Go is a strongly typed, statically typed, concurrency-supported programming language. Its core concepts are simplicity, efficiency and maintainability.
In Go, the for-range loop is a convenient and commonly used iteration method. It can be used to traverse data structures such as arrays, slices, and maps. This article will introduce in detail how to use for-range loops to traverse various data structures, and explore its advantages and disadvantages.
- Traverse arrays
Arrays are one of the most basic data structures in Go. It stores elements of the same type, arranged in order. You can use a for-range loop to iterate over array elements.
The following is a sample program that shows how to iterate over an array using the for-range statement.
package main import "fmt" func main() { nums := [5]int{1, 2, 3, 4, 5} for i, num := range nums { fmt.Printf("Index: %v, Value: %v ", i, num) } }
Output:
Index: 0, Value: 1 Index: 1, Value: 2 Index: 2, Value: 3 Index: 3, Value: 4 Index: 4, Value: 5
In this example, we first define an array nums containing 5 integers. We then use a for-range loop to iterate through the array, storing the index and value of the elements in the variables i and num. Finally, we print out the index and value of each element.
- Traversing through slices
In Go, a slice is a sequence of variable length. It is an array-based abstraction with more flexibility and convenience. You can use a for-range loop to iterate over slice elements.
The following is a sample program that shows how to iterate over a slice using a for-range statement.
package main import "fmt" func main() { fruits := []string{"apple", "banana", "orange"} for i, fruit := range fruits { fmt.Printf("Index: %v, Value: %v ", i, fruit) } }
Output:
Index: 0, Value: apple Index: 1, Value: banana Index: 2, Value: orange
In this example, we first create a slice of 3 strings using the slice literal. We then use a for-range loop to iterate over the slice, storing the index and value of the elements in the variables i and fruit. Finally, we print the index and value of each element.
- Traverse map
In Go, map is a data structure used to store key-value pairs. You can use a for-range loop to iterate over the key-value pairs in the map.
The following is a sample program that shows how to use a for-range statement to traverse a map.
package main import "fmt" func main() { scores := map[string]int{ "Alice": 90, "Bob": 80, "Charlie": 70, } for name, score := range scores { fmt.Printf("%v's Score: %v ", name, score) } }
Output:
Alice's Score: 90 Bob's Score: 80 Charlie's Score: 70
In this example, we first use the map literal to create a map containing three key-value pairs. We then use a for-range loop to iterate over the key-value pairs in the map, storing the keys in the variable name and the values in the variable score. Finally, we print everyone's score.
- Traverse the string
The string is an immutable sequence, and you can use a for-range loop to traverse the characters in the string.
The following is a sample program that shows how to use the for-range statement to iterate over the characters in a string.
package main import "fmt" func main() { str := "Hello, 世界" for i, ch := range str { fmt.Printf("Index: %v, Character: %c ", i, ch) } }
Output:
Index: 0, Character: H Index: 1, Character: e Index: 2, Character: l Index: 3, Character: l Index: 4, Character: o Index: 5, Character: , Index: 6, Character: Index: 7, Character: 世 Index: 10, Character: 界
In this example, we define a string str containing English and Chinese characters. We then use a for-range loop to iterate over the characters in the string, storing the index of the character in the variable i and the value of the character in the variable ch.
- Advantages and Disadvantages of for-range
The for-range loop is a convenient and commonly used method that can be used to traverse various data structures. The following are the advantages and disadvantages of for-range loops:
Advantages:
- Easy to understand: The syntax is simple and easy to understand.
- Safe and stable: No out-of-bounds errors will occur when using for-range loops.
- Concise code: Use for-range loops to reduce redundant code.
Disadvantages:
- Require index: In some cases, if we only need the value and not the index, using a for-range loop may affect the code performance .
- Not suitable for all situations: in some situations, it is necessary to use a traditional for loop or while loop.
To sum up, the for-range loop is an effective iteration method that can be used to traverse various data structures. It is simple and easy to understand, but in some cases it is necessary to use other types of loops.
The above is the detailed content of golang for range implementation. For more information, please follow other related articles on the PHP Chinese website!
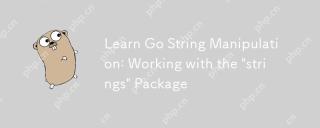
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
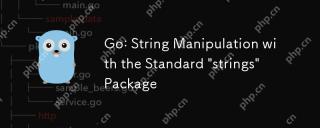
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
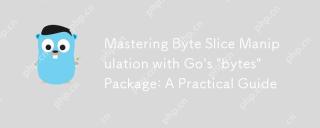
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
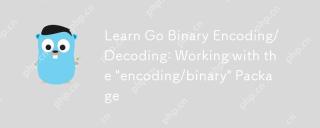
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
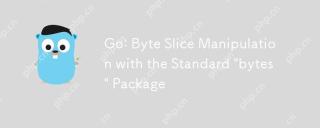
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
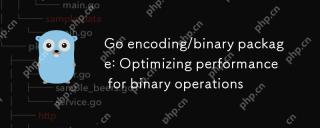
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
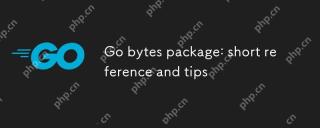
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
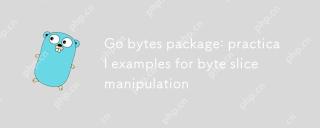
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
