How to use Set interface in Java to store arrays without duplicate elements
Set interface
The Set interface, like the List interface, inherits the Collection interface. The elements in the Set interface are unordered, and certain rules are used to ensure that the stored elements are not repeated.
HashSet collection
HashSet is an implementation class of the Set interface. The stored elements are non-repeatable, and the elements are unordered. When an object is added to the HashSet collection, First, the hashCode() method of the object is called to calculate the hash value of the object to determine the storage location of the element. If the hash values are the same at this time, call the equals() method of the object to ensure that there are no duplicate elements at this position.
package 集合类; import java.util.HashSet; import java.util.Iterator; public class Set { public static void main(String[] args) { HashSet set=new HashSet(); set.add("hello"); set.add("world"); set.add("abc"); set.add("hello"); Iterator it=set.iterator(); while(it.hasNext()){ Object obj=it.next(); System.out.print(obj+ " "); } } }
Running results
package 集合类; import java.util.HashSet; class Student{ String id; String name; public Student(String id,String name){ this.id=id; this.name=name; } public String toString(){ String s = id + ":" + name; return s; } } public class Set1 { public static void main(String[] args) { HashSet hs=new HashSet(); Student stu1=new Student("1","hello"); Student stu2=new Student("2","world"); Student stu3=new Student("1","hello"); hs.add(stu1); hs.add(stu2); hs.add(stu3); System.out.println(hs); } }Running results
package API; import java.util.HashSet; class Student{ private String id; private String name; public Student(String id,String name){ this.id=id; this.name=name; } //重写toString方法 public String toString(){ return id+ ":"+name; } //重写hashCode方法 public int hashCode(){ //返回id属性的哈希值 return id.hashCode(); } public boolean equals(Object obj){ //判断是否是同一个对象 if(this==obj){ return true; } //判断对象是Student类型 if(!(obj instanceof Student)){ return false; } //将对象强转为Student类型 Student stu=(Student) obj; //判断id是否相同 boolean b=this.id.equals(stu.id); return b; } } public class Set2 { public static void main(String[] args) { HashSet hs=new HashSet(); Student stu1=new Student("1","hello"); Student stu2=new Student("2","world"); Student stu3=new Student("1","hello"); hs.add(stu1); hs.add(stu2); hs.add(stu3); System.out.println(hs); } }Running results Because the Student class overrides the hashCode() and equals() methods of the Object class. Return the hash value of the id attribute in the hashCode() method, compare whether the id attributes of the objects are equal in the equals() method, and return the result. When the add() method of the HashSet collection is called to add the stu3 object, it is found that its hash value is the same as the stu2 object, and stu2.equals(stu3) returns true. HashSet determines that the two objects are the same, so the duplicate Student objects are removed.
The above is the detailed content of How to use Set interface in Java to store arrays without duplicate elements. For more information, please follow other related articles on the PHP Chinese website!
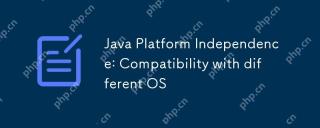
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
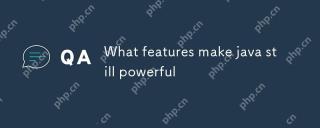
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
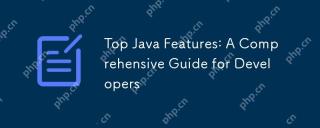
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
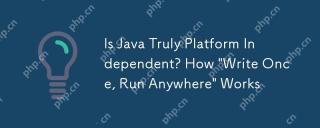
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
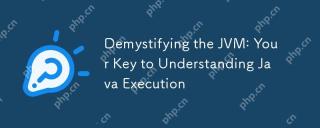
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
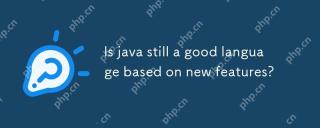
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
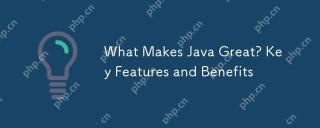
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
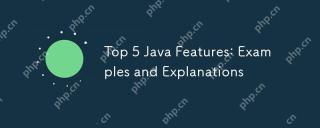
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
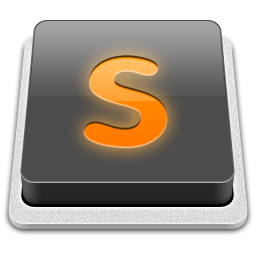
SublimeText3 Mac version
God-level code editing software (SublimeText3)
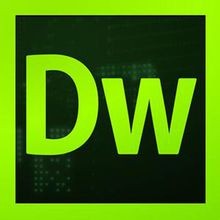
Dreamweaver CS6
Visual web development tools
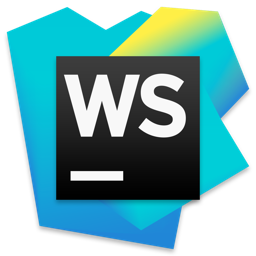
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
