Go is a strongly typed, statically typed programming language. It was created by Google to provide an efficient and simple syntax while being able to support concurrent programming.
In Go, there are many basic operators that can be used to perform various different operations. Understanding how to use these operators is important to writing efficient code. In this article, we will discuss using common basic operators in Go.
Arithmetic operators
In Go, arithmetic operators are used to perform basic arithmetic operations. These operators include:
• Addition operator ( )
• Subtraction operator (-)
• Multiplication operator (*)
• Division operator (/)
• Remainder operator (%)
Here are some sample codes:
package main
import "fmt"
func main() {
var a int = 10 var b int = 20 fmt.Println(a + b) fmt.Println(a - b) fmt.Println(a * b) fmt.Println(b / a) fmt.Println(b % a)
}
Output:
30
-10
200
2
0
Comparison operator
In Go, comparison operators are used to compare two values and return true or false. These operators include:
• Equals operator (==)
• Not equal to operator (!=)
• Greater than operator (>)
• Less than operator ( • Greater than or equal to operator (>=)
• Less than or equal to operator (
Here is some sample code:
package main
import "fmt"
func main() {
var a int = 10 var b int = 20 fmt.Println(a == b) fmt.Println(a != b) fmt.Println(a > b) fmt.Println(a < b) fmt.Println(a >= b) fmt.Println(a <= b)
}
Output:
false
true
false
true
false
true
Logical operators
In Go, logical operators are used to combine two or more conditions together. These operators include:
• Logical AND operator (&&)
• Logical OR operator (||)
• Logical NOT operator (!)
The following are Some sample code:
package main
import "fmt"
func main() {
var a int = 10 var b int = 20 var c int = 30 fmt.Println((a < b) && (b < c)) fmt.Println((a < b) || (b > c)) fmt.Println(!(a < b))
}
Output:
true
true
false
Bit operators
In Go, bit operators are used to perform binary bit operations. These operators include:
• Bitwise AND operator (&)
• Bitwise OR operator (|)
• Bitwise XOR operator (^)
• Left Shift operator (• Right shift operator (>>)
Here are some sample codes:
package main
import "fmt"
func main() {
var a uint = 60 /* 60 = 0011 1100 */ var b uint = 13 /* 13 = 0000 1101 */ fmt.Println(a & b) /* 0000 1100 */ fmt.Println(a | b) /* 0011 1101 */ fmt.Println(a ^ b) /* 0011 0001 */ fmt.Println(a << 2) /* 1111 0000 */ fmt.Println(a >> 2) /* 0000 1111 */
}
Output:
12
61
49
240
15
Assignment Operator
In Go, the assignment operator is used to assign a value to another variable. These operators include:
• = assignment
• = addition and assignment
• -= subtraction and assignment
• *= multiplication and assignment
• /= division and assignment
• %= Remainder and assignment
• • >>= Right shift and assignment
• &= Bitwise AND and assignment
• |= Bitwise OR and assignment
• ^= Bitwise XOR and assignment
Here are some sample codes:
package main
import "fmt "
func main() {
var a int = 10 var b int = 20 a = b fmt.Println(a) a += b fmt.Println(a) a -= b fmt.Println(a) a *= b fmt.Println(a) a /= b fmt.Println(a) a %= b fmt.Println(a) a <<= 2 fmt.Println(a) a >>= 2 fmt.Println(a) a &= 3 fmt.Println(a) a |= 3 fmt.Println(a) a ^= 3 fmt.Println(a)
}
Output:
20
40
20
400
20
0
80
20
0
3
0
Summary
Go supports a variety of basic operators, including arithmetic, comparison, logic, Bitwise and assignment operators. Knowing how to use these operators can help you write efficient code. When writing code, choose the appropriate operator based on your needs.
The above is the detailed content of How to use basic operators in Go?. For more information, please follow other related articles on the PHP Chinese website!
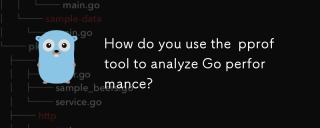
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
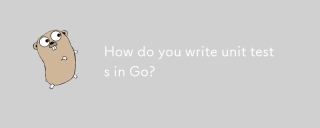
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
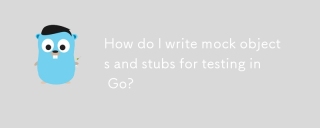
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
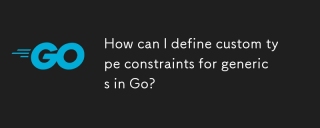
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
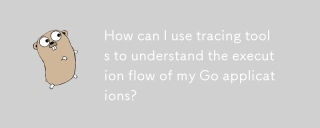
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
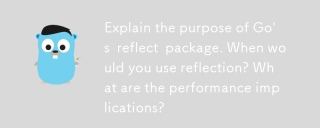
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
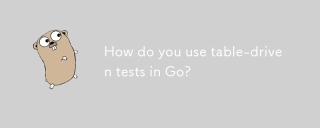
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
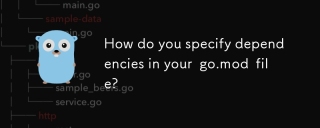
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
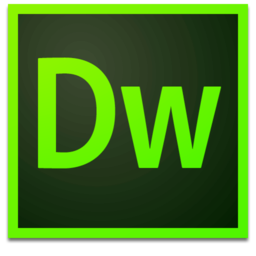
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
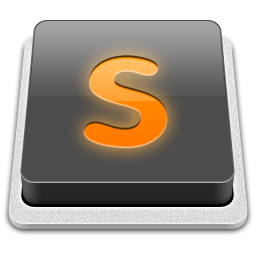
SublimeText3 Mac version
God-level code editing software (SublimeText3)
