In Golang, modifying the pointer of the DLL file is an important task, because the pointer in the DLL file is the key to connecting multiple modules. However, due to the special nature of the Golang language, it is relatively difficult to modify the DLL pointer.
Below, we will introduce one by one how to modify the DLL pointer in Golang.
1. Understand the Golang memory model
In Golang, the memory model is based on pointers. Therefore, we need to understand the use of pointers and their characteristics. In Golang, a pointer is a value that points to a memory address and can be used to read or modify the memory value at this address. Pointers can also be used to point to the address of a function, variable, or other data type.
In Golang, the pointer is declared as follows:
var ptr *int //Declare a pointer to int type
ptr = &num //Change the address of num Assign a value to the pointer ptr
. Among them, the & operator returns a pointer to a variable, and the * operator is used to dereference the pointer, that is, to read the value at the memory address pointed by the pointer.
2. Import DLL files
To import DLL files in Golang, you need to use the syscall package. Before importing the DLL file, we need to define some function pointers. These function pointers are declared in the DLL file, and we need to access them through pointers.
For example, if we want to import a DLL file named "mylib.dll", and it contains a function named "SetPointer", we need to use the following code:
import (
"syscall" "unsafe"
)
type SetPointerType func(ptr *int) int
var (
myLib *syscall.LazyDLL setPointerFunc SetPointerType
)
func init() {
myLib = syscall.NewLazyDLL("mylib.dll") setPointerFunc = myLib.NewProc("SetPointer").Addr()
}
In this code segment, we first define a SetPointerType type, which is a function type that receives a pointer to int type as a parameter and returns a value of int type.
After that, we use the LazyDLL function to import the mylib.dll file, and use the NewProc function to obtain the address of the SetPointer function.
3. Modify the DLL pointer
In the previous code segment, we have obtained the address of the SetPointer function, and now we can use it to modify the value of the pointer.
For example, for the following code:
num := 1 //This is an int type variable
ptr := &num //Assign the address of num to ptr Pointer
ret := setPointerFunc(ptr) //Pass the pointer to the setPointer function
fmt.Println(*ptr) //Output the value of the pointer
Among them, setPointerFunc The function points to the SetPointer function in the DLL file. We pass the pointer ptr as a parameter to this function. The function will modify the value at the corresponding address to 100 and return a value. Finally, we read the value on pointer ptr and print it.
It should be noted that in Golang, the copying of pointers is shallow copying, that is, only the value of the pointer is copied, but the value at the memory address pointed by the pointer is not copied. Therefore, you need to be careful when modifying pointers to avoid unexpected effects.
4. Summary
Modifying DLL pointers in Golang is a relatively basic task. You need to understand the Golang memory model and how to use pointers, and how to import DLL files. Modification of DLL pointers requires caution to avoid unexpected effects. Correctly modifying the DLL pointer can enhance the flexibility and scalability of the program and improve code reusability.
The above is the detailed content of golang modifies dll pointer. For more information, please follow other related articles on the PHP Chinese website!
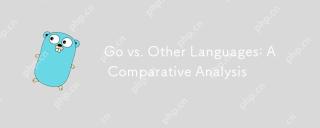
Goisastrongchoiceforprojectsneedingsimplicity,performance,andconcurrency,butitmaylackinadvancedfeaturesandecosystemmaturity.1)Go'ssyntaxissimpleandeasytolearn,leadingtofewerbugsandmoremaintainablecode,thoughitlacksfeatureslikemethodoverloading.2)Itpe
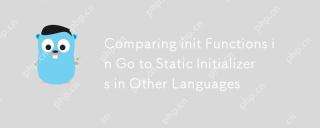
Go'sinitfunctionandJava'sstaticinitializersbothservetosetupenvironmentsbeforethemainfunction,buttheydifferinexecutionandcontrol.Go'sinitissimpleandautomatic,suitableforbasicsetupsbutcanleadtocomplexityifoverused.Java'sstaticinitializersoffermorecontr
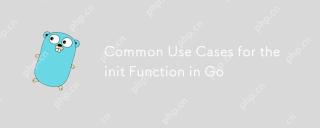
ThecommonusecasesfortheinitfunctioninGoare:1)loadingconfigurationfilesbeforethemainprogramstarts,2)initializingglobalvariables,and3)runningpre-checksorvalidationsbeforetheprogramproceeds.Theinitfunctionisautomaticallycalledbeforethemainfunction,makin
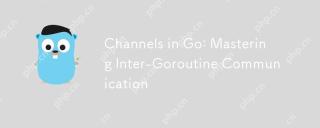
ChannelsarecrucialinGoforenablingsafeandefficientcommunicationbetweengoroutines.Theyfacilitatesynchronizationandmanagegoroutinelifecycle,essentialforconcurrentprogramming.Channelsallowsendingandreceivingvalues,actassignalsforsynchronization,andsuppor
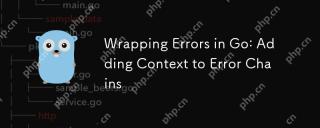
In Go, errors can be wrapped and context can be added via errors.Wrap and errors.Unwrap methods. 1) Using the new feature of the errors package, you can add context information during error propagation. 2) Help locate the problem by wrapping errors through fmt.Errorf and %w. 3) Custom error types can create more semantic errors and enhance the expressive ability of error handling.
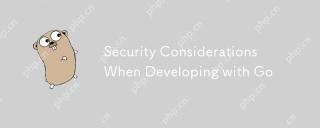
Gooffersrobustfeaturesforsecurecoding,butdevelopersmustimplementsecuritybestpracticeseffectively.1)UseGo'scryptopackageforsecuredatahandling.2)Manageconcurrencywithsynchronizationprimitivestopreventraceconditions.3)SanitizeexternalinputstoavoidSQLinj
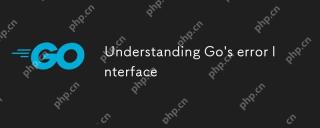
Go's error interface is defined as typeerrorinterface{Error()string}, allowing any type that implements the Error() method to be considered an error. The steps for use are as follows: 1. Basically check and log errors, such as iferr!=nil{log.Printf("Anerroroccurred:%v",err)return}. 2. Create a custom error type to provide more information, such as typeMyErrorstruct{MsgstringDetailstring}. 3. Use error wrappers (since Go1.13) to add context without losing the original error message,
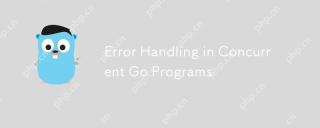
ToeffectivelyhandleerrorsinconcurrentGoprograms,usechannelstocommunicateerrors,implementerrorwatchers,considertimeouts,usebufferedchannels,andprovideclearerrormessages.1)Usechannelstopasserrorsfromgoroutinestothemainfunction.2)Implementanerrorwatcher


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
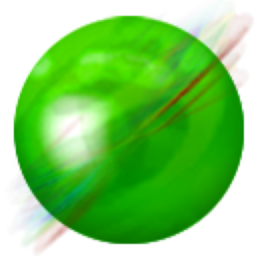
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Chinese version
Chinese version, very easy to use
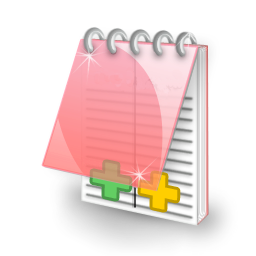
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
