In golang, the error type is a very common data type. During our development process, we often encounter situations where we need to convert the error type into a string type. This article will introduce several common golang error conversion methods for your reference.
Method 1: Use the Error() function
In golang, the Error() function is a built-in method of the error type, which returns a string type error message. Therefore, we can use the Error() function to convert the error type into a string type. The specific implementation is as follows:
func ErrorToString(err error) string { return err.Error() }
This method is very simple and direct. You only need to call the Error() function to convert the error into a string. However, sometimes the error information returned by calling the Error() function may not be detailed enough or vague, so we need to choose other conversion methods.
Method 2: Use fmt.Sprintf()
The fmt.Sprintf() function is a function that formats strings. We can use it to convert the error type into the string type. The specific implementation is as follows:
func ErrorToString(err error) string { return fmt.Sprintf("%v", err) }
This conversion method is very simple to use. You only need to pass the error type as a parameter to the fmt.Sprintf() function. However, the error message it generates may not be as detailed as that generated by the Error() function.
Method 3: Use errors.New()
The errors.New() function is a built-in function in golang. It returns a value of type error. We can use this function to create a new error message and convert it to string type. The specific implementation is as follows:
func ErrorToString(err error) string { return errors.New(err.Error()).Error() }
This conversion method first converts the error type into the string type, then uses the errors.New() function to create a new error type value, and finally calls the Error() function to convert it Convert to string type. Although this method is more cumbersome, it produces more detailed error messages.
Method 4: Using github.com/pkg/errors
github.com/pkg/errors is an open source golang library that provides some extended error handling functions. Among them, the most useful one is the Errorf() function, which is similar to the fmt.Sprintf() function and can format error information and return a new error type value. The specific implementation is as follows:
import ( "github.com/pkg/errors" ) func ErrorToString(err error) string { return errors.Errorf("%+v", err).Error() }
This conversion method is very convenient to use, and the error message generated is very detailed. Of course, using this library will increase your project's dependencies, so careful consideration is needed before using it.
Summary
In golang, converting error type to string type is a very common operation. This article introduces several commonly used conversion methods, including using the Error() function, fmt.Sprintf() function, errors.New() function, and the Errorf() function in the github.com/pkg/errors library. Which method to choose depends on the developer's specific needs and the circumstances of the project.
The above is the detailed content of golang error to string. For more information, please follow other related articles on the PHP Chinese website!
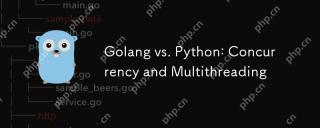
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
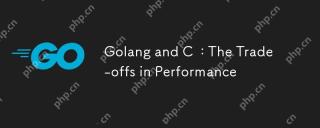
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
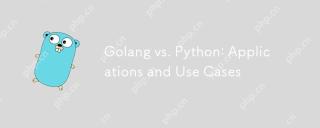
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
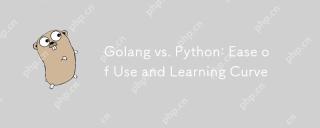
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
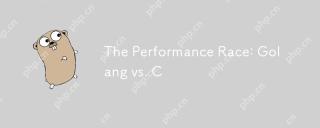
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
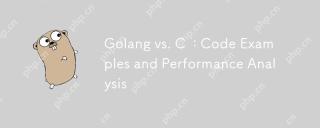
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
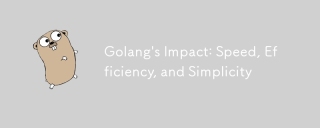
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
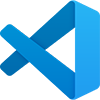
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
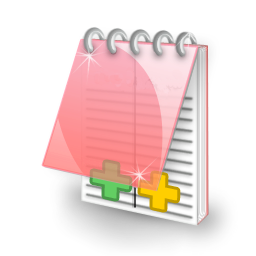
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function