Go language is a strongly typed, statically typed, compiled language. It is widely used in network programming, server development, system programming and other fields. Using binary files in Go language is a very common task. This article will detail how to use binary files in Go.
1. What is a binary file?
In a computer, a binary file is a file that the machine can directly execute. It contains all program code and data needed at run time. Binaries are typically produced by a compiler's process of compiling source code into machine executable code. In Unix/Linux systems, the suffix of binary files is usually ".bin" or ".elf".
There are two main types of binary files involved in the Go language: executable files and library files. An executable file is a binary file that can be directly executed. Common executable files include the executable file of the Go program (suffix ".exe" or no suffix), the executable file of the C program (suffix ".out"), etc. wait. A library file is a binary file containing functions and data that can be linked to other programs. The suffix of library files is usually ".a" (static library) or ".so" (dynamic library).
2. Generate executable file
- Write source code
First, we need to write Go language source code, such as the following simple program, It outputs "Hello, world!":
package main import "fmt" func main() { fmt.Println("Hello, world!") }
Save the above code to the file "main.go".
- Compile the executable file
Use the Go language compiler to compile the source code and generate the executable file. Execute the following command:
go build main.go
After execution, an executable file named "main" will be generated. We can run this file in the command line window:
./main
After running, "Hello, world!" will be output to the terminal.
- Cross-compilation
If we need to run our program on different operating systems or architectures, we can use cross-compilation to generate executable files on different platforms . For example, we can compile a binary file on Mac that can run on Linux:
GOOS=linux GOARCH=amd64 go build main.go
After execution, an executable file named "main" will be generated in the current directory, which can run on Linux .
3. Generate library files
- Write source code
First, we need to write Go language source code, such as the following simple library file, It defines a function Add that adds two integers and returns:
package mylib func Add(a, b int) int { return a + b }
Save the above code to the file "mylib.go".
- Compile library file
Use the Go language compiler to compile the source code and generate the library file. Execute the following command:
go build -o mylib.a -buildmode=c-archive mylib.go
After execution, a static library file named "mylib.a" will be generated in the current directory.
- Using library files
It is very easy to use library files in Go language. You only need to import the library file in the source code file that needs to use the library function. For example, in the following program, we import the "mylib" library file and call the Add function in it:
package main import ( "fmt" "mylib" ) func main() { sum := mylib.Add(1, 2) fmt.Println(sum) }
Execute the following command to compile the program:
go build main.go
After execution, Output "3" to the terminal.
4. Reading binary files
Go language provides some powerful APIs for reading binary files, such as os.Open function, io.ReadFull function, etc. These APIs allow us to read data from binary files and convert them into variables in Go language.
The following is an example, which opens a binary file named "test.bin" and reads an integer in it:
package main import ( "encoding/binary" "fmt" "os" ) func main() { // 打开二进制文件 file, err := os.Open("test.bin") if err != nil { fmt.Println(err) return } defer file.Close() // 读取一个int32类型的整数 var num int32 err = binary.Read(file, binary.LittleEndian, &num) if err != nil { fmt.Println(err) return } fmt.Println("Num is", num) }
Execute the above code, it will read an integer Be an integer in the binary file "test.bin" and print it to the terminal.
5. Summary
Using binary files is one of the basic tasks in the Go language. In this article, we covered how to generate executables and libraries in Go, and use libraries. At the same time, we also learned how to read data from binary files. These skills are all necessary to write truly useful applications. We can further extend and customize these features through advanced concepts of the Go language such as addresses and pipes. Therefore, it is very useful to master the skills of working with binaries in Go language.
The above is the detailed content of How to use binaries in Go?. For more information, please follow other related articles on the PHP Chinese website!
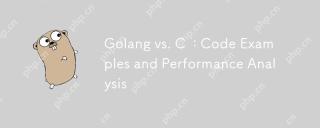
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
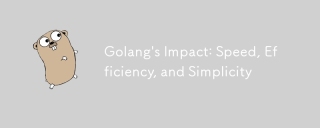
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
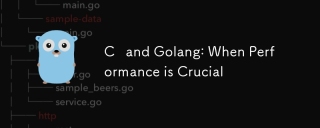
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
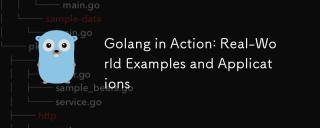
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
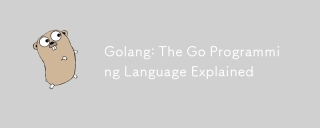
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
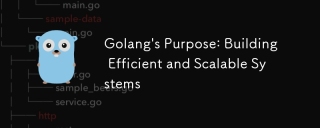
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
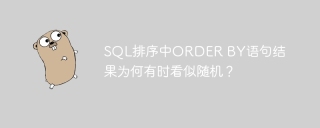
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
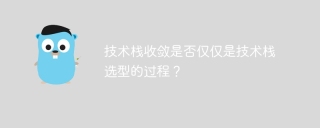
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.