1. The role of paging function
The paging function is an indispensable part of various websites and systems (such as pagination of Baidu search results, etc.). When a page has a large amount of data, the paging function is reflected. It has the following five functions.
(1) Reduce the consumption of system resources
(2) Improve the query performance of the database
(3) Improve the page access speed
(4 ) In line with the user’s browsing habits
(5) Adapt to the layout of the page
2. Establish a test database
Due to the need to implement the paging function, more data is required
DROP TABLE IF EXISTS tb_user; CREATE TABLE tb_user ( id int(11) NOT NULL AUTO_INCREMENT COMMENT '主键id', name varchar(100) NOT NULL DEFAULT '' COMMENT '登录名', password varchar(100) NOT NULL DEFAULT '' COMMENT '密码', PRIMARY KEY (id) USING BTREE ) ENGINE = InnoDB CHARACTER SET = utf8; insert into tb_user (id,name,password) value (1,'C','123456'), (2,'C++','123456'), (3,'Java','123456'), (4,'Python','123456'), (5,'R','123456'), (6,'C#','123456'); insert into tb_user (id,name,password) value (7,'test1','123456'); insert into tb_user (id,name,password) value (8,'test2','123456'); insert into tb_user (id,name,password) value (9,'test3','123456'); insert into tb_user (id,name,password) value (10,'test4','123456'); insert into tb_user (id,name,password) value (11,'test5','123456'); insert into tb_user (id,name,password) value (12,'test6','123456'); insert into tb_user (id,name,password) value (13,'test7','123456'); insert into tb_user (id,name,password) value (14,'test8','123456'); insert into tb_user (id,name,password) value (15,'test9','123456'); insert into tb_user (id,name,password) value (16,'test10','123456'); insert into tb_user (id,name,password) value (17,'test11','123456'); insert into tb_user (id,name,password) value (18,'test12','123456'); insert into tb_user (id,name,password) value (19,'test13','123456'); insert into tb_user (id,name,password) value (20,'test14','123456'); insert into tb_user (id,name,password) value (21,'test15','123456'); insert into tb_user (id,name,password) value (22,'test16','123456'); insert into tb_user (id,name,password) value (23,'test17','123456'); insert into tb_user (id,name,password) value (24,'test18','123456'); insert into tb_user (id,name,password) value (25,'test19','123456'); insert into tb_user (id,name,password) value (26,'test20','123456'); insert into tb_user (id,name,password) value (27,'test21','123456'); insert into tb_user (id,name,password) value (28,'test22','123456'); insert into tb_user (id,name,password) value (29,'test23','123456'); insert into tb_user (id,name,password) value (30,'test24','123456'); insert into tb_user (id,name,password) value (31,'test25','123456'); insert into tb_user (id,name,password) value (32,'test26','123456'); insert into tb_user (id,name,password) value (33,'test27','123456'); insert into tb_user (id,name,password) value (34,'test28','123456'); insert into tb_user (id,name,password) value (35,'test29','123456'); insert into tb_user (id,name,password) value (36,'test30','123456'); insert into tb_user (id,name,password) value (37,'test31','123456'); insert into tb_user (id,name,password) value (38,'test32','123456'); insert into tb_user (id,name,password) value (39,'test33','123456'); insert into tb_user (id,name,password) value (40,'test34','123456'); insert into tb_user (id,name,password) value (41,'test35','123456'); insert into tb_user (id,name,password) value (42,'test36','123456'); insert into tb_user (id,name,password) value (43,'test37','123456'); insert into tb_user (id,name,password) value (44,'test38','123456'); insert into tb_user (id,name,password) value (45,'test39','123456'); insert into tb_user (id,name,password) value (46,'test40','123456'); insert into tb_user (id,name,password) value (47,'test41','123456'); insert into tb_user (id,name,password) value (48,'test42','123456'); insert into tb_user (id,name,password) value (49,'test43','123456'); insert into tb_user (id,name,password) value (50,'test44','123456'); insert into tb_user (id,name,password) value (51,'test45','123456');
3. Encapsulation of the results returned by the paging function
Create a util package and create a new Result general result class in the package. The code is as follows:
package ltd.newbee.mall.entity; public class User { private Integer id; private String name; private String password; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } }
Data returned by the back-end interface Data will be encapsulated according to the above format, including business code, return information, and actual data results. This format is set by the developers themselves, and can be adjusted appropriately if there are other better solutions.
Create a new PageResult general result class in the util package, the code is as follows:
package ltd.newbee.mall.util; import java.util.List; /** * 分页工具类 */ public class PageResult { //总记录数 private int totalCount; //每页记录数 private int pageSize; //总页数 private int totalPage; //当前页数 private int currPage; //列表数据 private List<?> list; /** * * @param totalCount 总记录数 * @param pageSize 每页记录数 * @param currPage 当前页数 * @param list 列表数据 */ public PageResult(int totalCount, int pageSize, int currPage, List<?> list) { this.totalCount = totalCount; this.pageSize = pageSize; this.currPage = currPage; this.list = list; this.totalPage = (int) Math.ceil((double) totalCount / pageSize); } public int getTotalCount() { return totalCount; } public void setTotalCount(int totalCount) { this.totalCount = totalCount; } public int getPageSize() { return pageSize; } public void setPageSize(int pageSize) { this.pageSize = pageSize; } public int getTotalPage() { return totalPage; } public void setTotalPage(int totalPage) { this.totalPage = totalPage; } public int getCurrPage() { return currPage; } public void setCurrPage(int currPage) { this.currPage = currPage; } public List<?> getList() { return list; } public void setList(List<?> list) { this.list = list; } }
4. Specific implementation of paging function code
4.1 Data layer
In UserDao Two new methods, findUsers() and getTotalUser(), are added to the interface. The code is as follows:
/** * 返回分页数据列表 * * @param pageUtil * @return */ List<User> findUsers(PageQueryUtil pageUtil); /** * 返回数据总数 * * @param pageUtil * @return */ int getTotalUser(PageQueryUtil pageUtil);
Add the mapping statements of these two methods in the UserMapper.xml file. The code is as follows:
<!--分页--> <!--查询用户列表--> <select id="findUsers" parameterType="Map" resultMap="UserResult"> select id,name,password from tb_user order by id desc <if test="start!=null and limit!=null"> limit #{start}.#{limit} </if> </select> <!--查询用户总数--> <select id="getTotalUser" parameterType="Map" resultType="int"> select count(*) from tb_user </select>
4.2 Business layer
Create a new service package and add a new business class UserService. The code is as follows:
import ltd.newbee.mall.dao.UserDao; import ltd.newbee.mall.entity.User; import ltd.newbee.mall.util.PageResult; import ltd.newbee.mall.util.PageQueryUtil; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import java.util.List; @Service public class UserService { @Autowired private UserDao userDao; public PageResult getUserPage(PageQueryUtil pageUtil){ //当前页面中的数据列表 List<User> users = userDao.findUsers(pageUtil); //数据总条数,用于计算分页数据 int total = userDao.getTotalUser(pageUtil); //分页信息封装 PageResult pageResult = new PageResult(users,total,pageUtil.getLimit(),pageUtil.getPage()); return pageResult; } }
First query the current page based on the current page and the number of items per page Data collection, then call the select count (*) statement to query the total number of data to calculate the paging data, and finally encapsulate the obtained data into a PageResult object and return it to the control layer.
4.3 Control layer
Create a new PageTestController class in the controller package to process paging requests and return query results. The code is as follows:
@RestController @RequestMapping("users") public class PageTestController { @Autowired private UserService userService; //分页功能测试 @RequestMapping(value = "/list",method = RequestMethod.GET) public Result list(@RequestParam Map<String,Object> params){ Result result = new Result(); if (StringUtils.isEmpty(params.get("page"))||StringUtils.isEmpty(params.get("limit"))){ //返回错误码 result.setResultCode(500); //错误信息 result.setMessage("参数异常!"); return result; } //封装查询参数 PageQueryUtil queryParamList = new PageQueryUtil(params); //查询并封装分页结果集 PageResult userPage = userService.getUserPage(queryParamList); //返回成功码 result.setResultCode(200); result.setMessage("查询成功"); //返回分页数据 result.setData(userPage); return result; } }
Paging function Interaction process: The front-end transmits the required page number and number of items parameters to the back-end. The back-end calculates the paging parameters after receiving the paging request, and uses the limit keyword of MySQL to query the corresponding records. The query results are encapsulated and returned to front end. The @RestController annotation is used on the TestUserControler class, which is equivalent to the combined annotation of @ResponseBody+@Controller.
5.jqGrid paging plug-in
jqGrid is a jQuery plug-in used to display grid data. By using jqGrid, developers can easily implement Ajax asynchronous communication between the front-end page and the background data and implement paging functions. At the same time, jqGrid is an open source paging plug-in, and the source code has been in a state of iterative updates.
Download address: jqGrid
After downloading jqGrid, unzip the file and drag the unzipped file directly into the static directory of the project
The following are Steps to implement paging in jqGrid:
First, introduce the source files required by the jqGrid paging plug-in into the front-end page code. The code is as follows:
<link href="plugins/jqgrid-5.8.2/ui.jqgrid-bootstrap4.css" rel="external nofollow" rel="stylesheet"/> <!--jqGrid依赖jQuery,因此需要先引入jquery.min.js文件,下方地址为字节跳动提供的cdn地址--> <script src="http://s3.pstatp.com/cdn/expire-1-M/jquery/3.3.1/jquery.min.js"></script> <!--grid.locale-cn.js为国际化所需的文件,-cn表示中文--> <script src="plugins/jqgrid-5.8.2/grid.locale-cn.js"></script> <script src="plugins/jqgrid-5.8.2/jquery.jqGrid.min.js"></script>
Secondly, the paging data needs to be displayed on the page Add the code for jqGrid initialization in the area:
<!--jqGrid必要DOM,用于创建表格展示列表数据--> <table id="jqGrid" class="table table-bordered"></table> <!--jqGrid必要DOM,分页信息区域--> <div id="jqGridPager"></div>
Finally, call the jqGrid() method of the jqGrid paging plug-in to render the paging display area. The code is as follows:
The parameters and meanings in the jqGrid() method are as shown in the figure.
jqGrid front-end page test:
Create a new jqgrid-page-test.html file in the resources/static directory, the code is as follows:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>jqGrid分页测试</title> <!--引入bootstrap样式文件--> <link rel="stylesheet" href="/static/bootstrap-5.3.0-alpha3-dist/css/bootstrap.css" rel="external nofollow" /> <link href="jqGrid-5.8.2/css/ui.jqgrid-bootstrap4.css" rel="external nofollow" rel="stylesheet"/> </head> <body> <div > <!--数据展示列表,id为jqGrid--> <table id="jqGrid" class="table table-bordered"></table> <!--分页按钮展示区--> <div id="jqGridPager"></div> </div> </body> <!--jqGrid依赖jQuery,因此需要先引入jquery.min.js文件,下方地址为字节跳动提供的cdn地址--> <script src="http://s3.pstatp.com/cdn/expire-1-M/jquery/3.3.1/jquery.min.js"></script> <!--grid.locale-cn.js为国际化所需的文件,-cn表示中文--> <script src="plugins/jqgrid-5.8.2/grid.locale-cn.js"></script> <script src="plugins/jqgrid-5.8.2/jquery.jqGrid.min.js"></script> <script src="jqgrid-page-test.js"></script> </html>
jqGrid initialization
Create a new jqgrid-page-test.js file in the resources/static directory. The code is as follows:
$(function () { $("#jqGrid").jqGrid({ url: 'users/list', datatype: "json", colModel: [ {label: 'id',name: 'id', index: 'id', width: 50, hidden: true,key:true}, {label: '登录名',name: 'name',index: 'name', sortable: false, width: 80}, {label: '密码字段',name: 'password',index: 'password', sortable: false, width: 80} ], height: 485, rowNum: 10, rowList: [10,30,50], styleUI: 'Bootstrap', loadtext: '信息读取中...', rownumbers: true, rownumWidth: 35, autowidth: true, multiselect: true, pager: "#jqGridPager", jsonReader:{ root: "data.list", page: "data.currPage", total: "data.totalCount" }, prmNames:{ page: "page", rows: "limit", order: "order" }, gridComplete: function () { //隐藏grid底部滚动条 $("#jqGrid").closest(".ui-jqgrid-bdiv").css({"overflow-x": "hidden"}); } }); $(window).resize(function () { $("jqGrid").setGridWidth($(".card-body").width()); }); });
The above is the detailed content of How to implement springboot paging function. For more information, please follow other related articles on the PHP Chinese website!
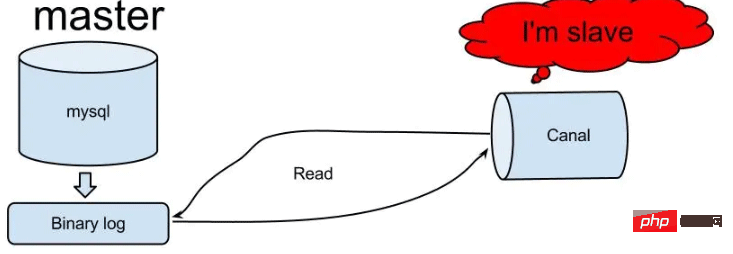
Canal工作原理Canal模拟MySQLslave的交互协议,伪装自己为MySQLslave,向MySQLmaster发送dump协议MySQLmaster收到dump请求,开始推送binarylog给slave(也就是Canal)Canal解析binarylog对象(原始为byte流)MySQL打开binlog模式在MySQL配置文件my.cnf设置如下信息:[mysqld]#打开binloglog-bin=mysql-bin#选择ROW(行)模式binlog-format=ROW#配置My
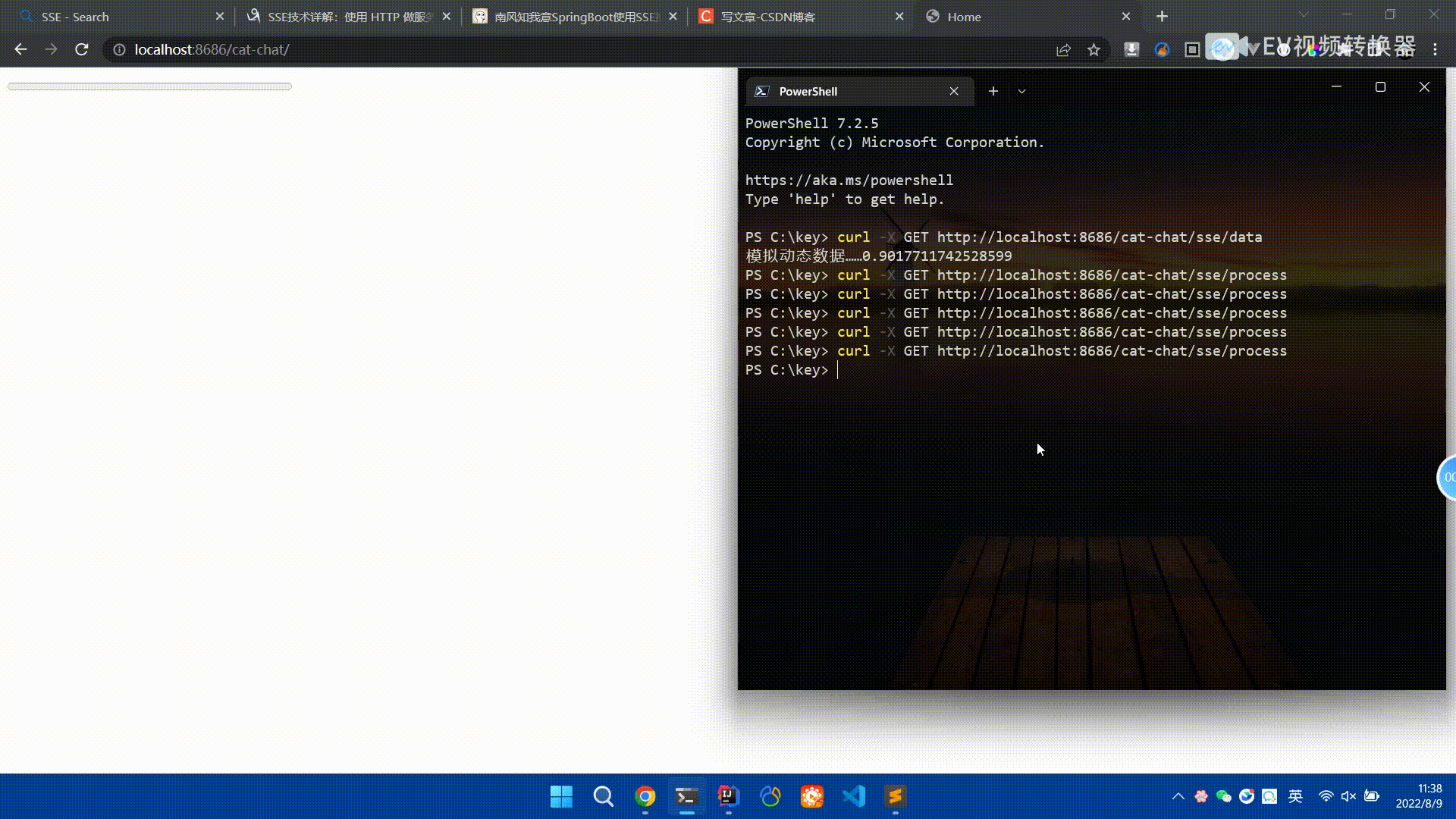
前言SSE简单的来说就是服务器主动向前端推送数据的一种技术,它是单向的,也就是说前端是不能向服务器发送数据的。SSE适用于消息推送,监控等只需要服务器推送数据的场景中,下面是使用SpringBoot来实现一个简单的模拟向前端推动进度数据,前端页面接受后展示进度条。服务端在SpringBoot中使用时需要注意,最好使用SpringWeb提供的SseEmitter这个类来进行操作,我在刚开始时使用网上说的将Content-Type设置为text-stream这种方式发现每次前端每次都会重新创建接。最
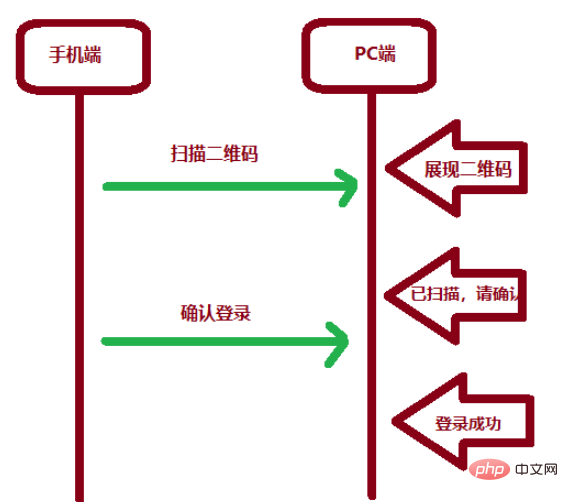
一、手机扫二维码登录的原理二维码扫码登录是一种基于OAuth3.0协议的授权登录方式。在这种方式下,应用程序不需要获取用户的用户名和密码,只需要获取用户的授权即可。二维码扫码登录主要有以下几个步骤:应用程序生成一个二维码,并将该二维码展示给用户。用户使用扫码工具扫描该二维码,并在授权页面中授权。用户授权后,应用程序会获取一个授权码。应用程序使用该授权码向授权服务器请求访问令牌。授权服务器返回一个访问令牌给应用程序。应用程序使用该访问令牌访问资源服务器。通过以上步骤,二维码扫码登录可以实现用户的快
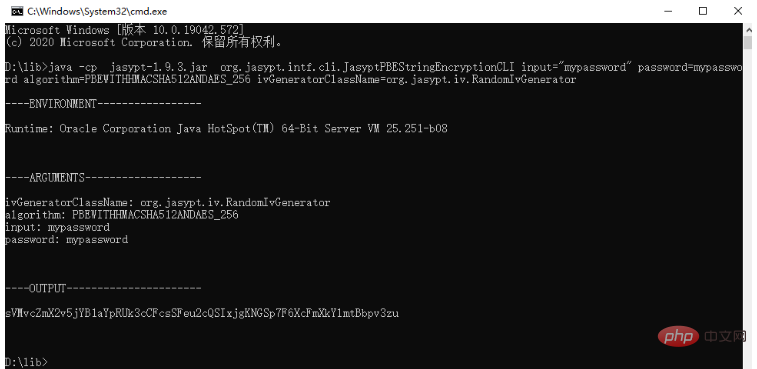
我们使用jasypt最新版本对敏感信息进行加解密。1.在项目pom文件中加入如下依赖:com.github.ulisesbocchiojasypt-spring-boot-starter3.0.32.创建加解密公用类:packagecom.myproject.common.utils;importorg.jasypt.encryption.pbe.PooledPBEStringEncryptor;importorg.jasypt.encryption.pbe.config.SimpleStrin
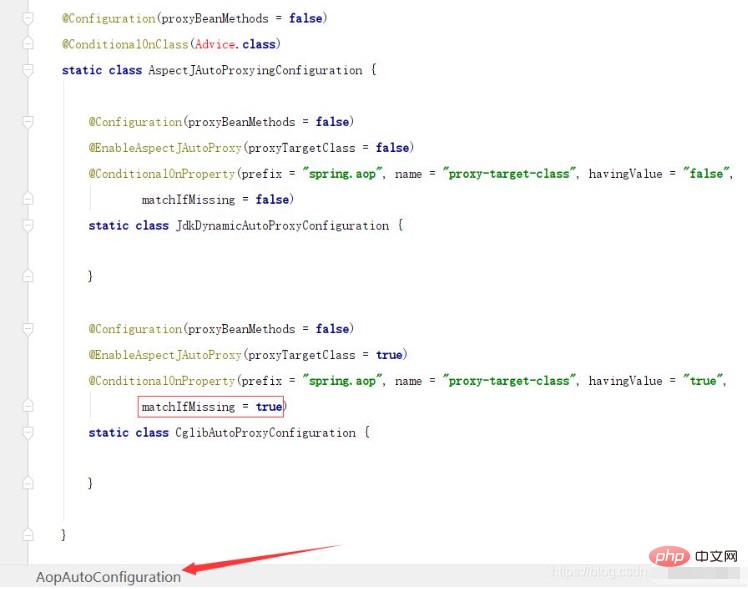
1.springboot2.x及以上版本在SpringBoot2.xAOP中会默认使用Cglib来实现,但是Spring5中默认还是使用jdk动态代理。SpringAOP默认使用JDK动态代理,如果对象没有实现接口,则使用CGLIB代理。当然,也可以强制使用CGLIB代理。在SpringBoot中,通过AopAutoConfiguration来自动装配AOP.2.Springboot1.xSpringboot1.xAOP默认还是使用JDK动态代理的3.SpringBoot2.x为何默认使用Cgl
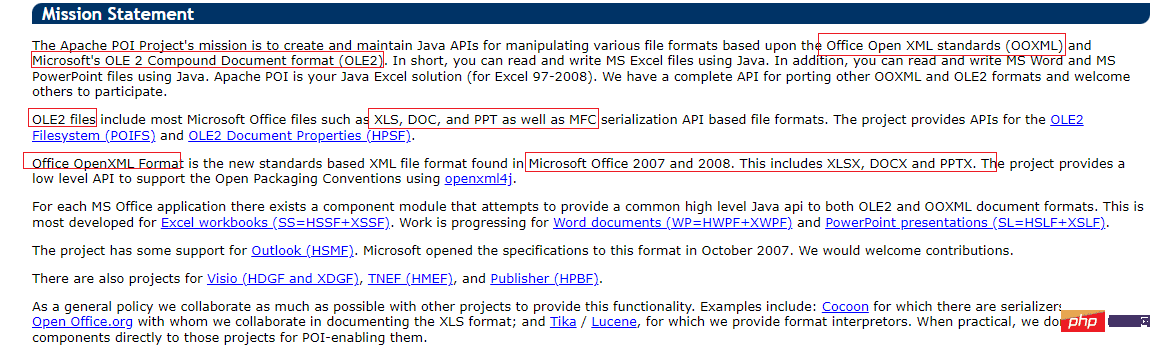
知识准备需要理解ApachePOI遵循的标准(OfficeOpenXML(OOXML)标准和微软的OLE2复合文档格式(OLE2)),这将对应着API的依赖包。什么是POIApachePOI是用Java编写的免费开源的跨平台的JavaAPI,ApachePOI提供API给Java程序对MicrosoftOffice格式档案读和写的功能。POI为“PoorObfuscationImplementation”的首字母缩写,意为“简洁版的模糊实现”。ApachePOI是创建和维护操作各种符合Offic
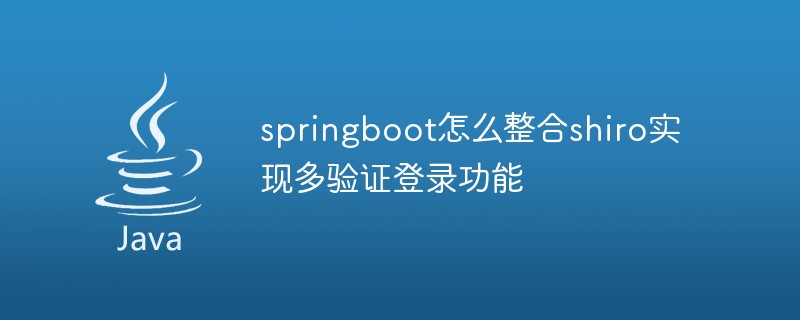
1.首先新建一个shiroConfigshiro的配置类,代码如下:@ConfigurationpublicclassSpringShiroConfig{/***@paramrealms这儿使用接口集合是为了实现多验证登录时使用的*@return*/@BeanpublicSecurityManagersecurityManager(Collectionrealms){DefaultWebSecurityManagersManager=newDefaultWebSecurityManager();

先说遇到问题的情景:初次尝试使用springboot框架写了个小web项目,在IntellijIDEA中能正常启动运行。使用maven运行install,生成war包,发布到本机的tomcat下,出现异常,主要的异常信息是.......LifeCycleException。经各种搜索,找到答案。springboot因为内嵌tomcat容器,所以可以通过打包为jar包的方法将项目发布,但是如何将springboot项目打包成可发布到tomcat中的war包项目呢?1.既然需要打包成war包项目,首


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
