in this process.
First of all, we need to find a C language algorithm suitable for our transformation. What I chose is the quick sort algorithm, which is a classic sorting algorithm based on the idea of divide and conquer and has a time complexity of O(nlogn). I have written this algorithm in C language and tested it. The following is the code of this C language algorithm:
#include <stdio.h> void quick_sort(int arr[], int left, int right) { if (left < right) { int i = left, j = right, x = arr[left]; while (i < j) { while (i < j && arr[j] >= x) { j--; } if (i < j) { arr[i++] = arr[j]; } while (i < j && arr[i] < x) { i++; } if (i < j) { arr[j--] = arr[i]; } } arr[i] = x; quick_sort(arr, left, i - 1); quick_sort(arr, i + 1, right); } } int main() { int arr[10] = {3, 7, 2, 8, 1, 5, 10, 6, 4, 9}; quick_sort(arr, 0, 9); for (int i = 0; i < 10; i++) { printf("%d ", arr[i]); } return 0; }
Before converting it into golang code, I need to understand some basic features and grammatical rules of the golang language. Golang is a statically typed programming language and does not support implicit type conversion. In golang, the keyword for variable declaration is var
, and the keyword for function declaration is func
. In addition, golang's code structure is based on packages. Each code file should belong to a certain package, and other packages can be imported through the import
keyword. Now, let's see how I translate the quick sort algorithm into golang code.
package main import ( "fmt" ) func quick_sort(arr []int, left int, right int) { if left < right { i := left j := right x := arr[left] for i < j { for i < j && arr[j] >= x { j-- } if i < j { arr[i] = arr[j] i++ } for i < j && arr[i] < x { i++ } if i < j { arr[j] = arr[i] j-- } } arr[i] = x quick_sort(arr, left, i-1) quick_sort(arr, i+1, right) } } func main() { arr := []int{3, 7, 2, 8, 1, 5, 10, 6, 4, 9} quick_sort(arr, 0, len(arr)-1) fmt.Println(arr) }
As you can see, the process of converting a C language algorithm into golang code is not difficult. We only need to be proficient in the corresponding grammar rules. In this example, I changed all variable types in C language to the corresponding golang types. In addition, since golang does not support the traditional function parameter writing method in C language, I used a function parameter writing method similar to C. In this way, I converted traditional C language programs into golang programs.
Of course, you may encounter some problems during the conversion process. For example, in golang, there is no sizeof
operator in C language, so we need to use the len
function to get the length of the array. In addition, there is no ternary operator in C language in golang. We need to use if-else statements to complete conditional judgment. These problems may make our golang code more lengthy and complex than C language code, but it also provides us with more freedom of thinking and design, making our code clearer and easier to understand.
To summarize, it is not too difficult to convert C language code into golang code. By learning the grammatical rules and features of golang, we can easily convert existing C language algorithm code into golang code. The programming styles of the two languages are different, but both can help us write efficient and reliable programs. I hope this article can provide some help to readers who want to understand golang.
The above is the detailed content of C code to golang. For more information, please follow other related articles on the PHP Chinese website!
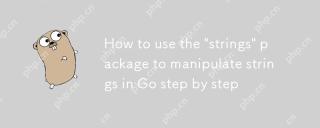
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
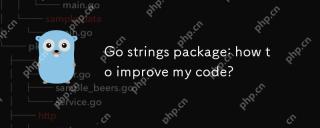
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
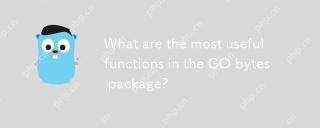
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
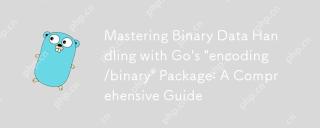
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
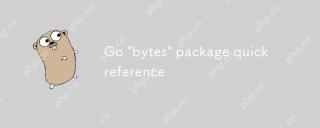
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
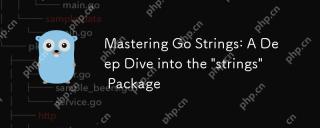
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
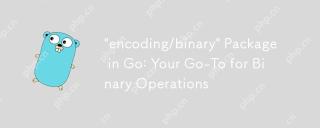
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
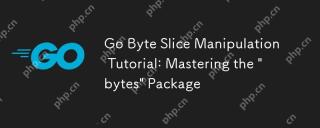
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
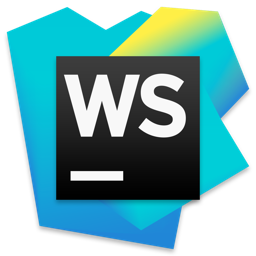
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
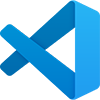
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
