With the development of Internet technology, distributed systems have become an important topic, and distributed locks are also one of the important technologies. In a distributed system, the order and security of access to shared resources by multiple processes or threads can be guaranteed by using distributed locks. In Java, there are many solutions to implement distributed locks, among which the Redis distributed lock solution is one of the more commonly used methods.
Redis is a high-performance, persistent, in-memory database with good data structure support and distributed characteristics. Redis cluster mode can easily expand the performance of the system, and at the same time, the distributed lock function can also be realized through the subscription mechanism based on PUB/SUB. Below we will introduce how to use Redis to implement distributed locks.
1. Design ideas of Redis lock
To implement a lock in a distributed system, the following conditions need to be met:
1. Mutual exclusion: only one lock can be locked at the same time A client holds the lock.
2. Reentrant: The same client can acquire the lock multiple times and needs to release the lock the same number of times.
3. Non-blocking: If the attempt to acquire the lock fails, it will return immediately and will not block the client thread.
4. Fault tolerance: The lock should be automatically released after it expires or expires, so as not to cause deadlock and other problems.
Based on the above conditions, we can design the following Redis lock implementation plan:
1. Use the SETNX command to try to set the value of the lock. If 1 is returned, it means the lock was successfully acquired, otherwise it means Failed to acquire lock.
2. Use the GET command to obtain the lock value and determine whether the current client holds the lock. If it holds the lock, increase the lock value by 1, otherwise it will return failure to obtain the lock.
3. Use the DEL command to release the lock.
4. Use expiration time to prevent deadlock. The expiration time of the lock should be greater than the business processing time, usually a few seconds to a few minutes.
2. Java code for implementing distributed locks
The following is an example of Java code for using Redis to implement distributed locks:
import redis.clients.jedis.Jedis; import redis.clients.jedis.JedisPool; import redis.clients.jedis.JedisPoolConfig; public class RedisLock { private static JedisPool jedisPool = null; static { JedisPoolConfig jedisPoolConfig = new JedisPoolConfig(); jedisPool = new JedisPool(jedisPoolConfig, "localhost", 6379); } /** * 获取锁 * @param key 锁的key值 * @param expireTime 锁的过期时间 * @return 获取锁的结果 */ public static boolean tryLock(String key, int expireTime) { Jedis jedis = jedisPool.getResource(); //尝试获取锁 Long result = jedis.setnx(key, "1"); if (result == 1) { //设置过期时间 jedis.expire(key, expireTime); jedis.close(); return true; } else { jedis.close(); return false; } } /** * 释放锁 * @param key 锁的key值 */ public static void releaseLock(String key) { Jedis jedis = jedisPool.getResource(); jedis.del(key); jedis.close(); } }
3. Examples of using distributed locks
The following is a Java code example using distributed locks. This example is a program that simulates high concurrency. The program will open multiple threads to operate shared resources at the same time.
public class ConcurrentTest { private static int count = 0; public static void main(String[] args) throws InterruptedException { ExecutorService executorService = Executors.newFixedThreadPool(10); for(int i=0; i<100000; i++){ executorService.execute(() -> { String key = "lock_key"; boolean result = RedisLock.tryLock(key, 2); if(result){ try { count ++; //操作共享资源 System.out.println(Thread.currentThread().getName() + "操作成功,count=" + count); Thread.sleep(100); } catch (Exception e) { e.printStackTrace(); }finally{ RedisLock.releaseLock(key); //释放锁 } } }); } executorService.shutdown(); } }
4. Summary
In a distributed system, the role of locks is very important. Reasonable and effective use of locks can ensure the security and efficiency of the system. Redis distributed lock is a relatively common method. Through the high performance and distributed characteristics of Redis, the function of distributed lock can be easily realized. Developers can decide whether to use Redis distributed locks based on business needs and system performance requirements.
The above is the detailed content of Using Redis to implement distributed locks in Java. For more information, please follow other related articles on the PHP Chinese website!
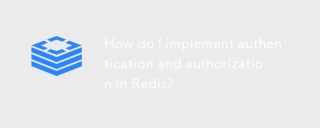
The article discusses implementing authentication and authorization in Redis, focusing on enabling authentication, using ACLs, and best practices for securing Redis. It also covers managing user permissions and tools to enhance Redis security.
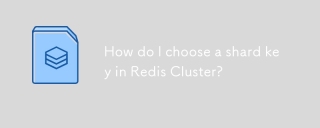
The article discusses choosing shard keys in Redis Cluster, emphasizing their impact on performance, scalability, and data distribution. Key issues include ensuring even data distribution, aligning with access patterns, and avoiding common mistakes l
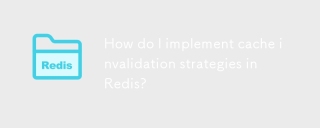
The article discusses strategies for implementing and managing cache invalidation in Redis, including time-based expiration, event-driven methods, and versioning. It also covers best practices for cache expiration and tools for monitoring and automat

The article discusses using Redis for job queues and background processing, detailing setup, job definition, and execution. It covers best practices like atomic operations and job prioritization, and explains how Redis enhances processing efficiency.
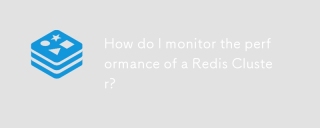
Article discusses monitoring Redis Cluster performance and health using tools like Redis CLI, Redis Insight, and third-party solutions like Datadog and Prometheus.
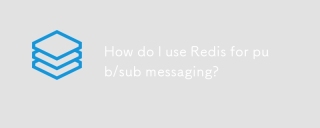
The article explains how to use Redis for pub/sub messaging, covering setup, best practices, ensuring message reliability, and monitoring performance.
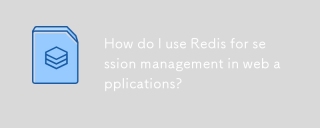
The article discusses using Redis for session management in web applications, detailing setup, benefits like scalability and performance, and security measures.

Article discusses securing Redis against vulnerabilities, focusing on strong passwords, network binding, command disabling, authentication, encryption, updates, and monitoring.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
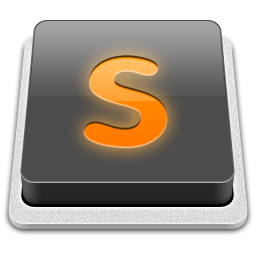
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
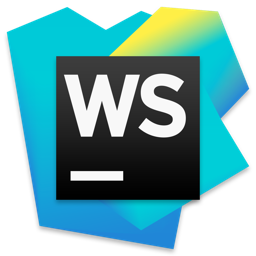
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
