In the microservice architecture, the gateway plays an important role, usually used for routing requests, load balancing and security verification. The proxy mode can provide better scalability and flexibility. This article will introduce how to use Golang to implement a simple gateway proxy.
- The role of gateway proxy
First, let us understand the role of gateway proxy. The gateway proxy can forward external requests to services in the internal microservice system, and can achieve the following functions:
1) Routing requests
The gateway proxy can, based on different request paths and parameters, Forward the request to the correct microservice instance.
2) Load balancing
When there are too many instances of a microservice system, the gateway proxy can achieve load balancing of requests to ensure that each microservice instance can get the request processed.
3) Security verification
The gateway proxy can perform security verification on the request, such as verifying the identity, signature, permissions, etc. of the request.
- Use Golang to implement a gateway proxy
Next, let us start using Golang to implement a simple gateway proxy.
2.1 Implement routing requests
First, we need to parse the request path and forward the request to the corresponding microservice instance according to different paths. For this we can use the routing functionality in Gin framework. The code is as follows:
router := gin.Default() // 转发请求给微服务 router.GET("/user/:id", func(c *gin.Context) { // 解析请求参数 id := c.Param("id") // 根据要请求的微服务实例,进行转发 req, err := http.NewRequest("GET", "http://user-service/"+id, nil) if err != nil { // 处理请求失败的情况 } resp, err := client.Do(req) if err != nil { // 处理请求失败的情况 } // 将响应返回给请求方 c.DataFromReader(resp.StatusCode, resp.ContentLength, resp.Header.Get("Content-Type"), resp.Body, nil) })
In the above code, we use the router.GET
method of the Gin framework to parse the request path, and use http.NewRequest
Method forwards the request to the correct microservice instance. It is assumed here that we have a microservice instance named user-service
and can correctly forward the request to it by parsing the request path.
2.2 Implementing load balancing
When there are too many microservice instances, a single gateway proxy may not be able to satisfy high-load requests. In order to solve this problem, we need to implement load balancing of requests. In Golang, we can use the load balancing plug-in in the Gin framework to implement the load balancing function. The code is as follows:
router := gin.Default() // 初始化负载均衡器 rr, err := roundrobin.NewBalancer( &roundrobin.Config{ Servers: []string{ "http://user-service-1:8080", "http://user-service-2:8080", "http://user-service-3:8080", }, Method: roundrobin.RoundRobin, }, ) if err != nil { // 处理初始化失败的情况 } // 转发请求给微服务 router.GET("/user/:id", func(c *gin.Context) { // 解析请求参数 id := c.Param("id") // 取得要请求的微服务实例 server, err := rr.GetServer() if err != nil { // 处理获取微服务实例失败的情况 } // 根据要请求的微服务实例,进行转发 url := fmt.Sprintf("%s/%s", server, id) req, err := http.NewRequest("GET", url, nil) if err != nil { // 处理请求失败的情况 } resp, err := client.Do(req) if err != nil { // 处理请求失败的情况 } // 将响应返回给请求方 c.DataFromReader(resp.StatusCode, resp.ContentLength, resp.Header.Get("Content-Type"), resp.Body, nil) })
In the above code, we use the roundrobin.NewBalancer
method to initialize a load balancer and specify the URLs of three microservice instances. When the gateway proxy receives the request, it will obtain a microservice instance from the load balancer and forward the request based on it.
2.3 Implement security verification
When the gateway proxy allows external system access, we need to perform security verification to prevent illegal requests and data leakage. In Golang, we can use JWT (JSON Web Tokens) as credentials for authentication and authorization. The code is as follows:
router := gin.Default() // 定义JWT密钥和超时时间 var jwtSecret = []byte("my_secret_key") var jwtTimeout = time.Hour * 24 // 1 day // 创建JWT验证中间件 authMiddleware := jwtmiddleware.New(jwtmiddleware.Options{ ValidationKeyGetter: func(token *jwt.Token) (interface{}, error) { return jwtSecret, nil }, SigningMethod: jwt.SigningMethodHS256, ErrorHandler: func(c *gin.Context, err error) { // 处理JWT验证错误的情况 }, }) // 转发请求给微服务 router.GET("/user/:id", authMiddleware.Handler(), func(c *gin.Context) { // 解析请求参数 id := c.Param("id") // 根据要请求的微服务实例,进行转发 req, err := http.NewRequest("GET", "http://user-service/"+id, nil) if err != nil { // 处理请求失败的情况 } resp, err := client.Do(req) if err != nil { // 处理请求失败的情况 } // 将响应返回给请求方 c.DataFromReader(resp.StatusCode, resp.ContentLength, resp.Header.Get("Content-Type"), resp.Body, nil) })
In the above code, we used the jwtmiddleware.New
method in the JWT framework to create a JWT verification middleware and specified the JWT key and timeout. . When the request reaches the gateway proxy, JWT verification will be performed first, and the request will be forwarded only after the verification is successful.
3. Summary
This article introduces how to use Golang to implement common functions of gateway proxy, including routing requests, load balancing, security verification, etc. These functions can help us better maintain and manage the microservice system. Of course, this is just an entry-level implementation, and the actual gateway proxy system may be more complex, and many practical issues need to be taken into consideration.
The above is the detailed content of Golang implements gateway proxy. For more information, please follow other related articles on the PHP Chinese website!
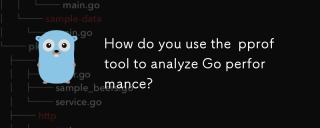
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
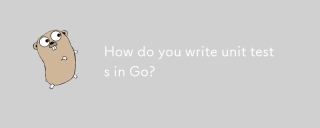
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
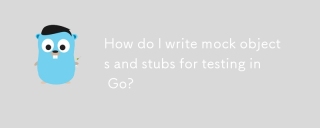
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
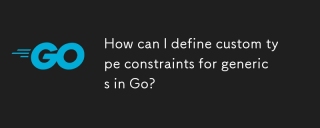
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
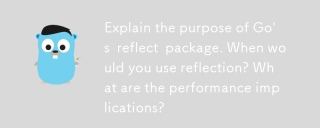
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
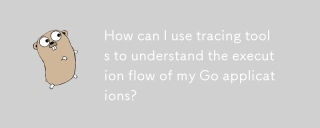
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
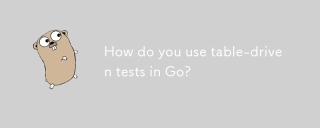
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
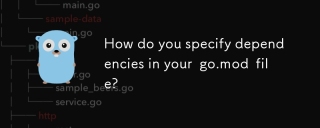
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
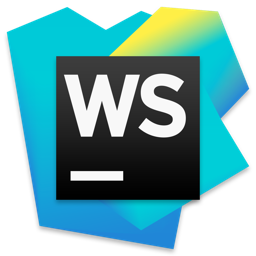
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
