With the development of the IT industry, Web applications are becoming more and more popular. Especially in recent years, with the rapid development of technologies such as mobile Internet and big data, the demand for Web applications has also increased.
In web applications, data query and display is one of the most important functions. In the case of large amounts of data, how to quickly and accurately query and display data has become a particularly important issue. Traditional paging can no longer meet the needs of users, because paging can only divide data into several pages for display, and users need to turn pages to view all the data.
In order to improve the user's query effect, we can use some new technologies to implement the query paging function. Among them, jQuery is a very popular web development tool with strong interactivity and ease of use, which can help us implement better web applications. This article will introduce how to use jQuery to implement query paging function.
1. The need for query paging
As the amount of data increases, the query results become larger and larger. If you want to display all the data on the page at once, it will cause problems for users in terms of fluency and data acquisition. For applications with large amounts of data, a common way is to solve this problem through paging.
The advantages of paging are:
- Improve the page opening speed and reduce the load on the server.
- The display is more orderly, making it easier for users to view and operate.
- Paging can accurately and quickly query the required data.
- Paging can make use of the browser's caching mechanism to relieve server pressure and reduce data transmission.
However, ordinary paging often makes the website boring, the waiting time for downloading is too long, and the user experience is not good. Therefore, the implementation of the query paging function is crucial, which can improve the user's query efficiency and user experience.
2. jQuery implements query paging
jQuery is a JavaScript-oriented library that is widely used to optimize and process JavaScript code. The method of using jQuery to implement query paging is relatively simple, and it is mainly divided into the following steps:
- Implement data query and store the query results in an array.
- Dynamicly generate query result page, paging control area and paging status bar.
- According to the user's query request, select the data node and dynamically create the paging node.
- According to the user's page turning request, reload the paging data and dynamically update the page.
Below, we will introduce step by step how to use jQuery to implement the query paging function:
- Implement data query
First, we need Storing data into an array is very easy for web applications written in JavaScript. Just call JSON's parse() method to convert a JSON-formatted string into a JavaScript object.
The following is an example:
let jsonData='[{"name":"张三","age":20,"salary":"5000"},{"name":"李四","age":22,"salary":"6000"},{"name":"王五","age":25,"salary":"8000"},{"name":"赵六","age":27,"salary":"9000"},{"name":"钱七","age":30,"salary":"10000"},{"name":"二哈","age":32,"salary":"12000"},{"name":"三哈","age":35,"salary":"15000"},{"name":"四哈","age":37,"salary":"18000"},{"name":"五哈","age":40,"salary":"20000"},{"name":"六哈","age":42,"salary":"22000"}]'; let data=JSON.parse(jsonData);
- Dynamic generation of page elements
Dynamic generation of page elements uses the DOM operations in jQuery, mainly through The append() and html() methods in jQuery are implemented. You can use the append() method to add new nodes and child nodes, and you can also use the html() method to change the innerHTML of the node.
The following is an example:
//动态添加查询结果区域 let $table=$('<table><thead><tr><th>姓名</th><th>年龄</th><th>薪资</th></tr></thead><tbody></tbody></table>'); $container.append($table); for(let item of data){ let $tr=$('<tr><td>'+item.name+'</td><td>'+item.age+'</td><td>'+item.salary+'</td></tr>'); $table.find('tbody').append($tr); } //动态添加分页控制区域 let $pagerBox=$('<div class="pager-box"></div>'); let $prePage=$('<a href="javascript:void(0);" class="pre-page">上一页</a>'); let $nextPage=$('<a href="javascript:void(0);" class="next-page">下一页</a>'); $pagerBox.append($prePage); for(let i=1;i<=data.length;i++){ let $pageNode=$('<a href="javascript:void(0);" class="pager-num">'+i+'</a>'); $pagerBox.append($pageNode); } $pagerBox.append($nextPage); $container.append($pagerBox); //动态添加分页状态条 let $pageStatus=$('<div class="page-status"><span class="current-page">1</span>/<span class="total-page">'+Math.ceil(data.length/pageSize)+'页</span></div>'); $container.append($pageStatus); //定义页面元素的CSS样式 $container.css({'text-align':'center','background-color':'#f7f7f7'}); $table.css({'width':'90%','margin':'0 auto','background-color':'#fff','border':'1px solid #ddd','border-radius':'5px'}); $table.find('thead th').css({'padding-top':'10px','padding-bottom':'10px','background-color':'#eee'}); $pagerBox.css({'width':'90%','margin':'20px auto','height':'30px','line-height':'30px'}); $pagerBox.find('.pager-num').css({'width':'30px','text-align':'center','background-color':'#fff','border':'1px solid #ddd','border-radius':'3px','margin':'0 5px'}); $pagerBox.find('.pager-num.active').css({'background-color':'#e4393c','color':'#fff'}); $pagerBox.find('.pre-page,.next-page').css({'display':'inline-block','color':'#333','text-decoration':'none','margin':'0 5px'}); $pageStatus.css({'width':'90%','margin':'10px auto','color':'#999','font-size':'14px'});
- Creating paging nodes
Through jQuery, paging nodes can be dynamically created. The click() method is used here. Monitor the user's page turning operations.
Here is an example:
let $pagerNodes=$('.pager-num'); $pagerNodes.eq(0).addClass('active'); $pagerNodes.click(function(){ let index=$(this).index(); let start=(index-1)*pageSize; let end=index*pageSize; let dataArr=data.slice(start,end); $table.find('tbody').html(''); for(let item of dataArr){ let $tr=$('<tr><td>'+item.name+'</td><td>'+item.age+'</td><td>'+item.salary+'</td></tr>'); $table.find('tbody').append($tr); } $pagerNodes.removeClass('active'); $(this).addClass('active'); $pageStatus.find('.current-page').text(index); });
- Update page
The last step is to update the page data. We need to reload the paging data according to the user's page turning request and dynamically update the page. The addClass() and removeClass() methods are used here to highlight the current page.
The following is an example:
let currentPage=1; $prePage.click(function(){ if(currentPage>1){ currentPage--; let index=currentPage-1; let start=index*pageSize; let end=(index+1)*pageSize; let dataArr=data.slice(start,end); $table.find('tbody').html(''); for(let item of dataArr){ let $tr=$('<tr><td>'+item.name+'</td><td>'+item.age+'</td><td>'+item.salary+'</td></tr>'); $table.find('tbody').append($tr); } $pagerNodes.removeClass('active'); $pagerNodes.eq(currentPage-1).addClass('active'); $pageStatus.find('.current-page').text(currentPage); } }); $nextPage.click(function(){ if(currentPage<Math.ceil(data.length/pageSize)){ currentPage++; let index=currentPage-1; let start=index*pageSize; let end=(index+1)*pageSize; let dataArr=data.slice(start,end); $table.find('tbody').html(''); for(let item of dataArr){ let $tr=$('<tr><td>'+item.name+'</td><td>'+item.age+'</td><td>'+item.salary+'</td></tr>'); $table.find('tbody').append($tr); } $pagerNodes.removeClass('active'); $pagerNodes.eq(currentPage-1).addClass('active'); $pageStatus.find('.current-page').text(currentPage); } });
3. Summary
Query paging is one of the common functions of Web applications, and it is very important when processing large amounts of data. jQuery is a very popular web development tool that can help us easily implement query paging functions. Through the introduction of this article, we have learned how to use jQuery to implement the query paging function, which involves operations such as data query, dynamic generation of page elements, creation of paging nodes, and dynamic page updating.
Using jQuery to implement query paging not only improves the interactivity and ease of use of web applications, but also provides us with more design space. In practical applications, the implementation of the query paging function also needs to be adjusted and optimized according to actual needs. Hope this article is helpful to readers.
The above is the detailed content of jquery implements query paging. For more information, please follow other related articles on the PHP Chinese website!

No,youshouldn'tusemultipleIDsinthesameDOM.1)IDsmustbeuniqueperHTMLspecification,andusingduplicatescancauseinconsistentbrowserbehavior.2)Useclassesforstylingmultipleelements,attributeselectorsfortargetingbyattributes,anddescendantselectorsforstructure

HTML5aimstoenhancewebcapabilities,makingitmoredynamic,interactive,andaccessible.1)Itsupportsmultimediaelementslikeand,eliminatingtheneedforplugins.2)Semanticelementsimproveaccessibilityandcodereadability.3)Featureslikeenablepowerful,responsivewebappl

HTML5aimstoenhancewebdevelopmentanduserexperiencethroughsemanticstructure,multimediaintegration,andperformanceimprovements.1)Semanticelementslike,,,andimprovereadabilityandaccessibility.2)andtagsallowseamlessmultimediaembeddingwithoutplugins.3)Featur

HTML5isnotinherentlyinsecure,butitsfeaturescanleadtosecurityrisksifmisusedorimproperlyimplemented.1)Usethesandboxattributeiniframestocontrolembeddedcontentandpreventvulnerabilitieslikeclickjacking.2)AvoidstoringsensitivedatainWebStorageduetoitsaccess

HTML5aimedtoenhancewebdevelopmentbyintroducingsemanticelements,nativemultimediasupport,improvedformelements,andofflinecapabilities,contrastingwiththelimitationsofHTML4andXHTML.1)Itintroducedsemantictagslike,,,improvingstructureandSEO.2)Nativeaudioand

Using ID selectors is not inherently bad in CSS, but should be used with caution. 1) ID selector is suitable for unique elements or JavaScript hooks. 2) For general styles, class selectors should be used as they are more flexible and maintainable. By balancing the use of ID and class, a more robust and efficient CSS architecture can be implemented.

HTML5'sgoalsin2024focusonrefinementandoptimization,notnewfeatures.1)Enhanceperformanceandefficiencythroughoptimizedrendering.2)Improveaccessibilitywithrefinedattributesandelements.3)Addresssecurityconcerns,particularlyXSS,withwiderCSPadoption.4)Ensur

HTML5aimedtoimprovewebdevelopmentinfourkeyareas:1)Multimediasupport,2)Semanticstructure,3)Formcapabilities,and4)Offlineandstorageoptions.1)HTML5introducedandelements,simplifyingmediaembeddingandenhancinguserexperience.2)Newsemanticelementslikeandimpr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
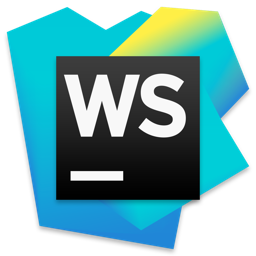
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
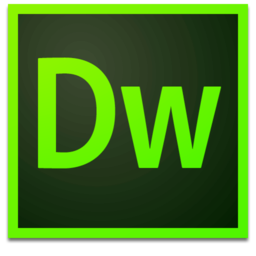
Dreamweaver Mac version
Visual web development tools
