In web development, JavaScript is an important programming language. It can help developers increase the interactivity of web pages, thereby improving user experience. And links are also one of the indispensable elements in web pages. In this article, we'll explore how to use JavaScript to set up links to make your web pages more interactive and flexible.
1. Basic hyperlinks
First, let us familiarize ourselves with basic hyperlinks. A basic HTML hyperlink looks like this:
<a href="https://www.example.com">Link Text</a>
Where the href
attribute is used to define the target URL of the link, and Link Text
is the text visible to the user. When the user clicks the link, the page will reload and take the user to the target URL.
2. Open the link to a new tab
Sometimes, we want the link to open in a new tab after the user clicks it, instead of loading the target URL in the current page. Using JavaScript, we can use the following code to achieve this function:
document.querySelector('a').addEventListener('click', function(event) { event.preventDefault(); window.open(this.href, '_blank'); });
This code uses the addEventListener()
function to listen for the click
event. The event.preventDefault()
function prevents the default behavior of loading the target URL in the current tab when a click event occurs. Afterwards, the window.open()
function is used, passing in the URL of the target link and the _blank
parameter, telling the browser to open the link in a new tab.
3. Add hover effect to link
It is very simple for us to use JavaScript to add hover effect to link. Just add listeners for the mouseenter
and mouseleave
events to the link to trigger the effect when the mouse is hovering. For example:
document.querySelector('a').addEventListener('mouseenter', function() { this.style.color = 'red'; }); document.querySelector('a').addEventListener('mouseleave', function() { this.style.color = ''; });
In this example, we use this
to reference the link element. On mouseover, we set the font color of the link to red. Resets link style to default after mouseover.
4. Dynamically adjust the link
Sometimes, we will need to change the link as a result of the user's interaction with the web page. Using JavaScript, we can very easily dynamically affect the properties of a link, such as the link's href, target or title, etc. For example:
document.querySelector('a').addEventListener('click', function() { this.href = 'https://www.new-link.com'; this.target = '_blank'; this.title = 'New Page'; });
In this example, when the user clicks on the link, the JavaScript code will change the link properties. The link's href
will be changed to point to https://www.new-link.com
, and the target
attribute will be set to _blank
, and the title
attribute will be changed to New Page
.
5. Dynamically update the link based on the scroll position
We can also use JavaScript to dynamically update the link status based on the user's scroll position. This technique can come in handy if you want to display a "back to top" link at the top of your website when the user scrolls to a certain point on the page. The following is a sample code on how to achieve this effect:
window.addEventListener('scroll', function() { var scrollPosition = window.scrollY; var link = document.querySelector('.back-to-top'); if (scrollPosition > 500) { link.classList.add('show'); } else { link.classList.remove('show'); } });
In this example, we use the window.addEventListener()
function to listen for the scroll
event. This event is fired every time the user scrolls the page. window.scrollY
property can get the current scroll position. In this code, we add a .show
to the class of .back-to-top
so that it appears at the top of the screen when the user scrolls down more than 500px. If the user scrolls up, the link's display will be hidden.
6. Summary
JavaScript is a flexible language and one of the valuable tools for web developers. By learning the tips and examples mentioned in this article, you can take advantage of Javascript and add more interactive links to make the user experience smoother and more natural. For readability and maintainability, you may want to put a lot of your JavaScript code into a separate file and then introduce it in your HTML via the script
tag.
In actual development, we can also use more JavaScript technologies to finely control links, such as template text, dynamic styles, link preloading, etc. If needed, you can find more related techniques in the JavaScript documentation.
The above is the detailed content of Set javascript link. For more information, please follow other related articles on the PHP Chinese website!

The advantages of React are its flexibility and efficiency, which are reflected in: 1) Component-based design improves code reusability; 2) Virtual DOM technology optimizes performance, especially when handling large amounts of data updates; 3) The rich ecosystem provides a large number of third-party libraries and tools. By understanding how React works and uses examples, you can master its core concepts and best practices to build an efficient, maintainable user interface.

React is a JavaScript library for building user interfaces, suitable for large and complex applications. 1. The core of React is componentization and virtual DOM, which improves UI rendering performance. 2. Compared with Vue, React is more flexible but has a steep learning curve, which is suitable for large projects. 3. Compared with Angular, React is lighter, dependent on the community ecology, and suitable for projects that require flexibility.

React operates in HTML via virtual DOM. 1) React uses JSX syntax to write HTML-like structures. 2) Virtual DOM management UI update, efficient rendering through Diffing algorithm. 3) Use ReactDOM.render() to render the component to the real DOM. 4) Optimization and best practices include using React.memo and component splitting to improve performance and maintainability.

React is widely used in e-commerce, social media and data visualization. 1) E-commerce platforms use React to build shopping cart components, use useState to manage state, onClick to process events, and map function to render lists. 2) Social media applications interact with the API through useEffect to display dynamic content. 3) Data visualization uses react-chartjs-2 library to render charts, and component design is easy to embed applications.

Best practices for React front-end architecture include: 1. Component design and reuse: design a single responsibility, easy to understand and test components to achieve high reuse. 2. State management: Use useState, useReducer, ContextAPI or Redux/MobX to manage state to avoid excessive complexity. 3. Performance optimization: Optimize performance through React.memo, useCallback, useMemo and other methods to find the balance point. 4. Code organization and modularity: Organize code according to functional modules to improve manageability and maintainability. 5. Testing and Quality Assurance: Testing with Jest and ReactTestingLibrary to ensure the quality and reliability of the code

To integrate React into HTML, follow these steps: 1. Introduce React and ReactDOM in HTML files. 2. Define a React component. 3. Render the component into HTML elements using ReactDOM. Through these steps, static HTML pages can be transformed into dynamic, interactive experiences.

React’s popularity includes its performance optimization, component reuse and a rich ecosystem. 1. Performance optimization achieves efficient updates through virtual DOM and diffing mechanisms. 2. Component Reuse Reduces duplicate code by reusable components. 3. Rich ecosystem and one-way data flow enhance the development experience.

React is the tool of choice for building dynamic and interactive user interfaces. 1) Componentization and JSX make UI splitting and reusing simple. 2) State management is implemented through the useState hook to trigger UI updates. 3) The event processing mechanism responds to user interaction and improves user experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
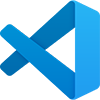
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
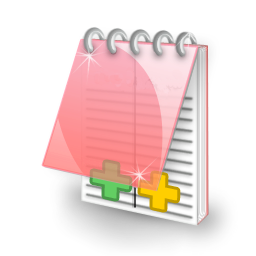
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function