Golang is an advanced programming language that is widely used in modern web applications, cloud computing, container technology and microservices. In the field of data processing, it is often necessary to convert data into big and small ends. This article will introduce you to Golang's big and small endian conversion methods.
- What is big and small endian
In a computer, each data type has its representation in memory, the most important of which is byte order, usually Called big or small endian. "Big endian" means that the highest byte is stored first, and "little endian" means that the lowest byte is stored first. For example, if a 16-bit number is 0x1234, it occupies two bytes of memory in big-endian mode. The first byte is 0x12 and the second byte is 0x34. In little-endian mode, The first byte is 0x34 and the second byte is 0x12.
- Big-endian conversion in Golang
In Golang, you can perform big-endian conversion operations through the Binary package in the standard library. The Binary package provides functions for converting between basic types and byte arrays, including functions for converting big-endian and little-endian byte order.
Among them, this article focuses on the following functions:
- func LittleEndian.Uint16(b []byte) uint16
Convert the little-endian sequence b Convert to an integer of type uint16.
- func LittleEndian.Uint32(b []byte) uint32
Convert the little-endian sequence b to an integer of type uint32.
- func LittleEndian.Uint64(b []byte) uint64
Convert the little-endian sequence b to an integer of uint64 type.
- func LittleEndian.PutUint16(b []byte, v uint16)
Write the uint16 type integer v into the little-endian sequence b.
- func LittleEndian.PutUint32(b []byte, v uint32)
Write the uint32 type integer v into the little-endian sequence b.
- func LittleEndian.PutUint64(b []byte, v uint64)
Write the uint64 type integer v into the little-endian sequence b.
For the conversion of big-endian sequences, the Binary package also provides corresponding functions. Just replace the function name prefix from BigEndian to LittleEndian.
- Little endian conversion example
Below, we give an example of little endian conversion, taking uint16 as an example.
package main
import (
"encoding/binary" "fmt"
)
func main() {
src := []byte{0x34, 0x12} val := binary.LittleEndian.Uint16(src) fmt.Println(val) dest := make([]byte, 2) binary.LittleEndian.PutUint16(dest, val) fmt.Printf("%x", dest)
}
at In this example, we define a byte array src with a length of 2, which contains a uint16 type value. We use binary.LittleEndian.Uint16(src) to convert it into a little-endian sequence and print it out. Then we write the value to the new byte array dest through binary.LittleEndian.PutUint16(dest, val), and use the Printf function to output its hexadecimal representation. Run the program and the output result is as follows:
4660
1234
It can be seen that the uint16 type value 0x1234 is represented as 0x3412 in the little-endian sequence, and is converted back It can be restored to the original value later.
- Summary
By using the functions of the Binary package, we can easily perform big-endian conversion operations in Golang. In practical applications, endian conversion is a very common data conversion method and is widely used in computer networks, storage systems, image processing and other fields. Therefore, mastering Golang's big and small endian conversion operations will be of great help to developers in data processing.
The above is the detailed content of golang big endian conversion. For more information, please follow other related articles on the PHP Chinese website!
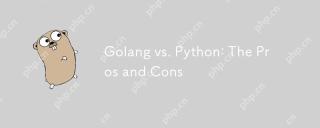
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
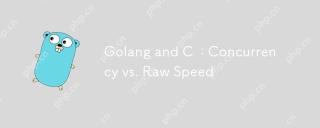
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
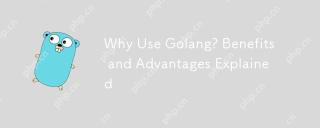
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
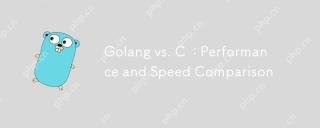
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
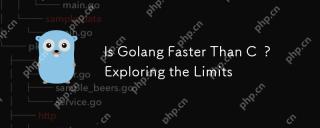
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
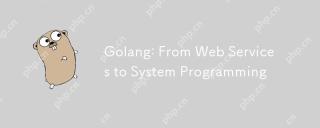
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
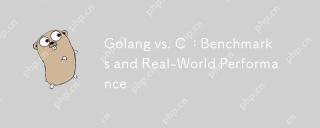
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
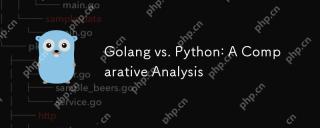
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.