Foreword
Scenario
The time parameters passed from the front end can be converted into whatever custom time format we want on the back end.
In different business scenarios, when connected with the front end, the basic time parameters of a control are in a fixed format. In order to prevent the front end from converting the format of the time parameters, we made an agreement with the front end and asked them to pass a fixed format to the back end. You can convert the format according to your needs.
Effect
① Convert from yyyy-MM-dd HH:mm:ss to yyyy-MM-dd using:
② To convert from yyyyMMddHHmmss to yyyy-MM-dd HH:mm:ss use:
③ No more examples, in fact, you can convert it however you want.
Actual combat
pom.xml (aop dependency, lombok dependency):
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.18.20</version> <scope>compile</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aspects</artifactId> <version>5.2.7.RELEASE</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjtools</artifactId> <version>1.9.5</version> </dependency> <dependency> <groupId>aopalliance</groupId> <artifactId>aopalliance</artifactId> <version>1.0</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.9.0</version> </dependency> <dependency> <groupId>cglib</groupId> <artifactId>cglib</artifactId> <version>3.3.0</version> </dependency>
Core (custom annotation interceptor):
Custom Annotation 1
DateField.java
Purpose: Used to mark which field needs time format conversion, configure old format, new format (default value can be written) .
import java.lang.annotation.ElementType; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; /** * @Author: JCccc * @Date: 2022-4-11 18:45 * @Description: */ @Target({ElementType.METHOD, ElementType.FIELD}) @Retention(RetentionPolicy.RUNTIME) public @interface DateField { String oldPattern() default DateUtil.YYYY_MM_DD_HH_MM_SS; //新格式可以写默认也可以不写,如果业务比较固定,那么新时间格式和旧时间格式都可以固定写好 String newPattern() default ""; }
Custom Annotation 2
NeedDateFormatConvert.java
Purpose: Used to mark which interface requires AOP time format conversion.
import java.lang.annotation.ElementType; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; /** * @Author: JCccc * @Date: 2022-4-11 18:44 * @Description: */ @Target({ElementType.METHOD}) @Retention(RetentionPolicy.RUNTIME) public @interface NeedDateFormatConvert { }
Interceptor
DateFormatAspect.java
Purpose: Core conversion implementation logic.
import com.jctest.dotestdemo.util.DateUtil; import org.aspectj.lang.ProceedingJoinPoint; import org.aspectj.lang.annotation.Around; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Pointcut; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.stereotype.Component; import org.springframework.util.StringUtils; import java.lang.reflect.Field; import java.util.Objects; /** * @Author: JCccc * @Date: 2022-4-11 18:57 * @Description: */ @Aspect @Component public class DateFormatAspect { private static Logger log = LoggerFactory.getLogger(DateFormatAspect.class); @Pointcut("@annotation(com.jctest.dotestdemo.aop.dateFormat.NeedDateFormatConvert)") public void pointCut() { } @Around("pointCut()") public Object around(ProceedingJoinPoint joinPoint) throws Throwable { //转换 dateFormat(joinPoint); return joinPoint.proceed(); } public void dateFormat(ProceedingJoinPoint joinPoint) { Object[] objects = null; try { objects = joinPoint.getArgs(); if (objects.length != 0) { for (int i = 0; i < objects.length; i++) { //当前只支持判断对象类型参数 convertObject(objects[i]); } } } catch (Exception e) { e.printStackTrace(); throw new RuntimeException("参数异常"); } } /** * 转换对象里面的值 * * @param obj * @throws IllegalAccessException */ private void convertObject(Object obj) throws IllegalAccessException { if (Objects.isNull(obj)) { log.info("当前需要转换的object为null"); return; } Field[] fields = obj.getClass().getDeclaredFields(); for (Field field : fields) { boolean containFormatField = field.isAnnotationPresent(DateField.class); if (containFormatField) { //获取访问权 field.setAccessible(true); DateField annotation = field.getAnnotation(DateField.class); String oldPattern = annotation.oldPattern(); String newPattern = annotation.newPattern(); Object dateValue = field.get(obj); if (Objects.nonNull(dateValue) && StringUtils.hasLength(oldPattern) && StringUtils.hasLength(newPattern)) { String newDateValue = DateUtil.strFormatConvert(String.valueOf(dateValue), oldPattern, newPattern); if (Objects.isNull(newDateValue)){ log.info("当前需要转换的日期数据转换失败 dateValue = {}",dateValue.toString()); throw new RuntimeException("参数转换异常"); } field.set(obj, newDateValue); } } } } }
Tool Class
DateUtil.java
Purpose: Time format conversion function, defining various time formats.
import lombok.extern.slf4j.Slf4j; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; /** * @Author: JCccc * @Date: 2022-4-1 14:48 * @Description: */ @Slf4j public class DateUtil { public static final String YYYY_MM_DD_HH_MM_SS = "yyyy-MM-dd HH:mm:ss"; public static final String YYYY_MM_DD = "yyyy-MM-dd"; public static final String YYYY_MM = "yyyy-MM"; public static final String YYYY = "yyyy"; public static final String MM = "MM"; public static final String DD = "dd"; public static final String YYYYMMDDHHMMSS = "yyyyMMddHHmmss"; public static final String YYYYMMDD = "yyyyMMdd"; /** * 指定日期格式转换 * * @param dateStr * @param oldPattern * @return */ public static String strFormatConvert(String dateStr, String oldPattern,String newPattern) { try { DateTimeFormatter oldFormatter = DateTimeFormatter.ofPattern(oldPattern); DateTimeFormatter newFormatter = DateTimeFormatter.ofPattern(newPattern); return LocalDateTime.parse(dateStr, oldFormatter).format(newFormatter); } catch (Exception e) { log.error("strToDate is Exception. e:", e); return null; } } }
Use
UserQueryVO.java
import com.jctest.dotestdemo.aop.dateFormat.DateField; import com.jctest.dotestdemo.util.DateUtil; import lombok.Data; import java.io.Serializable; /** * @Author: JCccc * @Date: 2022-4-1 14:48 * @Description: */ @Data public class UserQueryVO implements Serializable { /** * 开始时间 */ @DateField(oldPattern =DateUtil.YYYY_MM_DD_HH_MM_SS, newPattern = DateUtil.YYYY_MM_DD) private String startDate; /** * 结束时间 */ @DateField(oldPattern =DateUtil.YYYY_MM_DD_HH_MM_SS,newPattern = DateUtil.YYYY_MM_DD) private String endDate; }
Interface
import com.jctest.dotestdemo.aop.dateFormat.NeedDateFormatConvert; import com.jctest.dotestdemo.vo.UserQueryVO; import org.springframework.web.bind.annotation.*; /** * @Author: JCccc * @Date: 2022-4-18 11:52 * @Description: */ @RestController public class UserController { @NeedDateFormatConvert @PostMapping("/test") public String test( @RequestBody UserQueryVO userQueryVO){ System.out.println("时间格式转化完成:"); System.out.println(userQueryVO.getStartDate()); System.out.println(userQueryVO.getEndDate()); return userQueryVO.toString(); } }
Call
The above is the detailed content of How Springboot+AOP implements time parameter format conversion. For more information, please follow other related articles on the PHP Chinese website!
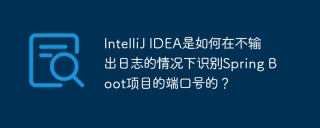
Start Spring using IntelliJIDEAUltimate version...
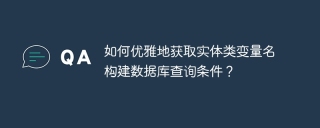
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
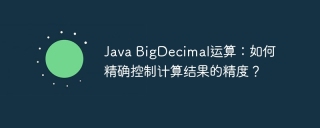
Java...
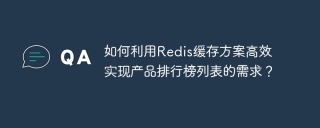
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
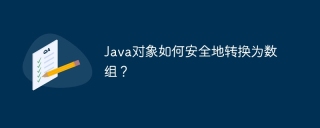
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
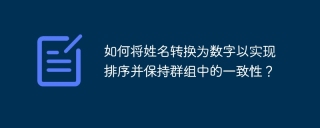
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
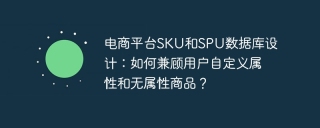
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
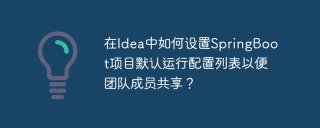
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
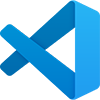
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software