In the Go language, nested structures are a very common technique. By embedding one structure within another, we can break down a complex data model into smaller parts, making it easier to understand and maintain. This article will introduce how to use nested structures in Go and some best practices.
1. Define nested structures
First, we need to define a structure that contains nested structures. The following code demonstrates how to define a Company structure that contains a Person structure:
type Person struct { Name string Age int } type Company struct { Name string Address string CEO Person }
In this example, we create a Person structure to represent the CEO of each company, and then we embed it in in the Company structure. In this way, we can access the CEO's fields just like a normal structure.
2. Initialize the nested structure
Next, we need to initialize the nested structure. We can use structure literals to initialize nested structures. The code is as follows:
company := Company{ Name: "ABC Inc.", Address: "123 Main St.", CEO: Person{ Name: "John Smith", Age: 45, }, }
In this example, we initialize a Company structure through literals. Note that we use the Person structure literal in the CEO field to initialize it.
3. Access fields of nested structures
Now we have defined and initialized the nested structure. We can access the fields of nested structures just like ordinary structures. The following shows how to access the name and age fields of the CEO:
fmt.Println(company.CEO.Name) // 输出 “John Smith” fmt.Println(company.CEO.Age) // 输出 “45”
In this example, we use the dot operator to access the Name and Age fields in the CEO structure. This is just like accessing a normal structure.
4. Inheritance and overwriting
In some scenarios, we may need to inherit and override in nested structures.
Inheritance
If two structures in a nested structure have the same fields, the nested structure will overwrite the fields in the embedded structure. If the nested structure contains additional fields, they will be added to the embedded structure. Here is an example:
type Animal struct { Name string Age int } type Bird struct { Animal // 继承Animal结构体 CanFly bool } bird := Bird{ Animal: Animal{ Name: "Polly", Age: 2, }, CanFly: true, } fmt.Println(bird.Name) // 输出 “Polly” fmt.Println(bird.Age) // 输出 “2” fmt.Println(bird.CanFly) // 输出 “true”
In this example, the Bird structure embeds the Animal structure, so we can access the fields of the Animal structure just like we access the Bird structure. When initializing the Bird structure, we initialize it using the Animal structure literal. Since the Bird structure inherits the Animal structure, it can naturally inherit the name and age fields of the Animal structure.
Override
If you need to override some fields or methods in a nested structure, we can overload in the same way as a normal structure. The following is an example:
type Person struct { Name string Age int } type Employee struct { Person // 继承Person结构体 EmployeeID string } func (p Person) Greet() { fmt.Println("Hello") } func (e Employee) Greet() { fmt.Println("Hi, I'm an employee") } employee := Employee{ Person: Person{ Name: "John Smith", Age: 35, }, EmployeeID: "12345", } employee.Person.Greet() // 输出 “Hello” employee.Greet() // 输出 “Hi, I'm an employee”
In this example, we define a Person structure and an Employee structure. The Employee structure inherits from the Person structure. We defined a Greet method in the Person structure, which prints "Hello". In the Employee structure, we overloaded the Greet method, which prints "Hi, I'm an employee". After we initialize the employee variable using the Employee structure, we can call the Greet method of the Person structure to print "Hello", or call the Greet method of the Employee structure to print "Hi, I'm an employee".
5. Nested interfaces and pointers
When using nested structures, we can also nest interfaces and pointers. The following is an example:
type Talker interface { Talk() } type Person struct { Name string Age int } func (p *Person) Talk() { fmt.Println("Hi, I'm " + p.Name) } type Employee struct { *Person // 嵌套指针类型 EmployeeID string Role string } func (e *Employee) Talk() { fmt.Println("Hi, I'm" + e.Name + ", and I work as a " + e.Role) } employee := Employee{ Person: &Person{ Name: "John Smith", Age: 35, }, EmployeeID: "12345", Role: "software engineer", } var talker Talker // 声明一个接口类型的变量 talker = &employee // 将employee赋值给talker talker.Talk() // 输出 “Hi, I'm John Smith, and I work as a software engineer”
In this example, we define a Talker interface, which has a Talk method. We defined a Person structure and an Employee structure, both of which implement the Talker interface. The Employee structure has a Person pointer nested inside it, so we can use the Talk method of the Person structure. After initializing the employee variable, we assign it to a variable of the Talker interface type, so that we can use the interface type method to access the employee variable.
6. Conclusion
In the Go language, nested structure is a very useful technology that can decompose complex data models into smaller parts. When using nested structures, we need to pay attention to the use of inheritance and override, as well as the use of nested interfaces and pointers. By mastering these technologies, we can better develop and maintain Go language projects.
The above is the detailed content of How to use nested structures in Go?. For more information, please follow other related articles on the PHP Chinese website!
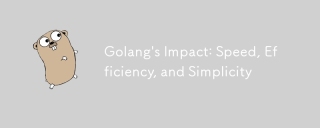
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
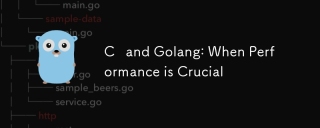
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
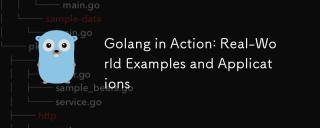
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
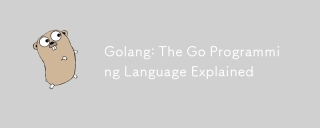
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
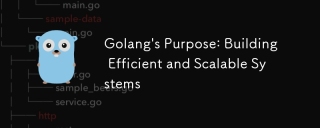
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
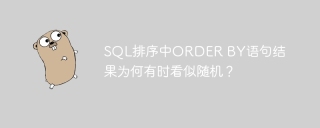
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
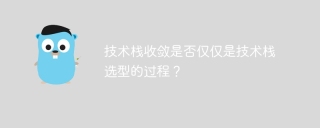
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
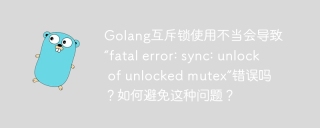
Golang ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
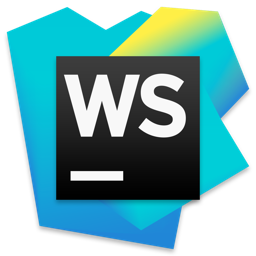
WebStorm Mac version
Useful JavaScript development tools
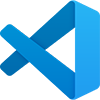
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool