In the development model where the front and back ends are separated, it is often necessary to send HTTP requests on the front end and respond on the back end. However, due to some reasons, sometimes the front end needs to communicate directly with the external system, so it is necessary to do so on the back end. The client forwards HTTP requests. This article will introduce how to use Golang to implement HTTP request forwarding.
First, we need to install the Golang environment and install the corresponding dependency packages. Next, we can start writing code.
package main import ( "log" "net/http" ) func main() { http.HandleFunc("/", handleRequestAndRedirect) log.Fatal(http.ListenAndServe(":8080", nil)) } func handleRequestAndRedirect(w http.ResponseWriter, r *http.Request) { url := "http://example.com" + r.URL.Path log.Printf("Redirecting to %s", url) req, err := http.NewRequest(r.Method, url, r.Body) if err != nil { http.Error(w, err.Error(), http.StatusInternalServerError) return } // 设置请求头 for name, values := range r.Header { for _, value := range values { req.Header.Add(name, value) } } client := http.Client{} resp, err := client.Do(req) if err != nil { http.Error(w, err.Error(), http.StatusBadGateway) return } defer resp.Body.Close() // 将响应转发到客户端 for name, values := range resp.Header { for _, value := range values { w.Header().Add(name, value) } } w.WriteHeader(resp.StatusCode) if _, err := io.Copy(w, resp.Body); err != nil { log.Printf("Error copying to client: %v", err) } }
The above code implements a basic function of HTTP request forwarding. The handleRequestAndRedirect function in the code receives the request from the client and requests the specified external system according to the request path. The code reads the header of the HTTP request, adds relevant information to the request header, and sends the HTTP request using the http package in Go's standard library. Subsequently, the response from the external system is received and forwarded to the client. It is worth noting that this code also handles error conditions and returns error information to the client.
If we need to implement more functions, such as request retry, request logging, etc., we can modify the above code appropriately. In terms of request retry, we can add the logic to resend the request after the request fails; in terms of request logging, we can add code to record the request log.
In summary, this article implements an example of HTTP request forwarding based on Golang. This allows the front-end to communicate directly with external systems without the need for back-end forwarding, improving the accessibility of the front-end interface. Of course, in practical applications, we need to add more functions according to specific needs to adapt to complex business scenarios.
The above is the detailed content of golang http request forwarding. For more information, please follow other related articles on the PHP Chinese website!
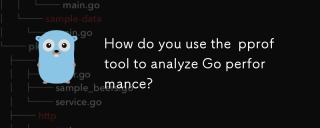
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
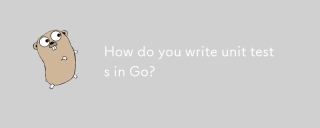
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
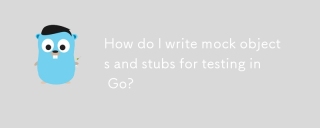
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
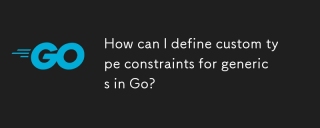
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
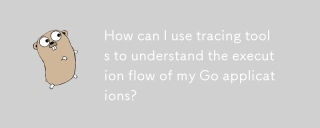
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
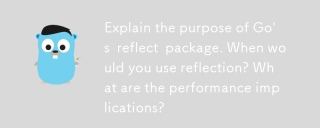
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
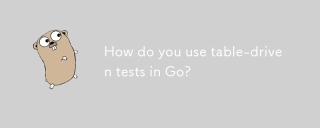
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
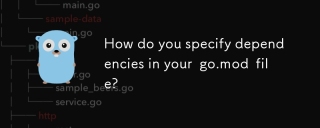
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
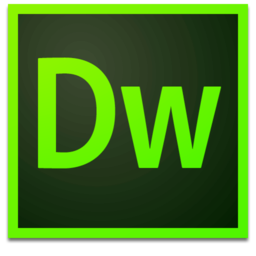
Dreamweaver Mac version
Visual web development tools
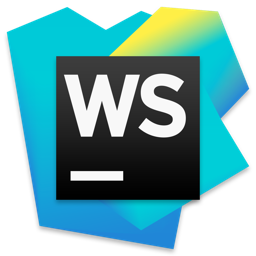
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
