Recently, PHP8.0 released a new mail library, making it easier to send and receive emails in PHP. This library has powerful features including building emails, sending emails, parsing emails, getting attachments and solving email getting stuck issues.
In many projects, we need to use email for communication and some necessary business operations. The mail library in PHP8.0 allows us to achieve this easily. Next, we'll explore this new mail library and see how to use it in our application.
Install the mail library
First, we need to use Composer to install the PHP8.0 mail library. In our project directory, we can run the following command:
composer require phpmailer/phpmailer
This command will install the PHPMailer library, which is the standard mail library for PHP8.0.
Establishing a connection
Before we use the mail library, we need to establish a connection with the SMTP server. We can use SMTP protocol to send emails. The SMTP server requires an SMTP address and port. Using the mail library in PHP8.0, we can use the following code to establish a connection with the SMTP server:
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require 'vendor/autoload.php ';
$mail = new PHPMailer(true);
try {
//Server settings $mail->SMTPDebug = SMTP::DEBUG_SERVER; //Enable verbose debug output $mail->isSMTP(); //Send using SMTP $mail->Host = 'smtp.gmail.com'; //Set the SMTP server to send through $mail->SMTPAuth = true; //Enable SMTP authentication $mail->Username = 'yourname@gmail.com'; //SMTP username $mail->Password = 'yourpassword'; //SMTP password $mail->SMTPSecure = PHPMailer::ENCRYPTION_SMTPS; //Enable implicit TLS encryption $mail->Port = 465; //TCP port to connect to; use 587 if you have no SSL/TLS support //Recipients $mail->setFrom('yourname@gmail.com', 'Your Name'); $mail->addAddress('recipient@example.com', 'Recipient Name'); //Add a recipient //Content $mail->isHTML(true); //Set email format to HTML $mail->Subject = 'Test Email'; $mail->Body = 'This is a test email.'; $mail->AltBody = 'This is the body in plain text for non-HTML mail clients'; $mail->send(); echo 'Message has been sent';
} catch (Exception $e) {
echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}";
}
In the above code, we first introduced the PHPMailer library and created a PHPMailer instance. We then set up the SMTP server's address, port number and username, password, and enabled SMTP authentication. We also format and content the email and specify the sender and recipient addresses.
Using the mail library
After setting up the connection with the SMTP server, we can use the mail library of PHP8.0 to send emails. We can use the following code to send an email:
//Content
$mail->isHTML(true); //Set email format to HTML
$mail->Subject = 'Test Email';
$mail->Body = 'This is a test email.';
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients' ;
$mail->send();
The isHTML() method in the above code is used to specify that the content of the email sent is in HTML format. The Subject attribute specifies the subject of the email, the Body attribute specifies the content of the email, and the AltBody attribute specifies the plain text content of the email so that it cannot be viewed using an HTML-formatted email client.
Parsing emails
The mail library of PHP8.0 also provides the function of parsing emails. We can use the following code to parse an email:
// Load the email message
$mail = new PHPMailer();
$mail->setServer('smtp.gmail. com', 'username', 'password');
$mail->setPort('587');
$mail->addAddress('recipient@example.com', 'John Doe') ;
// Retrieve the whole email content
$mail->retrieve();
// Convert the email body to a UTF-8 string
$emailBody = $mail->utf8ize($mail->Body);
// Parse the email using PHP's built-in imap functions
$imapStream = imap_open('{imap.gmail.com:993 /imap/ssl}INBOX', 'username', 'password');
$message = imap_fetchbody($imapStream, 1, "");
// Parse the email body using PHP's built- in DOM functions
$dom = new DOMDocument();
@$dom->loadHTML($emailBody);
$data = array();
$header = $dom-> getElementsByTagName('header')->item(0);
foreach($header->childNodes as $node) {
if ($node->nodeName == 'from') { list($name, $email) = explode(' <', $node->nodeValue); $data['from_name'] = $name; $data['from_email'] = str_replace('>', '', $email); } elseif ($node->nodeName == 'date') { $data['date'] = date('Y-m-d H:i:s', strtotime($node->nodeValue)); } elseif ($node->nodeName == 'subject') { $data['subject'] = $node->nodeValue; }
}
retrieve( in the above code ) method is used to retrieve the contents of the entire email. After converting the email content into UTF-8 format, we can use PHP's built-in imap function to parse the email. We can also use PHP's DOM functions to parse email header information.
Summary
PHP8.0’s mail library makes it easier to use email in PHP applications. The library provides powerful functionality including building emails, sending emails, parsing emails and getting attachments of emails. By using PHPMailer library we can easily implement mail functionality and use it in our application.
The above is the detailed content of Mail library in PHP8.0. For more information, please follow other related articles on the PHP Chinese website!
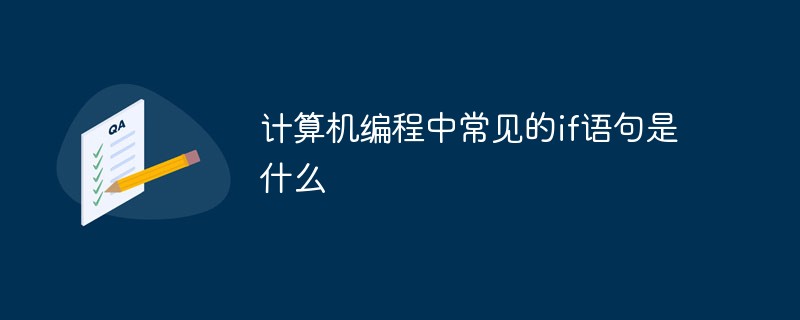
计算机编程中常见的if语句是条件判断语句。if语句是一种选择分支结构,它是依据明确的条件选择选择执行路径,而不是严格按照顺序执行,在编程实际运用中要根据程序流程选择适合的分支语句,它是依照条件的结果改变执行的程序;if语句的简单语法“if(条件表达式){// 要执行的代码;}”。
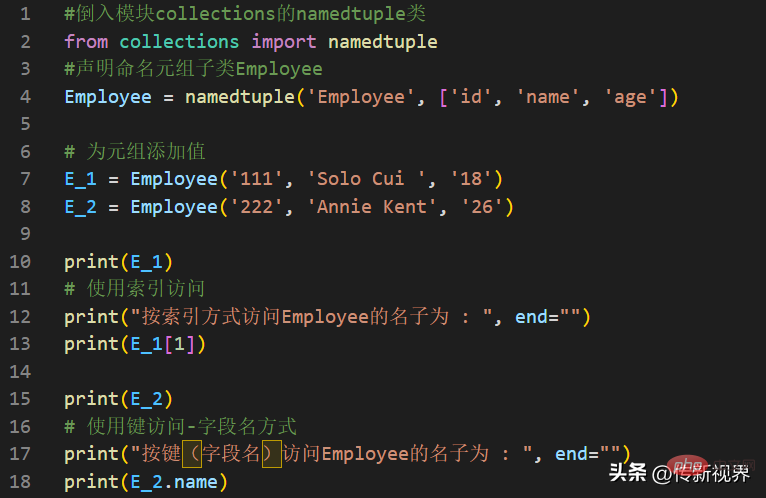
前言本文继续来介绍Python集合模块,这次主要简明扼要的介绍其内的命名元组,即namedtuple的使用。闲话少叙,我们开始——记得点赞、关注和转发哦~ ^_^创建命名元组Python集合中的命名元组类namedTuples为元组中的每个位置赋予意义,并增强代码的可读性和描述性。它们可以在任何使用常规元组的地方使用,且增加了通过名称而不是位置索引方式访问字段的能力。其来自Python内置模块collections。其使用的常规语法方式为:import collections XxNamedT
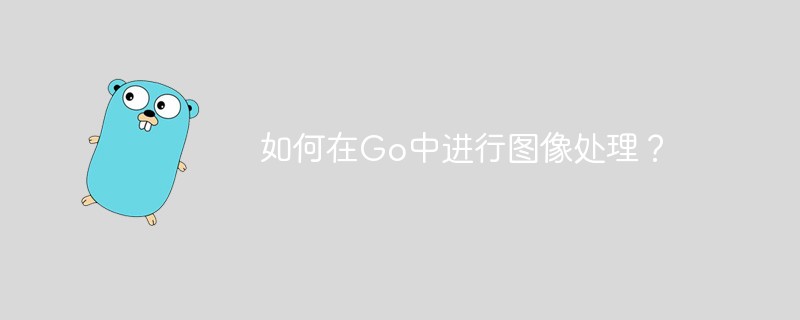
作为一门高效的编程语言,Go在图像处理领域也有着不错的表现。虽然Go本身的标准库中没有提供专门的图像处理相关的API,但是有一些优秀的第三方库可以供我们使用,比如GoCV、ImageMagick和GraphicsMagick等。本文将重点介绍使用GoCV进行图像处理的方法。GoCV是一个高度依赖于OpenCV的Go语言绑定库,其
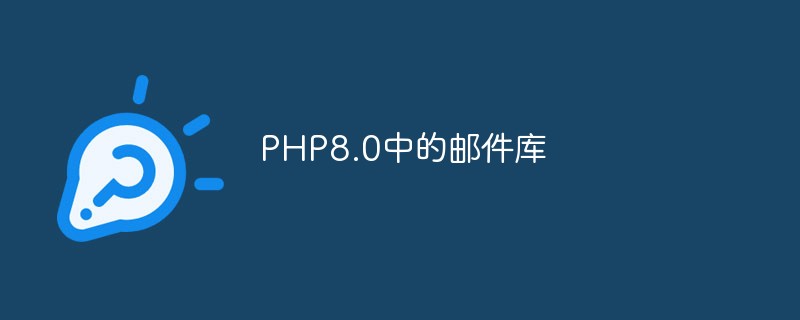
最近,PHP8.0发布了一个新的邮件库,使得在PHP中发送和接收电子邮件变得更加容易。这个库具有强大的功能,包括构建电子邮件,发送电子邮件,解析电子邮件,获取附件和解决电子邮件获得卡住的问题。在很多项目中,我们都需要使用电子邮件来进行通信和一些必备的业务操作。而PHP8.0中的邮件库可以让我们轻松地实现这一点。接下来,我们将探索这个新的邮件库,并了解如何在我
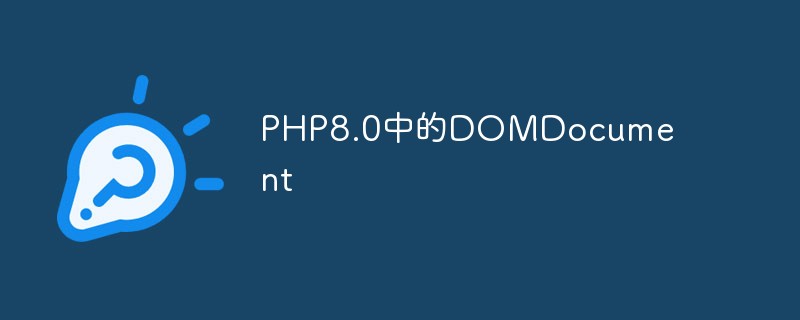
随着PHP8.0的发布,DOMDocument作为PHP内置的XML解析库,也有了新的变化和增强。DOMDocument在PHP中的重要性不言而喻,尤其在处理XML文档方面,它的功能十分强大,而且使用起来也十分简单。本文将介绍PHP8.0中DOMDocument的新特性和应用。一、DOMDocument概述DOM(DocumentObjectModel)
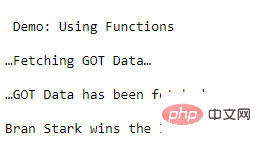
Python 中的 main 函数充当程序的执行点,在 Python 编程中定义 main 函数是启动程序执行的必要条件,不过它仅在程序直接运行时才执行,而在作为模块导入时不会执行。要了解有关 Python main 函数的更多信息,我们将从如下几点逐步学习:什么是 Python 函数Python 中 main 函数的功能是什么一个基本的 Python main() 是怎样的Python 执行模式Let’s get started什么是 Python 函数相信很多小伙伴对函数都不陌生了,函数是可
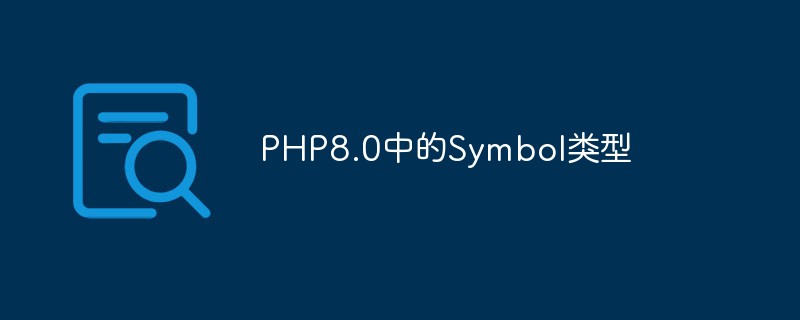
PHP8.0是PHP语言的最新版本,自发布以来已经引发了广泛的关注和争议。其中,最引人瞩目的新特性之一就是Symbol类型。Symbol类型是PHP8.0中新增的一种数据类型,它类似于JavaScript中的Symbol类型,可用于表示独一无二的值。这意味着,两个Symbol类型的值即使完全相同,它们也是不相等的。Symbol类型的使用可以避免在不同的代码段
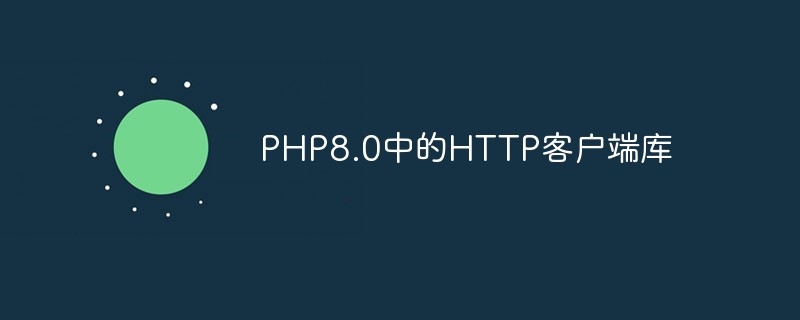
PHP8.0中的HTTP客户端库PHP8.0的发布带来了很多新特性和改进,其中一个最引人注目的是内置的HTTP客户端库的加入。这个库提供了一个简单的方法来发送HTTP请求并处理返回的响应。在本文中,我们将探讨这个库的主要功能和用法。发送HTTP请求使用PHP8.0内置的HTTP客户端库发送HTTP请求非常简单。在本例中,我们将使用GET方法获取这个网站的首页


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
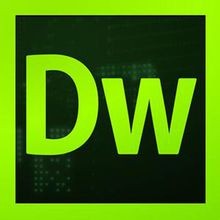
Dreamweaver CS6
Visual web development tools
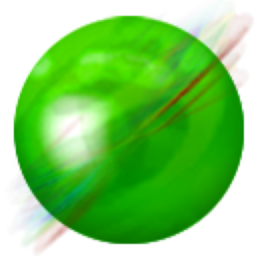
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
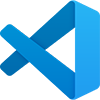
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
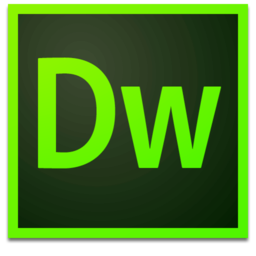
Dreamweaver Mac version
Visual web development tools
