Preface
The traditional Restful API has many problems. First of all, it cannot control the fields returned, and the front-end cannot predict the return results of the back-end. In addition, different return results correspond to different request addresses. This is This resulted in multiple requests. GraphQL is an API query language built based on this background. Compared with traditional Restful API, it has the following advantages:
Flexibility: GraphQL can be flexible according to the needs of the client. Query data efficiently instead of returning fixed-structured data like RESTful API.
Reduce network requests: GraphQL allows clients to obtain multiple resources in a single request, which helps reduce the number of network requests and improve performance.
Strong typing: GraphQL has a strongly typed system that allows clients to detect errors in queries at compile time, which helps reduce runtime errors.
Cacheable: GraphQL is cacheable, which means the server can cache the results of a query, improving performance and scalability.
Documentation: GraphQL has the ability to self-document, allowing developers to quickly understand the structure and functions of the API.
Implementation plan of GraphQL in Spring Boot
If the back-end language is Java, then GraphQL Java is the basic library for implementing GraphQL. In addition, Spring has integrated GraphQL. If Spring is used in the project, Spring GraphQL is more recommended.
The development of Spring GraphQL is generally divided into the following steps
Add Spring GraphQL dependencies
Add Spring GraphQL dependencies in your project. You can add dependencies through build tools like Maven or Gradle. For example, if you use Maven, you can add the following dependency
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-graphql</artifactId> </dependency>
Define GraphQL Schema
Define GraphQL Schema in your application. Schema defines queryable types and fields. You can define a schema using SDL (Schema Definition Language) or programmatically.
For the Spring Boot project, the schema file is placed in the resources/graphql/ directory, and the file name is suffixed with graphqls. The following is a simple schema.graphqls that I defined.
It specifies two query implementations. author(id:Int) means querying the Author by id, and allAuthors means querying the Author array.
schema {
query: Query
}type Query {
author(id:Int): Author
allAuthors: [Author]
}type Author {
id:Int
firstName:String
lastName:String
email:String
birthdate:String
}
Implementing RuntimeWiringConfigurer
RuntimeWiringConfigurer is the core of implementing GraphQL to obtain data. Using GraphQL cannot directly remove persistence layer frameworks such as Mybatis/Jpa. Obtaining data from the database still requires the support of such frameworks.
The RuntimeWiringConfigurer is similar to the service layer in Spring, which is the core of implementing basic data.
The following is a simple example:
@Component public class AuthorWiring implements RuntimeWiringConfigurer { private final AuthorRepository authorRepository; public AuthorWiring(AuthorRepository authorRepository) { this.authorRepository = authorRepository; } @Override public void configure(RuntimeWiring.Builder builder) { builder.type("Query", typeWiring -> typeWiring .dataFetcher("allAuthors", environment -> authorRepository.findAll()) .dataFetcher("author", environment -> authorRepository.getReferenceById(environment.getArgument("id"))) } }
Here, two DataFetcher objects are defined inside the configure method to specify how author and allAuthors query data. It can be seen that JPA is still used to query data. Query data.
Define GraphQL Controller
We define GraphQLController to receive the input parameters of web requests. The example is as follows:
@RestController @RequestMapping("graphql") public class GraphQLController { private final GraphQL graphQL; @Autowired public GraphQLController(GraphQlSource graphQlSource) { graphQL = graphQlSource.graphQl(); } @PostMapping("query") public ResponseEntity<Object> query(@RequestBody String query) { ExecutionResult result = graphQL.execute(query); return ResponseEntity.ok(result.getData()); } }
The GraphQL object in the code is the entrance to execute the query, but GraphQL There is only one private constructor, so it cannot be injected directly. You must obtain the GraphQL object by injecting GraphQlSource.
Note that in GraphQL we can only use String to receive parameters, and cannot use model objects. This is because the Graph request parameters are not json structures.
Test Graph request
We create a graphql.http file to perform http requests in idea
}Content-Type: application/json
Send POST request with json body
POST http://localhost:8080/graphql/query}
{
author(id: 1) {
id
firstName
lastName
birthdate
}POST http://localhost:8080/graphql/query
Send POST request with json bodyContent -Type: application/json
}
{
allAuthors {
id
firstName
lastName
birthdate
Run the query for author(id: 1) and you can see that the results are returned normally. If we only need the firstName and lastName fields, then just remove the id and birthdate from the request input parameters without changing any back-end code.
The above is the detailed content of How SpringBoot uses GraphQL to develop Web API. For more information, please follow other related articles on the PHP Chinese website!
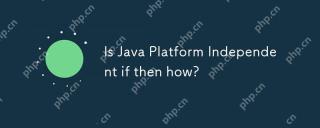
Java is platform-independent because of its "write once, run everywhere" design philosophy, which relies on Java virtual machines (JVMs) and bytecode. 1) Java code is compiled into bytecode, interpreted by the JVM or compiled on the fly locally. 2) Pay attention to library dependencies, performance differences and environment configuration. 3) Using standard libraries, cross-platform testing and version management is the best practice to ensure platform independence.
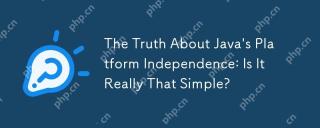
Java'splatformindependenceisnotsimple;itinvolvescomplexities.1)JVMcompatibilitymustbeensuredacrossplatforms.2)Nativelibrariesandsystemcallsneedcarefulhandling.3)Dependenciesandlibrariesrequirecross-platformcompatibility.4)Performanceoptimizationacros
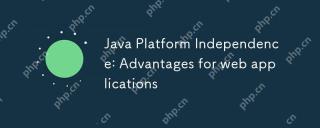
Java'splatformindependencebenefitswebapplicationsbyallowingcodetorunonanysystemwithaJVM,simplifyingdeploymentandscaling.Itenables:1)easydeploymentacrossdifferentservers,2)seamlessscalingacrosscloudplatforms,and3)consistentdevelopmenttodeploymentproce
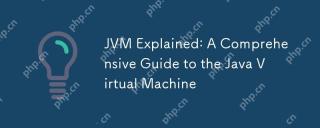
TheJVMistheruntimeenvironmentforexecutingJavabytecode,crucialforJava's"writeonce,runanywhere"capability.Itmanagesmemory,executesthreads,andensuressecurity,makingitessentialforJavadeveloperstounderstandforefficientandrobustapplicationdevelop
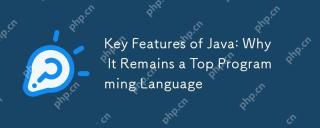
Javaremainsatopchoicefordevelopersduetoitsplatformindependence,object-orienteddesign,strongtyping,automaticmemorymanagement,andcomprehensivestandardlibrary.ThesefeaturesmakeJavaversatileandpowerful,suitableforawiderangeofapplications,despitesomechall
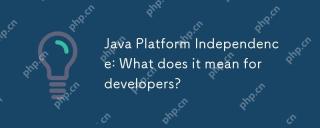
Java'splatformindependencemeansdeveloperscanwritecodeonceandrunitonanydevicewithoutrecompiling.ThisisachievedthroughtheJavaVirtualMachine(JVM),whichtranslatesbytecodeintomachine-specificinstructions,allowinguniversalcompatibilityacrossplatforms.Howev
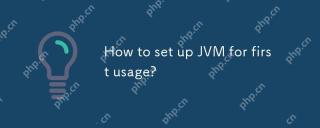
To set up the JVM, you need to follow the following steps: 1) Download and install the JDK, 2) Set environment variables, 3) Verify the installation, 4) Set the IDE, 5) Test the runner program. Setting up a JVM is not just about making it work, it also involves optimizing memory allocation, garbage collection, performance tuning, and error handling to ensure optimal operation.
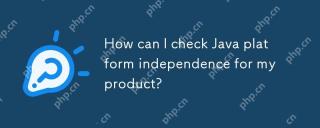
ToensureJavaplatformindependence,followthesesteps:1)CompileandrunyourapplicationonmultipleplatformsusingdifferentOSandJVMversions.2)UtilizeCI/CDpipelineslikeJenkinsorGitHubActionsforautomatedcross-platformtesting.3)Usecross-platformtestingframeworkss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
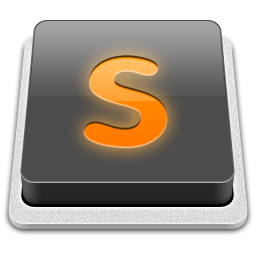
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
