How to set fields using reflection in Go?
Go language is a strongly typed static programming language, in which reflection is a very powerful tool. Using reflection you can dynamically call functions and access variables, making your code more flexible and reusable. In the Go language, reflection can be used to operate the fields of a structure, which allows us to modify the values of the fields at runtime.
This article will focus on how to use reflection to set fields in the Go language. We'll first learn the basics of reflection and then go through a simple code example to illustrate how to use reflection in Go to set the fields of a structure.
Basic knowledge of reflection
In the Go language, reflection is a mechanism that can check the type and value of an object while the program is running. Reflection allows us to check the type of a variable, as well as the value and structure of the value it holds. Reflection also allows us to dynamically manipulate variables at runtime.
Reflection in Go language mainly depends on the reflect package. Through the reflect package, we can obtain type information, methods, fields and values of structures. You need to follow the following steps to use the reflection package:
- Use the reflect.TypeOf() function to get the type of a value.
- Use the reflect.ValueOf() function to get the reflection value of a value.
- Use reflection values to perform operations, such as getting or setting its fields, methods, etc.
Reflection operation of structure
In Go language, structure is a user-defined complex data type, which consists of multiple fields. Using reflection allows us to dynamically modify the field values of a structure at runtime. We will use the following example to introduce how to use reflection to set the fields of a structure in Go.
Sample code:
package main import ( "fmt" "reflect" ) type Person struct { Name string Age int Salary float64 } func main() { person := Person{Name: "Alice", Age: 22, Salary: 5000.0} // 使用ValueOf获取person的反射值 value := reflect.ValueOf(&person).Elem() // 获取Name字段 field := value.FieldByName("Name") if field.IsValid() { if !field.CanSet() { fmt.Println("Name字段不可修改") } else { field.SetString("Bob") fmt.Println("Name字段已修改为:", person.Name) } } else { fmt.Println("Name字段不存在") } // 获取Age字段 field = value.FieldByName("Age") if field.IsValid() { if !field.CanSet() { fmt.Println("Age字段不可修改") } else { field.SetInt(25) fmt.Println("Age字段已修改为:", person.Age) } } else { fmt.Println("Age字段不存在") } // 获取Salary字段 field = value.FieldByName("Salary") if field.IsValid() { if !field.CanSet() { fmt.Println("Salary字段不可修改") } else { field.SetFloat(6000.0) fmt.Println("Salary字段已修改为:", person.Salary) } } else { fmt.Println("Salary字段不存在") } }
The above example code defines a Person structure and creates a Person type variable in the main function. We will modify it below.
First, we use the reflect.ValueOf() function to get the reflection value of the person variable. Then, obtain the value of the structure field through the FieldByName() method provided by the Value structure. If the field exists, determine whether it can be modified, and modify its value through the SetString(), SetInt() or SetFloat() method. Finally, we will print the modification results of the field.
Summary
This article introduces how to use reflection to set structure field values in the Go language. Reflection is a very powerful tool that can greatly improve programming flexibility and reusability. By studying the sample code provided in this article, I believe you have learned how to use reflection to set structure field values in the Go language.
The above is the detailed content of How to set fields using reflection in Go?. For more information, please follow other related articles on the PHP Chinese website!
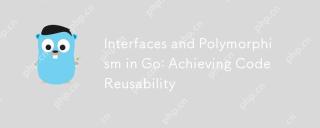
InterfacesandpolymorphisminGoenhancecodereusabilityandmaintainability.1)Defineinterfacesattherightabstractionlevel.2)Useinterfacesfordependencyinjection.3)Profilecodetomanageperformanceimpacts.
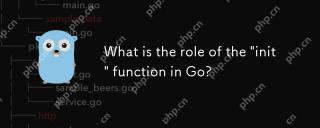
TheinitfunctioninGorunsautomaticallybeforethemainfunctiontoinitializepackagesandsetuptheenvironment.It'susefulforsettingupglobalvariables,resources,andperformingone-timesetuptasksacrossanypackage.Here'showitworks:1)Itcanbeusedinanypackage,notjusttheo
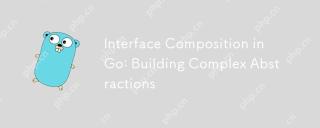
Interface combinations build complex abstractions in Go programming by breaking down functions into small, focused interfaces. 1) Define Reader, Writer and Closer interfaces. 2) Create complex types such as File and NetworkStream by combining these interfaces. 3) Use ProcessData function to show how to handle these combined interfaces. This approach enhances code flexibility, testability, and reusability, but care should be taken to avoid excessive fragmentation and combinatorial complexity.
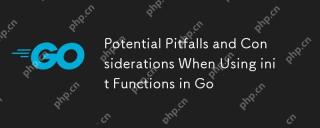
InitfunctionsinGoareautomaticallycalledbeforethemainfunctionandareusefulforsetupbutcomewithchallenges.1)Executionorder:Multipleinitfunctionsrunindefinitionorder,whichcancauseissuesiftheydependoneachother.2)Testing:Initfunctionsmayinterferewithtests,b
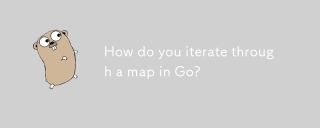
Article discusses iterating through maps in Go, focusing on safe practices, modifying entries, and performance considerations for large maps.Main issue: Ensuring safe and efficient map iteration in Go, especially in concurrent environments and with l
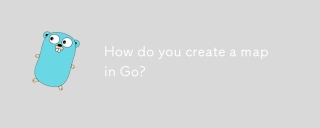
The article discusses creating and manipulating maps in Go, including initialization methods and adding/updating elements.
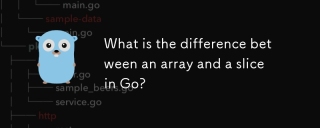
The article discusses differences between arrays and slices in Go, focusing on size, memory allocation, function passing, and usage scenarios. Arrays are fixed-size, stack-allocated, while slices are dynamic, often heap-allocated, and more flexible.
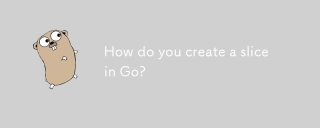
The article discusses creating and initializing slices in Go, including using literals, the make function, and slicing existing arrays or slices. It also covers slice syntax and determining slice length and capacity.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
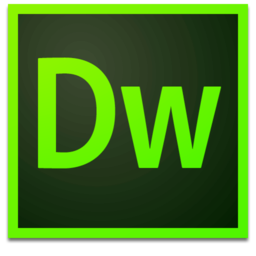
Dreamweaver Mac version
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
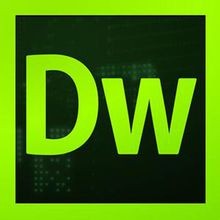
Dreamweaver CS6
Visual web development tools
