With the continuous development of Web development technology, front-end and back-end separation and modular development have become a widespread trend. PHP is a commonly used back-end language. When doing modular development, we need to use some tools to manage and package modules. Webpack is a very easy-to-use modular packaging tool. This article will introduce how to use PHP and webpack for modular development.
1. What is modular development
Modular development refers to decomposing a program into different independent modules. Each module has its own scope and dependencies. These modules can be developed independently. , test, deploy, and then combine into a complete program. This separation not only improves code reusability and readability, but also makes the project easier to maintain and upgrade.
2. Installation and configuration of webpack
Webpack is a Node.js module packaging tool that can package various types of files into one or more files. We can install webpack through npm:
npm install webpack webpack-cli --save-dev
After the installation is complete, we need to perform some basic configuration. The webpack configuration file is named webpack.config.js, and it should be placed in the root directory of the project. The following is a simple webpack.config.js configuration file:
const path = require('path'); module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist') } };
The above configuration file specifies that the main entry file is src/index.js, and the output file is dist/bundle.js, in which the path.resolve method Used to resolve paths. This configuration file also needs to specify how to handle different types of files, such as CSS files, image files, HTML files, etc. These files need to be processed by the corresponding loader. You can specify the usage rules of the loader through module.rules. For example:
module.exports = { // ... module: { rules: [ { test: /.css$/, use: ['style-loader', 'css-loader'] }, { test: /.(png|svg|jpg|gif)$/, use: ['file-loader'] }, { test: /.html$/, use: ['html-loader'] } ] } };
The above code means that when webpack encounters a file ending with .css, it will use css first. -loader to parse CSS files, and then use style-loader to apply CSS styles to HTML. When an image file is encountered, use file-loader to convert the image file into a file name and output it to the dist directory. When encountering a file ending in .html, use html-loader to parse the HTML file.
3. How to use webpack in PHP
There are two methods to choose from when using webpack in PHP. The first is to package all the content of Webpack and link it into the PHP file. The second is to integrate Webpack's workflow into PHP to realize automated construction of Webpack.
- Package Webapck and introduce it into the PHP file
This method is the simplest. Reference the packaged JavaScript and CSS files in HTML, and then reference the HTML files in PHP through include or require. For example:
include 'dist/index.html';
The disadvantage of this method is that every time you modify a JS or CSS file, you need to re-execute webpack packaging and copy the files in the dist directory to the PHP web directory to see the update. Effect.
- Integrate Webpack's workflow into PHP
This method is to integrate Webpack's workflow into PHP, so that after modifying the JS or CSS file, it will automatically Packaging and output. This requires the help of some plug-ins or libraries, such as webpack-dev-server, webpack-merge, etc.
webpack-dev-server is a webpack development server that provides real-time reloading. It implements a multiplexing server and WebSocket server based on Node.js and Express, which can monitor file changes and refresh the browser in real time.
webpack-merge is a simple tool library for merging and selecting configurations. When we need to deal with different environments (such as development environment and production environment), using webpack-merge can easily merge different configurations.
The following is an example of a webpack.config.js file using webpack-dev-server and webpack-merge, which can achieve real-time packaging and output:
const path = require('path'); const webpackMerge = require('webpack-merge'); const commonConfig = require('./webpack.common'); module.exports = webpackMerge(commonConfig, { mode: 'development', output: { filename: '[name].js' }, devServer: { contentBase: path.join(__dirname, 'dist'), compress: true, port: 9000 } });
Starting the Webpack server in PHP is usually Execute the webpack-dev-server startup command through the shell_exec or exec method. For example:
shell_exec('webpack-dev-server --mode development --port 9000');
A Socket.io server with port 9000 and mode development is started here.
4. Summary
This article introduces how to use PHP and webpack for modular development. By using webpack, we can manage our modules more conveniently and improve code reusability and maintainability. At the same time, we can also integrate PHP and webpack to realize automated packaging and output, thus further simplifying our development process.
The above is the detailed content of How to use PHP and webpack for modular development. For more information, please follow other related articles on the PHP Chinese website!
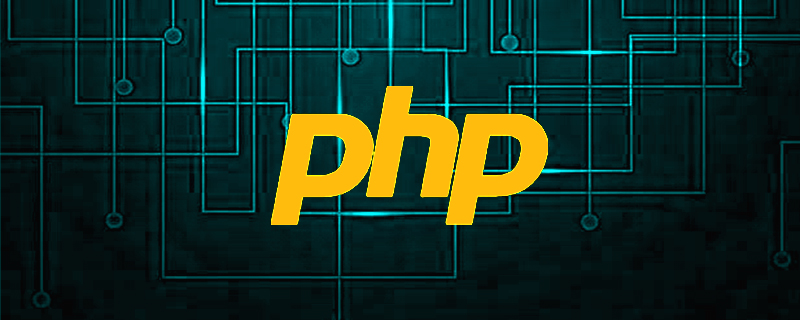
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
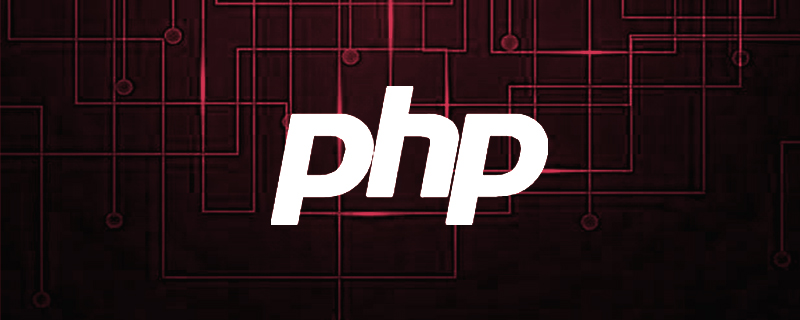
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
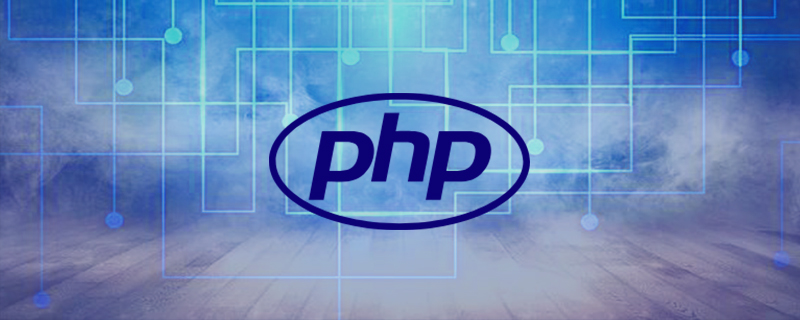
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
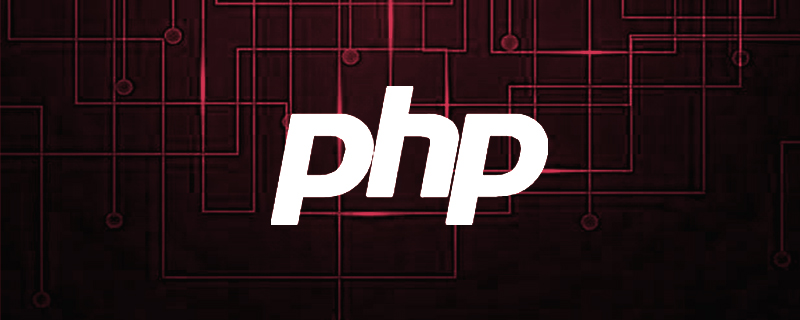
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
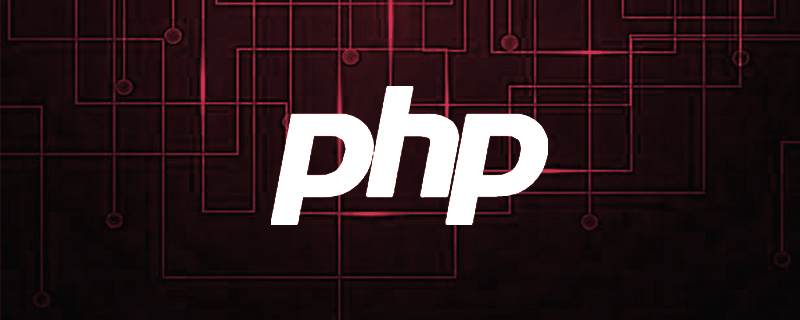
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
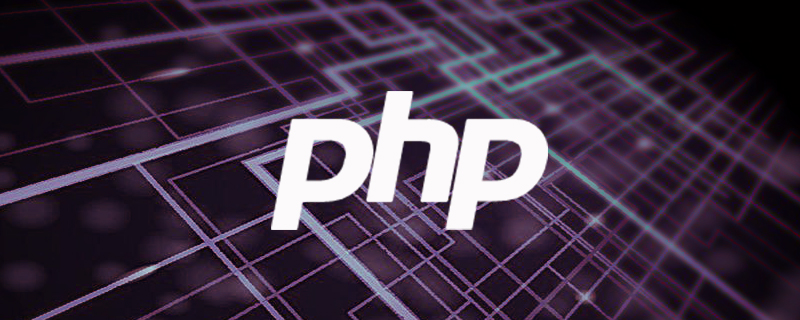
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
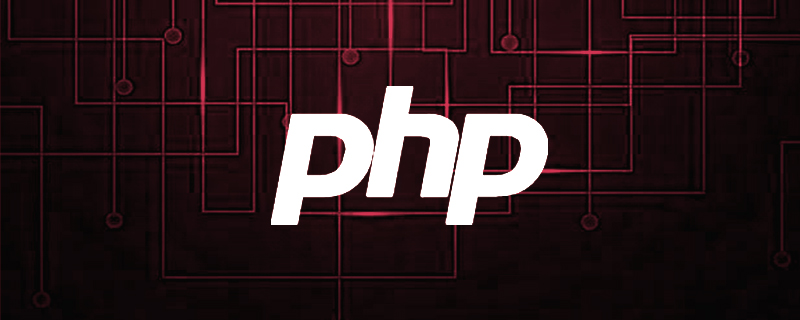
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
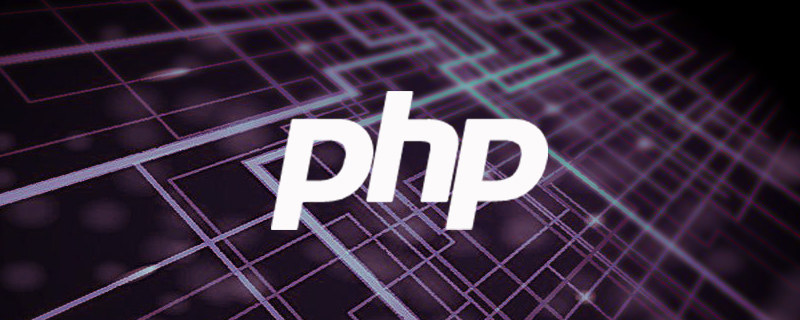
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
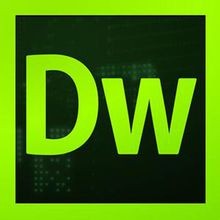
Dreamweaver CS6
Visual web development tools
