Practical application of Redis in Java
With the rapid development of the Internet and information technology, a large amount of data and applications need to be stored, processed and accessed. In this context, Redis, as a high-performance, high-reliability, distributed memory database, has gradually become one of the necessary skills for Java developers. This article will introduce the actual application of Redis in Java, including the use of data structures, implementation of connection pools, cluster construction, and application scenario cases.
1. Use of data structures
Redis has a very rich data structure, including String, List, Set, Sorted Set, Hash and HyperLogLog, etc. The following describes how to use it in Java.
-
String
String is the most basic data type of Redis. You can set a Key and the corresponding Value.
Jedis jedis = new Jedis("localhost", 6379);
jedis.set("name", "Tom");
String name = jedis.get ("name");
- List
#List is an ordered collection that stores multiple elements and can be added, deleted and queried based on the index value.
jedis.lpush("list", "a", "b", "c");
jedis.rpush("list", "d", "e", " f");
List
- Set
Set is An unordered collection that does not allow duplicate elements.
jedis.sadd("set", "a", "b", "c", "d");
jedis.srem("set", "a");
Set
- Sorted Set
Sorted Set is an ordered set. Each element has a score and can be sorted based on the score.
jedis.zadd("sortedset", 5, "a");
jedis.zadd("sortedset", 10, "b");
jedis. zrem("sortedset", "a");
Set
- Hash
Hash is a key-value pair storage structure that can store multiple attributes and corresponding values.
jedis.hset("hash", "name", "Tom");
jedis.hset("hash", "age", "20");
String name = jedis.hget("hash", "name");
- HyperLogLog
HyperLogLog is a radix algorithm used to count the number of elements. This can be done without recording the original value.
jedis.pfadd("hll", "a", "b", "c");
long count = jedis.pfcount("hll");
2. Implementation of connection pool
In order to ensure high concurrency and high performance, Redis Java clients generally use connection pools to manage connections. Here we take Jedis as an example to introduce the implementation method of connection pool.
JedisPoolConfig poolConfig = new JedisPoolConfig();
poolConfig.setMaxIdle(10);
poolConfig.setMaxTotal(20);
poolConfig.setMaxWaitMillis( 1000);
JedisPool jedisPool = new JedisPool(poolConfig, "localhost", 6379);
Jedis jedis = null;
try {
jedis = jedisPool.getResource(); ...
} finally {
if (jedis != null) { jedis.close(); } jedisPool.close();
}
3. Cluster construction
When the amount of data reaches a certain scale, a single Redis instance can no longer meet the demand, and a Redis cluster needs to be built. . Redis officially provides Cluster mode for cluster construction. Multiple Redis instances are started to form a cluster to achieve high data availability and load balancing. Here is an introduction to how to build Cluster mode.
redis-cli --cluster create node1:6379 node2:6379 node3:6379
Start 3 Redis instances respectively, the port numbers are 6379, use the redis-cli command to combine them a cluster.
4. Application scenario cases
- Caching
Redis can be used as a cache to improve access speed. Storing some frequently accessed data in Redis can reduce the access pressure on the database and improve system performance.
- Distributed lock
Redis can implement distributed locks to avoid problems caused by multiple processes accessing the same resource at the same time and improve the stability and reliability of the system. .
- Counter
Redis can be used as a counter. The value of the counter can be incremented or decremented, and concurrent operations are supported.
- Queue
Redis can be used as a queue, supports producer and consumer modes, and provides multiple queue implementation methods.
Summary:
This article introduces the actual application of Redis in Java, including the use of data structures, implementation of connection pools, cluster construction, and application scenario cases. With the powerful functions of Redis and the rich library functions of Java, we can quickly build a high-performance, high-reliability distributed application system and improve the efficiency and scalability of the system.
The above is the detailed content of Practical application of Redis in Java. For more information, please follow other related articles on the PHP Chinese website!
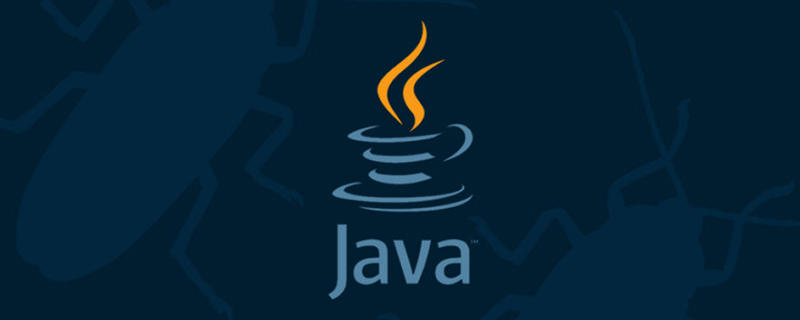
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
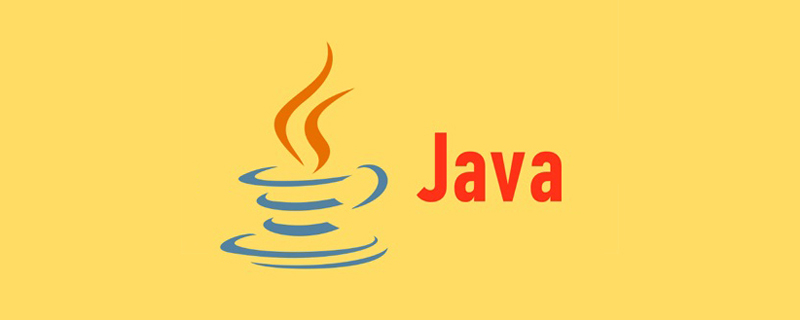
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
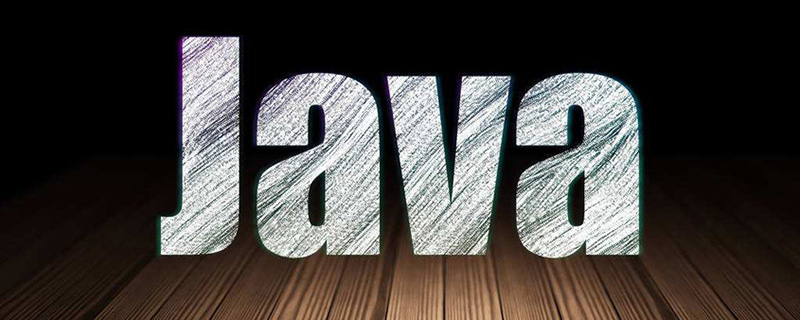
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
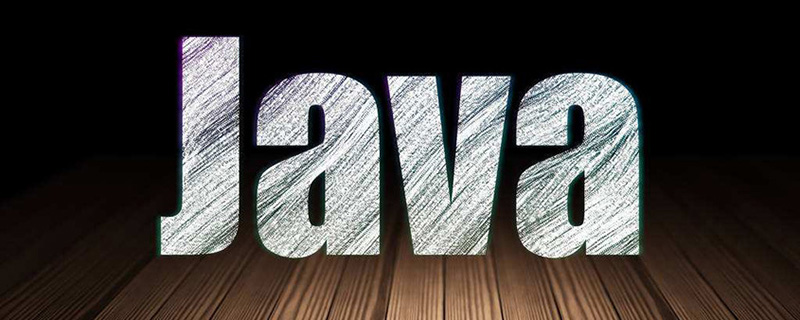
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
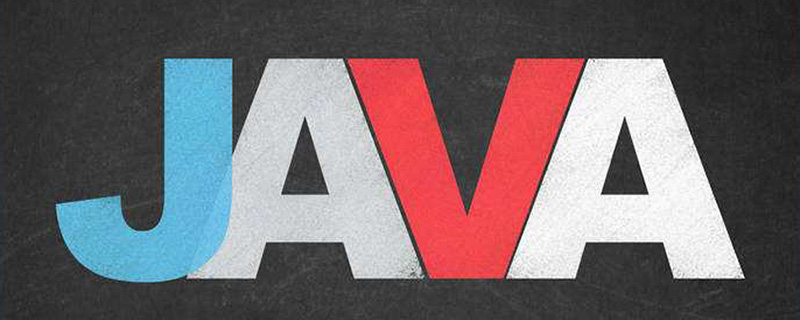
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
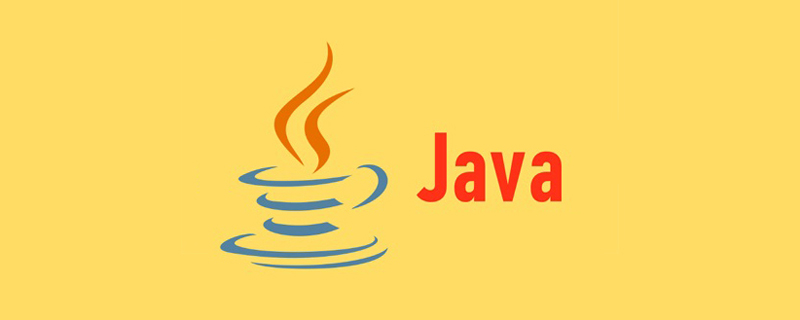
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
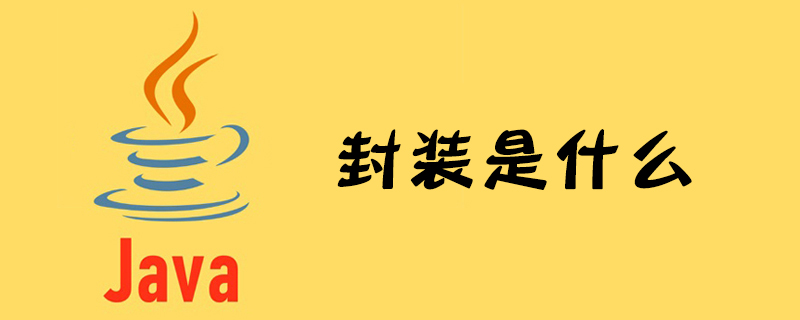
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
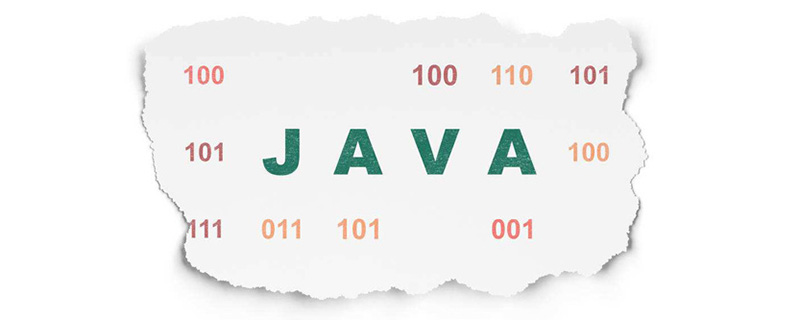
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于设计模式的相关问题,主要将装饰器模式的相关内容,指在不改变现有对象结构的情况下,动态地给该对象增加一些职责的模式,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
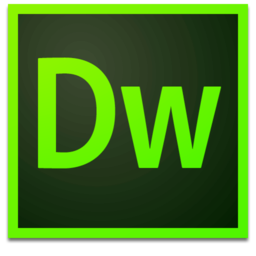
Dreamweaver Mac version
Visual web development tools
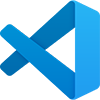
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
