PHP, as a commonly used server-side programming language, has a data type - array. Arrays are one of the most important data types in PHP and are mainly used to store and manipulate multiple values. However, when we work with an array, we often need to detect whether it is empty. In this article, we will share how to detect if an array is empty in PHP.
1. Definition of empty array
An empty array refers to an array without elements. In PHP, if an array has no elements, it is considered an empty array. For example:
$empty_arr = array();
This is an empty array because it does not have any elements. Conversely, an array is considered non-empty if it has one or more elements. For example:
$non_empty_arr = array('apple', 'banana', 'orange');
This array has 3 elements, so it is not an empty array.
2. Use the count() function to detect whether the array is empty
In PHP, we can use the count() function to detect whether the array is empty. The count() function returns the number of elements in the specified array. If there are no elements in the array, the count() function returns 0. Therefore, we can use the count() function to check if an array is empty. For example:
$empty_arr = array(); if (count($empty_arr) == 0) { echo '数组为空'; }
In this example, we first define an empty array $empty_arr. Then, we use the count() function to detect whether the array is empty. If there are no elements in the array, the count() function returns 0, so we can use a conditional statement to check whether the count() function return value is equal to 0. Here, if $empty_arr is empty, we will output "array is empty".
Similarly, we can use the count() function to detect non-empty arrays:
$non_empty_arr = array('apple', 'banana', 'orange'); if (count($non_empty_arr) > 0) { echo '数组不为空'; }
In this example, we check whether the $non_empty_arr array has elements. So if the $non_empty_arr array is not empty, we will output "array is not empty".
3. Use the empty() function to check whether the array is empty
In addition to the count() function, we can also use PHP's empty() function to check whether the array is empty. The empty() function checks whether a variable is empty or does not exist. The empty() function returns true if the variable is considered empty, false otherwise.
The empty() function works slightly differently than the count() function when checking an array. When using the empty() function to check an array, if there are no elements in the array, or the array is undefined, the empty() function returns true. Example:
$empty_arr = array(); if (empty($empty_arr)) { echo '数组为空'; }
In this example, we use the empty() function to check whether the $empty_arr array is empty. Since the $empty_arr array is empty, the empty() function returns true, so we will output "array is empty".
Similarly, we can use the empty() function to check a non-empty array:
$non_empty_arr = array('apple', 'banana', 'orange'); if (!empty($non_empty_arr)) { echo '数组不为空'; }
In this example, we use the ! operator to invert the return value of the empty() function. Here, if the $non_empty_arr array is not empty, the empty() function returns false, which becomes true after negation, and we will output "the array is not empty".
4. The isset() function detects whether the array is empty
Finally, we can also use the isset() function to detect whether an array is empty. The isset() function checks whether the variable has been set and is not null. The isset() function returns true if the variable is set, otherwise it returns false.
For arrays, we can use the isset() function to detect whether the array exists and has at least one element. Example:
$empty_arr = array(); if (!isset($empty_arr[0])) { echo '数组为空'; }
In this example, we use the isset() function to check the first element of the $empty_arr array. Since the $empty_arr array does not have any elements, we can use a conditional statement to check if the isset() function returns false. Here, if the $empty_arr array is empty, we will output "array is empty".
Similarly, we can use the isset() function to check a non-empty array:
$non_empty_arr = array('apple', 'banana', 'orange'); if (isset($non_empty_arr[0])) { echo '数组不为空'; }
In this example, we use the isset() function to check the first element of the $non_empty_arr array. Since the $non_empty_arr array has at least one element, the isset() function returns true, so we will output "array is not empty".
5. Summary
In PHP, we can use the count() function, empty() function and isset() function to check whether the array is empty. These functions are used in slightly different ways, but they all effectively detect whether an array is empty. Finally, please note that when checking an array, try to use the strict equal (===) or strict not equal (! ==) operator, as this will help you avoid precision loss problems in PHP.
The above is the detailed content of php detect if array is empty. For more information, please follow other related articles on the PHP Chinese website!
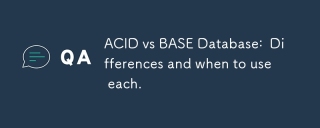
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
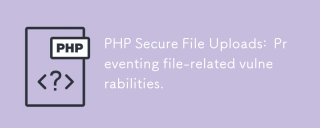
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
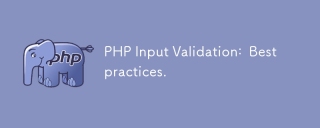
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
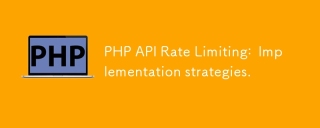
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
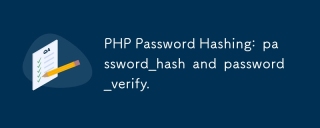
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
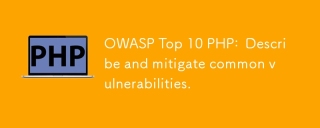
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
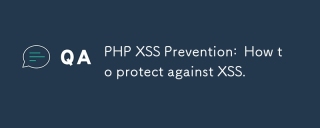
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
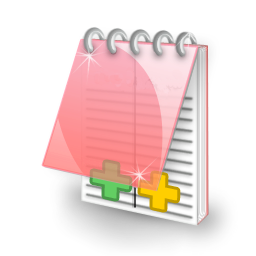
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
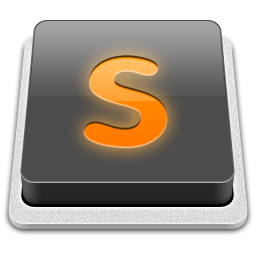
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor