Node.js realizes the drift bottle
The drift bottle is a traditional and romantic thing that can drift with the current and drift far away. In the digital age, we can simulate this kind of toys through technical means. This article will introduce how to use Node.js to implement a simple drift bottle application.
Step 1: Build a basic environment
First, we need to ensure that Node.js is installed on the computer. After the installation is complete, enter the following command on the command line to check whether Node.js is installed successfully:
node -v
If the installation is successful, output the version number.
Next, we need to install some necessary dependent libraries, including the Express framework and MongoDB database. Use the following command on the command line to install:
npm install express mongodb --save
The npm command is used here to install the dependent library, where the --save
parameter indicates that the name and version number of the dependent library will be recorded in the project package.json
file to facilitate team collaboration and code maintenance.
Step 2: Implement background logic
Next, we need to write some background logic, including routing and data operations. Create a new app.js
file in the root directory of the project, and enter the following content in it:
const express = require('express'); const mongodb = require('mongodb').MongoClient; const app = express(); const port = process.env.PORT || 3000; const dbUrl = 'mongodb://localhost:27017/bottle'; app.get('/', function(req, res) { res.send('Hello, world!'); }); app.listen(port, function() { console.log(`Server is listening on port ${port}`); });
This code implements a basic Express application, listening on port 3000. Also connect to the MongoDB database named bottle
. A root route /
is also defined, returning Hello, world!
.
Next, we need to implement three routes:
-
/throw
: used to drop drift bottles. -
/pick
: Used to find drift bottles. -
/delete/:id
: used to delete drift bottles.
Let’s first look at how to implement the first route. Add the following code to the app.js
file:
app.get('/throw', function(req, res) { const user = req.query.user; const content = req.query.content; const bottle = {user: user, content: content}; mongodb.connect(dbUrl, function(err, client) { if (err) { return console.log('Failed to connect to database'); } const db = client.db('bottle'); db.collection('bottles').insertOne(bottle, function(err, result) { if (err) { return console.log('Failed to insert bottle'); } res.send('Throw bottle success'); client.close(); }); }); });
This code is used to drop the drift bottle. It first obtains the user name and content in the query parameter, and then assembles it into a JSON object. Then connect to the MongoDB database and insert the object in the bottles
collection. If the insertion is successful, Throw bottle success
is returned.
Next, we need to implement the second route. Add the following code to the app.js
file:
app.get('/pick', function(req, res) { const user = req.query.user; mongodb.connect(dbUrl, function(err, client) { if (err) { return console.log('Failed to connect to database'); } const db = client.db('bottle'); db.collection('bottles').findOne({user: user}, function(err, result) { if (err) { return console.log('Failed to find bottle'); } if (!result) { return res.send('No bottle found'); } const bottle = {user: result.user, content: result.content}; db.collection('bottles').deleteOne({_id: result._id}, function(err, result) { if (err) { return console.log('Failed to delete bottle'); } res.send(bottle); client.close(); }); }); }); });
This code is used to find drift bottles. First, get the username in the query parameter, then connect to the MongoDB database, and find the drifting bottles that match the username in the bottles
collection. If a drift bottle is found, delete it from the database and return the result to the client.
Finally, we need to implement the third route. Add the following code to the app.js
file:
app.get('/delete/:id', function(req, res) { const id = req.params.id; mongodb.connect(dbUrl, function(err, client) { if (err) { return console.log('Failed to connect to database'); } const db = client.db('bottle'); db.collection('bottles').deleteOne({_id: mongodb.ObjectID(id)}, function(err, result) { if (err) { return console.log('Failed to delete bottle'); } res.send('Delete bottle success'); client.close(); }); }); });
This code is used to delete the drift bottle. First get the drift bottle ID in the routing parameters, then connect to the MongoDB database, and delete the drift bottle in the bottles
collection. If the deletion is successful, Delete bottle success
is returned.
Step 3: Implement front-end interaction
Now, we have implemented a complete background logic. Next, we need to implement some front-end interactions. Create a new public
folder in the root directory of the project, create a index.html
file in it, and then enter the following code:
<!DOCTYPE html> <html> <head> <title>Drifting Bottle</title> <meta charset="utf-8"> </head> <body> <form id="throwForm"> <input type="text" name="user" placeholder="Your name"><br> <textarea name="content" placeholder="Message"></textarea><br> <input type="submit" value="Throw"> </form> <hr> <form id="pickForm"> <input type="text" name="user" placeholder="Your name"><br> <input type="submit" value="Pick"> </form> <hr> <ul id="bottleList"></ul> <script src="https://code.jquery.com/jquery-3.6.0.slim.min.js"></script> <script> $(function() { $('#throwForm').submit(function(event) { event.preventDefault(); const form = $(this); $.get('/throw', form.serialize(), function(data) { alert(data); }); }); $('#pickForm').submit(function(event) { event.preventDefault(); const form = $(this); $.get('/pick', form.serialize(), function(data) { if (typeof(data) === 'string') { alert(data); } else { $('#bottleList').append(`<li>${data.user}: ${data.content}</li>`); } }); }); $('#bottleList').on('click', 'li', function() { if (confirm('Are you sure to delete this bottle?')) { const id = $(this).attr('data-id'); $.get(`/delete/${id}`, function(data) { alert(data); $(this).remove(); }.bind(this)); } }); }); </script> </body> </html>
This code implements A simple user interface consists of two forms and a list. Among them, the form is used to submit drift bottles and find drift bottles, and the list is used to display the found drift bottles.
Next, add the following code in the app.js
file to set the static file directory to public
:
app.use(express.static('public'));
Finally, in the command Enter the following command in the line to start the application:
node app.js
Visit http://localhost:3000
to use the drift bottle application.
Summary
This article introduces how to use Node.js to implement a drift bottle application. By implementing back-end logic and front-end interaction, we established a basically usable drifting bottle system. Of course, this is just a very simple drift bottle application, and there are many areas that need to be improved and optimized, such as data verification, exception handling, security, etc. But this application is enough to demonstrate the powerful capabilities and elegant programming methods of Node.js in web applications. I hope this article can be helpful to readers who want to learn Node.js.
The above is the detailed content of Nodejs implements drift bottle. For more information, please follow other related articles on the PHP Chinese website!

React is a JavaScript library developed by Meta for building user interfaces, with its core being component development and virtual DOM technology. 1. Component and state management: React manages state through components (functions or classes) and Hooks (such as useState), improving code reusability and maintenance. 2. Virtual DOM and performance optimization: Through virtual DOM, React efficiently updates the real DOM to improve performance. 3. Life cycle and Hooks: Hooks (such as useEffect) allow function components to manage life cycles and perform side-effect operations. 4. Usage example: From basic HelloWorld components to advanced global state management (useContext and

The React ecosystem includes state management libraries (such as Redux), routing libraries (such as ReactRouter), UI component libraries (such as Material-UI), testing tools (such as Jest), and building tools (such as Webpack). These tools work together to help developers develop and maintain applications efficiently, improve code quality and development efficiency.

React is a JavaScript library developed by Facebook for building user interfaces. 1. It adopts componentized and virtual DOM technology to improve the efficiency and performance of UI development. 2. The core concepts of React include componentization, state management (such as useState and useEffect) and the working principle of virtual DOM. 3. In practical applications, React supports from basic component rendering to advanced asynchronous data processing. 4. Common errors such as forgetting to add key attributes or incorrect status updates can be debugged through ReactDevTools and logs. 5. Performance optimization and best practices include using React.memo, code segmentation and keeping code readable and maintaining dependability

The application of React in HTML improves the efficiency and flexibility of web development through componentization and virtual DOM. 1) React componentization idea breaks down the UI into reusable units to simplify management. 2) Virtual DOM optimization performance, minimize DOM operations through diffing algorithm. 3) JSX syntax allows writing HTML in JavaScript to improve development efficiency. 4) Use the useState hook to manage state and realize dynamic content updates. 5) Optimization strategies include using React.memo and useCallback to reduce unnecessary rendering.

React's main functions include componentized thinking, state management and virtual DOM. 1) The idea of componentization allows splitting the UI into reusable parts to improve code readability and maintainability. 2) State management manages dynamic data through state and props, and changes trigger UI updates. 3) Virtual DOM optimization performance, update the UI through the calculation of the minimum operation of DOM replica in memory.

The advantages of React are its flexibility and efficiency, which are reflected in: 1) Component-based design improves code reusability; 2) Virtual DOM technology optimizes performance, especially when handling large amounts of data updates; 3) The rich ecosystem provides a large number of third-party libraries and tools. By understanding how React works and uses examples, you can master its core concepts and best practices to build an efficient, maintainable user interface.

React is a JavaScript library for building user interfaces, suitable for large and complex applications. 1. The core of React is componentization and virtual DOM, which improves UI rendering performance. 2. Compared with Vue, React is more flexible but has a steep learning curve, which is suitable for large projects. 3. Compared with Angular, React is lighter, dependent on the community ecology, and suitable for projects that require flexibility.

React operates in HTML via virtual DOM. 1) React uses JSX syntax to write HTML-like structures. 2) Virtual DOM management UI update, efficient rendering through Diffing algorithm. 3) Use ReactDOM.render() to render the component to the real DOM. 4) Optimization and best practices include using React.memo and component splitting to improve performance and maintainability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
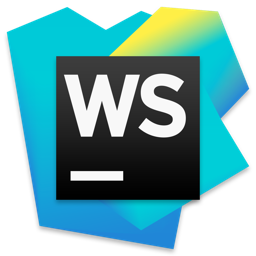
WebStorm Mac version
Useful JavaScript development tools
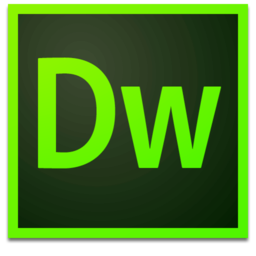
Dreamweaver Mac version
Visual web development tools
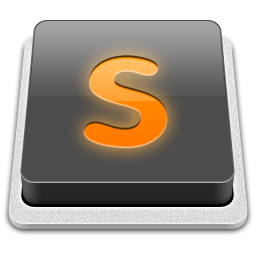
SublimeText3 Mac version
God-level code editing software (SublimeText3)